from PIL import Image import numpy as np import matplotlib.pyplot as plt img = Image.open("work/Lena_RGB.jpg") plt.imshow(img) plt.show() plt.close() img_gray = img.convert('L') # 转换为灰度图像 img_arr = np.array(img_gray) h, w = img_arr.shape gray_levels = np.arange(256) freq = np.zeros(256) for i in range(h): for j in range(w): freq[img_arr[i, j]] += 1 prob = freq / (h * w) plt.bar(gray_levels, prob) fig, axs = plt.subplots(2, 2, figsize=(12, 8)) axs[0, 0].imshow(img, cmap='gray') axs[0, 0].set_title('Original Image') axs[0, 1].bar(gray_levels, freq) axs[0, 1].set_title('Gray Level Frequency') axs[1, 0].bar(gray_levels, self_info) axs[1, 0].set_title('Self Information') axs[1, 1].text(0.5, 0.5, f'Entropy: {entropy:.2f}', fontsize=20, ha='center') axs[1, 1].axis('off') plt.show() 修改代码
时间: 2023-12-24 16:04:53 浏览: 31
以下是修改后的代码:
```python
from PIL import Image
import numpy as np
import matplotlib.pyplot as plt
img = Image.open("work/Lena_RGB.jpg")
plt.imshow(img)
plt.show()
plt.close()
img_gray = img.convert('L') # 转换为灰度图像
img_arr = np.array(img_gray)
h, w = img_arr.shape
gray_levels = np.arange(256)
freq = np.zeros(256)
for i in range(h):
for j in range(w):
freq[img_arr[i, j]] += 1
prob = freq / (h * w)
self_info = -np.log2(prob)
entropy = np.sum(prob * self_info)
fig, axs = plt.subplots(2, 2, figsize=(12, 8))
axs[0, 0].imshow(img_gray, cmap='gray')
axs[0, 0].set_title('Gray Image')
axs[0, 1].bar(gray_levels, freq)
axs[0, 1].set_title('Gray Level Frequency')
axs[1, 0].bar(gray_levels, self_info)
axs[1, 0].set_title('Self Information')
axs[1, 1].text(0.5, 0.5, f'Entropy: {entropy:.2f}', fontsize=20, ha='center')
axs[1, 1].axis('off')
plt.show()
```
我在代码中添加了计算图像熵的部分,并且修改了绘图部分,使其显示灰度图像、灰度级频率直方图、自信息直方图和图像熵。
相关推荐
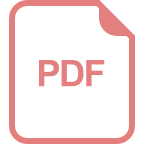
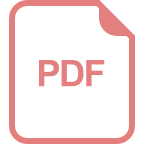














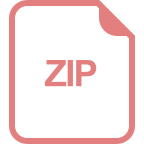
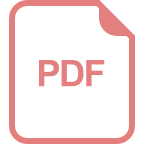