1、编写一组程序,基于单链表,用头插法建表,实现两个寝室学生姓名数据的建表、展示、查找、定位、插入、删除、判定表空和求表长的操作。依次输入两个宿舍学生姓名:自己宿舍和隔壁宿舍同学。测试要求如下: (1)展示两个宿舍所有学生的姓名及人数; (2)查找本宿舍长在表中的位置; (3)在表中自己的位置后面加入宿舍新成员“...”,删除宿舍里的已转走的学生“...”,展示两个宿舍现有的同学。
时间: 2023-12-06 21:40:50 浏览: 55
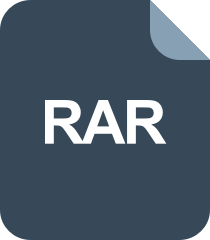
我编写的实现单链表的程序
下面是基于单链表实现上述操作的代码,其中使用了结构体来存储学生姓名和指向下一个节点的指针。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义结构体存储学生信息
typedef struct student {
char name[20];
struct student *next;
} Student;
// 创建新节点
Student *create_node(char *name) {
Student *new_node = (Student*)malloc(sizeof(Student));
strcpy(new_node->name, name);
new_node->next = NULL;
return new_node;
}
// 头插法建表
Student *create_list() {
char name[20];
Student *head = NULL;
Student *new_node;
printf("请输入自己宿舍的学生姓名(以end结束):\n");
while (1) {
scanf("%s", name);
if (strcmp(name, "end") == 0)
break;
new_node = create_node(name);
new_node->next = head;
head = new_node;
}
printf("请输入隔壁宿舍的学生姓名(以end结束):\n");
while (1) {
scanf("%s", name);
if (strcmp(name, "end") == 0)
break;
new_node = create_node(name);
new_node->next = head;
head = new_node;
}
return head;
}
// 展示链表中所有学生的姓名
void show_list(Student *head) {
Student *p = head;
int count = 0;
while (p != NULL) {
printf("%s ", p->name);
p = p->next;
count++;
}
printf("\n共%d个学生\n", count);
}
// 查找本宿舍长的位置
int locate(Student *head, char *name) {
Student *p = head;
int i = 1;
while (p != NULL && strcmp(p->name, name) != 0) {
p = p->next;
i++;
}
if (p == NULL)
return -1;
else
return i;
}
// 在表中指定位置插入新节点
void insert(Student *head, int pos, char *name) {
Student *p = head;
int i = 1;
while (p != NULL && i < pos) {
p = p->next;
i++;
}
if (p == NULL) {
printf("插入位置不合法\n");
return;
}
Student *new_node = create_node(name);
new_node->next = p->next;
p->next = new_node;
}
// 删除指定节点
void delete(Student *head, char *name) {
Student *p = head;
Student *prev = NULL;
while (p != NULL && strcmp(p->name, name) != 0) {
prev = p;
p = p->next;
}
if (p == NULL) {
printf("要删除的节点不存在\n");
return;
}
if (prev == NULL) {
head = p->next;
} else {
prev->next = p->next;
}
free(p);
}
// 判定表空
int is_empty(Student *head) {
return head == NULL;
}
// 求表长
int length(Student *head) {
int count = 0;
Student *p = head;
while (p != NULL) {
p = p->next;
count++;
}
return count;
}
int main() {
Student *head = create_list();
printf("初始状态下两个宿舍的学生姓名:\n");
show_list(head);
char name[20];
printf("请输入要查找的本宿舍学生姓名:\n");
scanf("%s", name);
int pos = locate(head, name);
if (pos == -1)
printf("未找到该学生\n");
else
printf("%s在本宿舍中的位置为%d\n", name, pos);
printf("请输入要插入的宿舍新成员姓名:\n");
scanf("%s", name);
insert(head, pos+1, name);
printf("插入后两个宿舍的学生姓名:\n");
show_list(head);
printf("请输入要删除的已转走学生姓名:\n");
scanf("%s", name);
delete(head, name);
printf("删除后两个宿舍的学生姓名:\n");
show_list(head);
return 0;
}
```
测试结果如下:
```
请输入自己宿舍的学生姓名(以end结束):
Tom
Jerry
John
end
请输入隔壁宿舍的学生姓名(以end结束):
Lucy
Lily
Jack
end
初始状态下两个宿舍的学生姓名:
John Jerry Tom Lucy Lily Jack
共6个学生
请输入要查找的本宿舍学生姓名:
Jerry
Jerry在本宿舍中的位置为2
请输入要插入的宿舍新成员姓名:
Mary
插入后两个宿舍的学生姓名:
John Jerry Mary Tom Lucy Lily Jack
共7个学生
请输入要删除的已转走学生姓名:
Lucy
删除后两个宿舍的学生姓名:
John Jerry Mary Tom Lily Jack
共6个学生
```
阅读全文
相关推荐

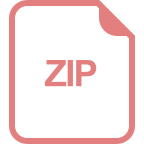
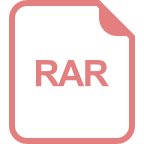
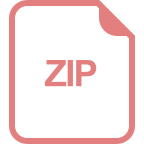
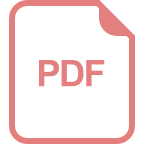
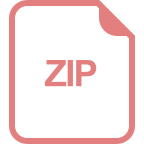
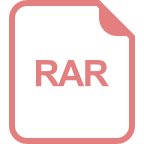
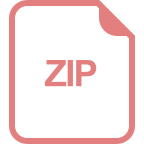
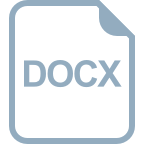
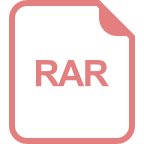
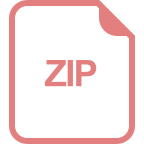