python的异步接收的回调函数
时间: 2024-01-01 19:54:21 浏览: 24
在 Python 中,异步接收的回调函数可以使用 `asyncio` 模块中的 `Protocol` 类来实现。`Protocol` 是一个抽象基类,用于定义异步网络协议。具体的实现中,需要定义一个继承自 `Protocol` 的类,并实现其中的方法。
下面是一个简单的例子,演示如何使用 `Protocol` 类实现异步接收的回调函数:
```python
import asyncio
class MyProtocol(asyncio.Protocol):
def __init__(self, on_data_received):
self.on_data_received = on_data_received
def connection_made(self, transport):
print('Connection made')
self.transport = transport
def data_received(self, data):
print('Data received:', data)
self.on_data_received(data)
def connection_lost(self, exc):
print('Connection lost')
asyncio.get_event_loop().stop()
async def main():
def on_data_received(data):
print('Data received in callback:', data)
loop = asyncio.get_event_loop()
_, protocol = await loop.create_connection(lambda: MyProtocol(on_data_received), 'localhost', 1234)
await asyncio.sleep(1)
protocol.transport.write(b'Hello, world!')
await asyncio.sleep(1)
if __name__ == '__main__':
asyncio.run(main())
```
在上面的例子中,`MyProtocol` 类继承自 `asyncio.Protocol`,并实现了 `connection_made`、`data_received` 和 `connection_lost` 三个方法。其中,`connection_made` 在连接建立时被调用,`data_received` 在接收到数据时被调用,`connection_lost` 在连接断开时被调用。
在 `main` 函数中,我们使用 `create_connection` 方法创建了一个与服务器的连接,并将 `MyProtocol` 类的实例作为回调函数传入。在回调函数中,我们定义了一个 `on_data_received` 函数,用于处理接收到的数据。当数据接收完成后,调用 `on_data_received` 函数进行处理。
最后,在 `main` 函数中,我们使用 `asyncio.sleep` 方法暂停了一段时间,然后向服务器发送了一条消息。
相关推荐
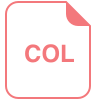
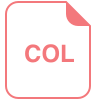
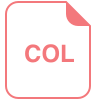
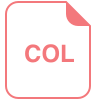
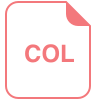









