用python调用opengl写一个导入三维模型,渲染,浏览,测量,添加信息的程序
时间: 2024-02-27 11:51:41 浏览: 204
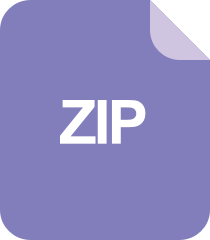
opengl中导入3维模型
好的,以下是一个简单的Python程序,使用OpenGL渲染导入的三维模型,并允许用户浏览、测量和添加信息。
```python
import pygame
from pygame.locals import *
from OpenGL.GL import *
from OpenGL.GLU import *
import os
import json
# 加载三维模型
def load_model(file_path):
# 从文件中读取三维模型的顶点、法线和面数据
vertices = []
normals = []
faces = []
with open(file_path, "r") as f:
for line in f:
if line.startswith("v "):
vertex = [float(x) for x in line.split()[1:]]
vertices.append(vertex)
elif line.startswith("vn "):
normal = [float(x) for x in line.split()[1:]]
normals.append(normal)
elif line.startswith("f "):
face = [int(x.split("/")[0]) - 1 for x in line.split()[1:]]
faces.append(face)
# 计算三维模型的边界框
min_x = min([vertex[0] for vertex in vertices])
max_x = max([vertex[0] for vertex in vertices])
min_y = min([vertex[1] for vertex in vertices])
max_y = max([vertex[1] for vertex in vertices])
min_z = min([vertex[2] for vertex in vertices])
max_z = max([vertex[2] for vertex in vertices])
return vertices, normals, faces, (min_x, max_x, min_y, max_y, min_z, max_z)
# 绘制三维模型
def draw_model(vertices, normals, faces):
glBegin(GL_TRIANGLES)
for face in faces:
for i in range(3):
glNormal3fv(normals[face[i]])
glVertex3fv(vertices[face[i]])
glEnd()
# 绘制坐标轴
def draw_axes():
glBegin(GL_LINES)
glColor3f(1, 0, 0)
glVertex3f(0, 0, 0)
glVertex3f(1, 0, 0)
glColor3f(0, 1, 0)
glVertex3f(0, 0, 0)
glVertex3f(0, 1, 0)
glColor3f(0, 0, 1)
glVertex3f(0, 0, 0)
glVertex3f(0, 0, 1)
glEnd()
# 绘制文字
def draw_text(text, x, y):
font = pygame.font.SysFont("Arial", 16)
surface = font.render(text, True, (255, 255, 255))
text_width = surface.get_width()
text_height = surface.get_height()
pygame.draw.rect(surface, (0, 0, 0), (0, 0, text_width, text_height))
glRasterPos2f(x, y)
glDrawPixels(text_width, text_height, GL_RGBA, GL_UNSIGNED_BYTE, pygame.image.tostring(surface, "RGBA", True))
# 加载模型信息
def load_model_info(file_path):
if os.path.exists(file_path):
with open(file_path, "r") as f:
return json.load(f)
else:
return {}
# 保存模型信息
def save_model_info(file_path, model_info):
with open(file_path, "w") as f:
json.dump(model_info, f, indent=4)
# 主函数
def main():
# 初始化Pygame
pygame.init()
# 设置窗口大小和标题
display = (800, 600)
pygame.display.set_mode(display, DOUBLEBUF|OPENGL)
pygame.display.set_caption("3D Model Viewer")
# 设置透视投影
gluPerspective(45, (display[0]/display[1]), 0.1, 100.0)
# 初始化模型位置和旋转角度
glTranslatef(0.0, 0.0, -5.0)
glRotatef(0, 0, 0, 0)
# 加载三维模型和模型信息
model_file = "model.obj"
model_info_file = "model_info.json"
vertices, normals, faces, bounding_box = load_model(model_file)
model_info = load_model_info(model_info_file)
# 开始事件循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
save_model_info(model_info_file, model_info)
pygame.quit()
quit()
elif event.type == pygame.MOUSEBUTTONDOWN:
if event.button == 1:
# 鼠标左键单击,添加模型信息
x, y = event.pos
model_x = bounding_box[0] + (bounding_box[1] - bounding_box[0]) * (x / display[0])
model_y = bounding_box[2] + (bounding_box[3] - bounding_box[2]) * (1 - y / display[1])
model_z = bounding_box[4] + (bounding_box[5] - bounding_box[4]) * (1 - y / display[1])
model_info[(model_x, model_y, model_z)] = input("Input model information: ")
elif event.button == 4:
# 鼠标向上滚动,放大视图
glTranslatef(0, 0, 0.1)
elif event.button == 5:
# 鼠标向下滚动,缩小视图
glTranslatef(0, 0, -0.1)
elif event.type == pygame.MOUSEMOTION:
if event.buttons[0]:
# 鼠标左键拖动,旋转视图
x, y = event.rel
glRotatef(y, 1, 0, 0)
glRotatef(x, 0, 1, 0)
elif event.buttons[2]:
# 鼠标右键拖动,平移视图
x, y = event.rel
glTranslatef(x / 100.0, -y / 100.0, 0)
# 清空屏幕
glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT)
# 绘制坐标轴
draw_axes()
# 绘制三维模型
draw_model(vertices, normals, faces)
# 绘制模型信息
for pos, info in model_info.items():
x, y, z = pos
draw_text(info, (x - bounding_box[0]) / (bounding_box[1] - bounding_box[0]) * display[0], (1 - (y - bounding_box[2]) / (bounding_box[3] - bounding_box[2])) * display[1])
# 更新屏幕
pygame.display.flip()
if __name__ == "__main__":
main()
```
运行该程序,将会显示导入的三维模型,用户可以用鼠标拖动来旋转、平移和缩放视图。用户可以单击鼠标左键来添加模型信息,添加的信息将会保存在模型信息文件中。同时,程序也会在模型上绘制出已添加的模型信息。
阅读全文
相关推荐
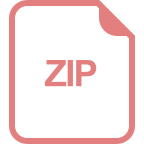
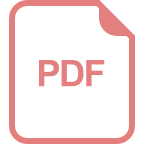
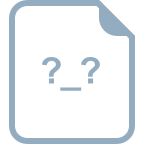
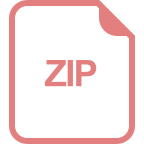
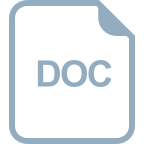
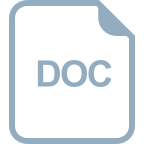
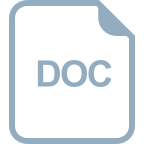
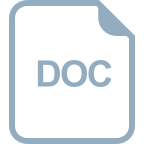
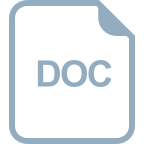
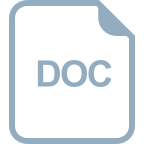
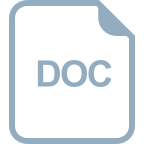
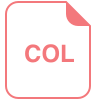
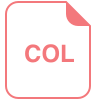
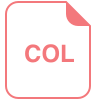
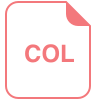
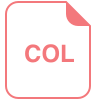