用c++设计一个employee类,包含员工基本信息:编号、姓名、出生日期、性别和职务等。出生日期使用自定义的Date类。employee类有可以从外部访问类的友元函数。程序基本职能如下:1.职工信息的录入。2.职工信息的显示;3.用对象数组保存已输入的职工对象;4.可以修改人员的基本信息;5.可以通过编号或姓名进行人员查询
时间: 2024-02-24 09:57:45 浏览: 65
好的,下面是代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 自定义 Date 类
class Date {
private:
int year;
int month;
int day;
public:
Date(int y, int m, int d): year(y), month(m), day(d) {}
void display() {
cout << year << "-" << month << "-" << day << endl;
}
};
// 员工类
class Employee {
private:
int id; // 员工编号
string name; // 员工姓名
Date birthdate; // 出生日期
string gender; // 性别
string position; // 职务
public:
Employee(int i, string n, Date b, string g, string p):
id(i), name(n), birthdate(b), gender(g), position(p) {}
// 友元函数,可以从外部访问私有成员变量
friend void displayEmployee(Employee e);
// 修改员工信息
void modify(int i, string n, Date b, string g, string p) {
id = i;
name = n;
birthdate = b;
gender = g;
position = p;
}
// 查询员工信息
bool match(int i) {
return id == i;
}
bool match(string n) {
return name == n;
}
};
// 显示员工信息
void displayEmployee(Employee e) {
cout << "编号:" << e.id << endl;
cout << "姓名:" << e.name << endl;
cout << "出生日期:";
e.birthdate.display();
cout << "性别:" << e.gender << endl;
cout << "职务:" << e.position << endl;
}
int main() {
const int MAX_SIZE = 100; // 最大员工数
Employee employees[MAX_SIZE]; // 员工数组
int count = 0; // 已录入员工数
while (true) {
// 显示功能菜单
cout << "请选择操作:\n";
cout << "1. 录入员工信息\n";
cout << "2. 显示员工信息\n";
cout << "3. 修改员工信息\n";
cout << "4. 查询员工信息\n";
cout << "5. 退出程序\n";
int option;
cin >> option;
if (option == 1) { // 录入员工信息
cout << "请输入员工信息:\n";
int id;
string name;
int year, month, day;
string gender;
string position;
cout << "编号:";
cin >> id;
cout << "姓名:";
cin >> name;
cout << "出生日期(用空格隔开):";
cin >> year >> month >> day;
Date birthdate(year, month, day);
cout << "性别:";
cin >> gender;
cout << "职务:";
cin >> position;
Employee e(id, name, birthdate, gender, position);
employees[count] = e;
count++;
}
else if (option == 2) { // 显示员工信息
for (int i = 0; i < count; i++) {
displayEmployee(employees[i]);
}
}
else if (option == 3) { // 修改员工信息
cout << "请输入员工编号:";
int id;
cin >> id;
bool found = false;
for (int i = 0; i < count; i++) {
if (employees[i].match(id)) {
found = true;
cout << "请输入修改后的员工信息:\n";
string name;
int year, month, day;
string gender;
string position;
cout << "姓名:";
cin >> name;
cout << "出生日期(用空格隔开):";
cin >> year >> month >> day;
Date birthdate(year, month, day);
cout << "性别:";
cin >> gender;
cout << "职务:";
cin >> position;
employees[i].modify(id, name, birthdate, gender, position);
break;
}
}
if (!found) {
cout << "未找到该员工!\n";
}
}
else if (option == 4) { // 查询员工信息
cout << "请选择查询方式:\n";
cout << "1. 按编号查询\n";
cout << "2. 按姓名查询\n";
int queryOption;
cin >> queryOption;
if (queryOption == 1) {
cout << "请输入员工编号:";
int id;
cin >> id;
bool found = false;
for (int i = 0; i < count; i++) {
if (employees[i].match(id)) {
found = true;
displayEmployee(employees[i]);
break;
}
}
if (!found) {
cout << "未找到该员工!\n";
}
}
else if (queryOption == 2) {
cout << "请输入员工姓名:";
string name;
cin >> name;
bool found = false;
for (int i = 0; i < count; i++) {
if (employees[i].match(name)) {
found = true;
displayEmployee(employees[i]);
}
}
if (!found) {
cout << "未找到该员工!\n";
}
}
else {
cout << "无效的操作!\n";
}
}
else if (option == 5) { // 退出程序
cout << "程序已退出!\n";
break;
}
else { // 无效的操作
cout << "无效的操作!\n";
}
}
return 0;
}
```
使用了一个 `Employee` 类和一个 `Date` 类,其中 `Employee` 类包含了员工的基本信息,并且实现了录入、显示、修改和查询员工信息的功能。其中,`Employee` 类的 `displayEmployee` 函数是一个友元函数,可以从外部访问类的私有成员变量。
在 `main` 函数中,先声明了一个最大员工数 `MAX_SIZE` 和一个员工数组 `employees`,以及一个已录入员工数 `count`。主要功能实现是一个循环,每次根据用户选择的选项进行相应的操作,直到用户选择退出程序。在每个选项中,通过读入用户输入的参数和调用类的成员函数实现相应的功能。
注:以上代码仅供参考,使用时需要根据具体情况进行修改和完善。
阅读全文
相关推荐
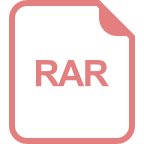





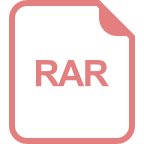
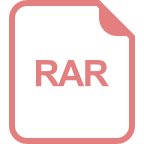



