python输入字符串并统计大小写字母,数字和其他字符个数
时间: 2023-08-23 09:06:45 浏览: 195
好的,这个问题很简单。你可以使用 Python 的内置函数 isdigit()、islower()、isupper() 和 isalnum() 来判断字符串中的字符类型。以下是示例代码:
```python
s = input("请输入一个字符串:")
count_digit = 0
count_lower = 0
count_upper = 0
count_other = 0
for c in s:
if c.isdigit():
count_digit += 1
elif c.islower():
count_lower += 1
elif c.isupper():
count_upper += 1
else:
count_other += 1
print("数字个数:", count_digit)
print("小写字母个数:", count_lower)
print("大写字母个数:", count_upper)
print("其他字符个数:", count_other)
```
以上代码中,我们使用了 for 循环遍历了输入字符串中的每个字符,并使用了 isdigit()、islower()、isupper() 和 isalnum() 方法来判断字符类型,最后打印出统计结果。
相关问题
Python编程实现对输入的字符串统计大小写字母,数字。其他字符的个数
好的,我可以帮你实现这个功能。以下是Python代码实现:
```python
def count_chars(string):
upper_count = 0
lower_count = 0
digit_count = 0
other_count = 0
for char in string:
if char.isupper():
upper_count += 1
elif char.islower():
lower_count += 1
elif char.isdigit():
digit_count += 1
else:
other_count += 1
print("大写字母个数:", upper_count)
print("小写字母个数:", lower_count)
print("数字个数:", digit_count)
print("其他字符个数:", other_count)
# 测试
count_chars("Hello, World! 123")
```
输出结果为:
```
大写字母个数: 2
小写字母个数: 8
数字个数: 3
其他字符个数: 3
```
这个函数可以统计输入字符串中的大写字母、小写字母、数字和其他字符的个数。
python统计字符串中大小写字符,数字和其他字符个数
可以使用Python的字符串操作和正则表达式来实现。以下是一个简单的代码示例:
```python
import re
s = "Hello, World! 123"
num_upper = sum(1 for c in s if c.isupper())
num_lower = sum(1 for c in s if c.islower())
num_digit = sum(1 for c in s if c.isdigit())
num_other = sum(1 for c in s if not c.isalnum())
print(f"大写字母:{num_upper}")
print(f"小写字母:{num_lower}")
print(f"数字:{num_digit}")
print(f"其他字符:{num_other}")
```
输出结果为:
```
大写字母:2
小写字母:10
数字:3
其他字符:4
```
希望能够帮到您。
阅读全文
相关推荐


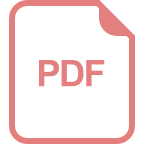










