three.js 怎么在模型上添加下雪 完整代码
时间: 2024-03-28 22:37:27 浏览: 14
下面是一个使用 three.js 在模型上添加下雪的完整代码:
``` html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Snowy Scene</title>
<style>
body {
margin: 0;
overflow: hidden;
}
</style>
</head>
<body>
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/dat-gui/0.7.7/dat.gui.min.js"></script>
<script>
// 创建场景 Scene
const scene = new THREE.Scene();
// 创建相机 Camera
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
camera.position.set(0, 0, 5);
// 创建渲染器 Renderer
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
// 添加雪花材质
const snowflakeTexture = new THREE.TextureLoader().load('https://threejsfundamentals.org/threejs/resources/images/snowflake.png');
const snowflakeMaterial = new THREE.SpriteMaterial({
map: snowflakeTexture,
color: 0xffffff,
fog: true,
});
// 添加雪花粒子群
const snowflakeCount = 500;
const snowflakeRadius = 5;
const snowflakeGeometry = new THREE.Geometry();
const snowflakeParticles = new THREE.Points(snowflakeGeometry, snowflakeMaterial);
for (let i = 0; i < snowflakeCount; i++) {
const x = THREE.Math.randFloatSpread(snowflakeRadius * 2);
const y = THREE.Math.randFloatSpread(snowflakeRadius * 2);
const z = THREE.Math.randFloatSpread(snowflakeRadius * 2);
const snowflake = new THREE.Vector3(x, y, z);
snowflakeGeometry.vertices.push(snowflake);
}
scene.add(snowflakeParticles);
// 加载模型并添加到场景中
const loader = new THREE.GLTFLoader();
loader.load('model.gltf', (gltf) => {
scene.add(gltf.scene);
});
// 控制面板
const controls = {
snowflakeCount: snowflakeCount,
snowflakeRadius: snowflakeRadius,
};
const gui = new dat.GUI();
gui.add(controls, 'snowflakeCount', 0, 1000, 10).onChange(() => {
snowflakeGeometry.verticesNeedUpdate = true;
snowflakeGeometry.vertices.length = 0;
for (let i = 0; i < controls.snowflakeCount; i++) {
const x = THREE.Math.randFloatSpread(controls.snowflakeRadius * 2);
const y = THREE.Math.randFloatSpread(controls.snowflakeRadius * 2);
const z = THREE.Math.randFloatSpread(controls.snowflakeRadius * 2);
const snowflake = new THREE.Vector3(x, y, z);
snowflakeGeometry.vertices.push(snowflake);
}
});
gui.add(controls, 'snowflakeRadius', 1, 10, 1).onChange(() => {
snowflakeGeometry.verticesNeedUpdate = true;
snowflakeGeometry.vertices.length = 0;
for (let i = 0; i < controls.snowflakeCount; i++) {
const x = THREE.Math.randFloatSpread(controls.snowflakeRadius * 2);
const y = THREE.Math.randFloatSpread(controls.snowflakeRadius * 2);
const z = THREE.Math.randFloatSpread(controls.snowflakeRadius * 2);
const snowflake = new THREE.Vector3(x, y, z);
snowflakeGeometry.vertices.push(snowflake);
}
});
// 动画循环
function animate() {
requestAnimationFrame(animate);
snowflakeParticles.rotation.y += 0.001;
renderer.render(scene, camera);
}
animate();
</script>
</body>
</html>
```
在这个代码中,我们首先创建了一个场景 `Scene`、一个相机 `Camera` 和一个渲染器 `Renderer`,然后使用 `THREE.TextureLoader()` 加载了雪花材质,创建了一个雪花粒子群,并将其添加到了场景中。
接着使用 `THREE.GLTFLoader()` 加载了模型文件,并将模型添加到了场景中。
最后,使用 `requestAnimationFrame()` 创建了一个动画循环,在其中旋转了雪花粒子群并渲染了场景。
通过控制面板 `dat.GUI()`,可以动态修改雪花粒子群的数量和半径,从而实现更加丰富的效果。
注意:在使用上述代码时,需要将 `https://threejsfundamentals.org/threejs/resources/images/snowflake.png` 替换为你自己的雪花图片链接,并将 `model.gltf` 替换为你自己的模型文件链接。
相关推荐
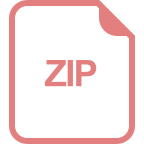














