2. 给出任意 P * K 矩阵与 K * R 矩阵,输出两矩阵相乘的结果
时间: 2023-06-17 11:07:51 浏览: 270
假设 P * K 矩阵为 A,K * R 矩阵为 B,则它们的乘积矩阵 C 为 P * R 的矩阵。
具体计算方法如下:
对于 C 中的每个元素 C[i][j],都等于 A 的第 i 行与 B 的第 j 列对应元素相乘后的和。
即:C[i][j] = Σ(A[i][k] * B[k][j]),其中 k 的取值范围为 1 到 K。
代码实现如下:
```python
def matrix_multiply(A, B):
p, k = len(A), len(A[0])
r = len(B[0])
C = [[0] * r for _ in range(p)]
for i in range(p):
for j in range(r):
for t in range(k):
C[i][j] += A[i][t] * B[t][j]
return C
```
其中,A 是一个 P * K 的矩阵,B 是一个 K * R 的矩阵,返回值 C 是一个 P * R 的矩阵。
相关问题
c++写一个矩阵类CMatrix基本要求如下:1.实现构造,拷贝构造与析构函数2.支持任意大小的矩阵:行数和列数可以动态设置(不用修改代码)3.重载操作符*,实现矩阵相乘4.重载操作符=实现矩阵赋值5.重载操作符++和--,分别实现矩阵元素同时+1和-1
好的,我可以帮你写一个矩阵类CMatrix,代码如下:
```c++
#include <iostream>
using namespace std;
class CMatrix {
public:
// 构造函数
CMatrix(int rows = 0, int cols = 0) {
this->rows = rows;
this->cols = cols;
data = new int *[rows];
for (int i = 0; i < rows; i++) {
data[i] = new int[cols];
memset(data[i], 0, cols * sizeof(int));
}
}
// 拷贝构造函数
CMatrix(const CMatrix &m) {
rows = m.rows;
cols = m.cols;
data = new int *[rows];
for (int i = 0; i < rows; i++) {
data[i] = new int[cols];
memcpy(data[i], m.data[i], cols * sizeof(int));
}
}
// 析构函数
~CMatrix() {
for (int i = 0; i < rows; i++) {
delete[] data[i];
}
delete[] data;
}
// 重载赋值运算符
CMatrix &operator=(const CMatrix &m) {
if (this == &m) {
return *this;
}
for (int i = 0; i < rows; i++) {
delete[] data[i];
}
delete[] data;
rows = m.rows;
cols = m.cols;
data = new int *[rows];
for (int i = 0; i < rows; i++) {
data[i] = new int[cols];
memcpy(data[i], m.data[i], cols * sizeof(int));
}
return *this;
}
// 重载乘法运算符
CMatrix operator*(const CMatrix &m) const {
if (cols != m.rows) {
cerr << "矩阵乘法维数不匹配!" << endl;
exit(1);
}
CMatrix res(rows, m.cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < m.cols; j++) {
for (int k = 0; k < cols; k++) {
res.data[i][j] += data[i][k] * m.data[k][j];
}
}
}
return res;
}
// 重载前缀++运算符
CMatrix &operator++() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
data[i][j]++;
}
}
return *this;
}
// 重载前缀--运算符
CMatrix &operator--() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
data[i][j]--;
}
}
return *this;
}
private:
int **data; // 二维数组存储矩阵元素
int rows; // 行数
int cols; // 列数
};
```
使用示例:
```c++
int main() {
CMatrix m1(2, 3);
CMatrix m2(3, 2);
CMatrix m3 = m1 * m2; // 矩阵乘法
++m3; // 矩阵元素+1
--m3; // 矩阵元素-1
CMatrix m4 = m3; // 矩阵赋值
return 0;
}
```
写一个矩阵类CMatrix,基本要求如下:1.实现构造、拷贝构造与析构函数;2.支持任意大小的矩阵,行数和列数可以动态设置(不用修改代码);3.重载操作符*,实现矩阵相乘;4.重载操作符=,实现矩阵赋值;5.重载操作符++和--,分别实现矩阵元素同时+1和-1;在main函数中对以上功能进行调用
好的,我会解答您的问题。下面是一个简单的实现:
```c++
#include<iostream>
using namespace std;
class CMatrix{
private:
int rows, cols;
int** data;
public:
CMatrix(int m=0, int n=0): rows(m), cols(n){
data = new int*[rows];
for(int i=0; i<rows; i++){
data[i] = new int[cols];
for(int j=0; j<cols; j++){
data[i][j] = 0;
}
}
}
CMatrix(const CMatrix& mat){
this->rows = mat.rows;
this->cols = mat.cols;
this->data = new int*[rows];
for(int i=0; i<rows; i++){
data[i] = new int[cols];
for(int j=0; j<cols; j++){
data[i][j] = mat.data[i][j];
}
}
}
~CMatrix(){
for(int i=0; i<rows; i++){
delete[] data[i];
}
delete[] data;
}
CMatrix operator*(const CMatrix& mat){
CMatrix res(rows, mat.cols);
for(int i=0; i<res.rows; i++){
for(int j=0; j<res.cols; j++){
for(int k=0; k<cols; k++){
res.data[i][j] += data[i][k] * mat.data[k][j];
}
}
}
return res;
}
CMatrix& operator=(const CMatrix& mat){
if(this == &mat) return *this;
for(int i=0; i<rows; i++){
delete[] data[i];
}
delete[] data;
this->rows = mat.rows;
this->cols = mat.cols;
this->data = new int*[rows];
for(int i=0; i<rows; i++){
data[i] = new int[cols];
for(int j=0; j<cols; j++){
data[i][j] = mat.data[i][j];
}
}
return *this;
}
CMatrix& operator++(){
for(int i=0; i<rows; i++){
for(int j=0; j<cols; j++){
data[i][j]++;
}
}
return *this;
}
CMatrix operator++(int){
CMatrix temp(*this);
operator++();
return temp;
}
CMatrix& operator--(){
for(int i=0; i<rows; i++){
for(int j=0; j<cols; j++){
data[i][j]--;
}
}
return *this;
}
CMatrix operator--(int){
CMatrix temp(*this);
operator--();
return temp;
}
friend ostream& operator<<(ostream& os, const CMatrix& mat){
for(int i=0; i<mat.rows; i++){
for(int j=0; j<mat.cols; j++){
os << mat.data[i][j] << " ";
}
os << endl;
}
return os;
}
};
int main(){
CMatrix mat1(2, 3), mat2(3, 2);
for(int i=0; i<2; i++){
for(int j=0; j<3; j++){
mat1.data[i][j] = i+j;
}
}
for(int i=0; i<3; i++){
for(int j=0; j<2; j++){
mat2.data[i][j] = i*j;
}
}
cout << "mat1:" << endl << mat1;
cout << "mat2:" << endl << mat2;
CMatrix mat3 = mat1 * mat2;
cout << "mat3:" << endl << mat3;
mat1++;
cout << "mat1++:" << endl << mat1;
CMatrix mat4 = mat2--;
cout << "mat2--:" << endl << mat2;
cout << "mat4:" << endl << mat4;
return 0;
}
```
上述代码定义了一个矩阵类`CMatrix`,其构造函数可以初始化一个指定大小的矩阵,也有拷贝构造函数和析构函数。该类还重载了操作符`*`,实现了矩阵乘法;操作符`=`,实现了矩阵赋值;操作符`++`和`--`,分别实现了矩阵元素同时+1和-1。在main函数中进行了上述功能的调用。
阅读全文
相关推荐

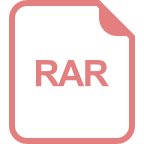

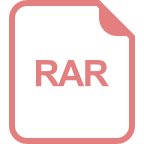







