java从前端传实体类给后端如果字段为空就设置为0进行更新代码如何写
时间: 2024-04-09 13:34:12 浏览: 135
你可以在后端的更新代码中进行判断,如果前端传来的实体类字段为空,就将其设置为0再进行更新操作。以下是一个示例代码:
```java
@PostMapping("/update")
public ResponseEntity<String> updateEntity(@RequestBody YourEntity entity) {
if (entity.getField1() == null) {
entity.setField1(0);
}
if (entity.getField2() == null) {
entity.setField2(0);
}
// 其他字段的判断和设置...
// 进行更新操作
// ...
return ResponseEntity.ok("Update successful");
}
```
在这个示例中,我们假设实体类名为 `YourEntity`,其中有两个字段 `field1` 和 `field2`。在接收到前端传来的实体类后,我们先判断每个字段是否为空,如果为空,就将其设置为0,然后再进行更新操作。
你可以根据实际情况,将上述代码中的 `YourEntity` 替换为你自己的实体类名,并根据实际字段进行判断和设置。
相关问题
在java中,post请求前端传来对象中的time端为“2023-09-23”,后端实体类time字段类型为LocalDateTime,如何接收
在Java中处理POST请求并接收前端传来的LocalDateTime类型的`time`值时,你需要做以下几个步骤:
1. 创建后端实体类(通常是一个Java Bean):
```java
import java.time.LocalDateTime;
public class YourRequestObject {
private LocalDateTime time; // 时间字段
// 构造函数、getter和setter方法
public YourRequestObject(LocalDateTime time) {
this.time = time;
}
public LocalDateTime getTime() {
return time;
}
public void setTime(LocalDateTime time) {
this.time = time;
}
}
```
2. 定义REST控制器(Spring MVC或类似框架)的方法来处理POST请求:
```java
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class YourController {
@PostMapping("/your-endpoint")
public ResponseEntity<?> handlePostRequest(@RequestBody YourRequestObject requestObject) {
// 检查时间是否有效,如果需要
if (requestObject.getTime().isValid()) {
// 进行其他业务处理
// ...
return ResponseEntity.ok("Received and processed");
} else {
return ResponseEntity.badRequest().body("Invalid date format");
}
}
}
```
在这个例子中,`@RequestBody`注解告诉Spring将接收到的JSON数据转换成`YourRequestObject`实例。
3. 接收前端的请求:
前端通常是使用JavaScript库(如axios或fetch)发送POST请求,并将日期格式化为合适的字符串,例如ISO 8601格式 (`yyyy-MM-dd'T'HH:mm:ss.SSSZ`):
```javascript
const formData = new FormData();
formData.append('time', '2023-09-23T00:00:00'); // 假设是这个时间格式
axios.post('/your-endpoint', formData)
.then(response => console.log(response.data))
.catch(error => console.error(error));
```
当前端发送请求时,服务器会自动尝试将其转换为`LocalDateTime`,前提是前端传递的格式正确匹配。
mybatisplus java 根据前端传表名和查询条件 后端获取指定表中数据 请补全所有方法
以下是根据前端传表名和查询条件获取指定表中数据的完整方法:
1. 在实体类中添加表名注解,用于映射数据库表名:
```java
@Table(name = "your_table_name")
public class YourEntity {
// 实体类字段和对应数据库表字段
// ...
}
```
2. 创建 DAO 层接口,继承 MyBatis-Plus 的 `BaseMapper` 接口,并添加对应的方法:
```java
public interface YourDao extends BaseMapper<YourEntity> {
// 根据查询条件查询指定表中数据
List<YourEntity> selectByCondition(@Param("tableName") String tableName, @Param("condition") Map<String, Object> condition);
}
```
3. 在 Service 层中调用 DAO 层的方法:
```java
@Service
public class YourService {
@Autowired
private YourDao yourDao;
public List<YourEntity> selectByCondition(String tableName, Map<String, Object> condition) {
return yourDao.selectByCondition(tableName, condition);
}
}
```
4. 在 Controller 层中接收前端传来的表名和查询条件,并调用 Service 层的方法:
```java
@RestController
@RequestMapping("/your-api")
public class YourController {
@Autowired
private YourService yourService;
@GetMapping("/{tableName}")
public List<YourEntity> selectByCondition(@PathVariable("tableName") String tableName, @RequestParam Map<String, Object> condition) {
return yourService.selectByCondition(tableName, condition);
}
}
```
这样,前端传来表名和查询条件时,Controller 层会将它们传递给 Service 层,Service 层再调用 DAO 层的方法进行查询,最终返回查询结果。
阅读全文
相关推荐
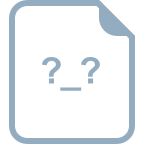
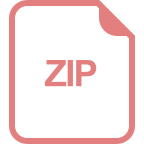
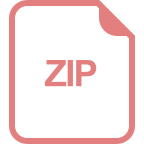
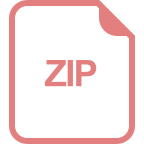
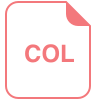









