qt联合halcon编程实现打开图像文件,显示图像文件,图像可以放大,缩小,移动
时间: 2024-05-07 09:15:58 浏览: 175
以下是一个示例代码,使用Qt的QGraphicsView和QGraphicsPixmapItem类来显示图像,同时使用Halcon的zoom_image_factor函数来实现图像的放大、缩小和移动。
```cpp
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include <QFileDialog>
#include <QGraphicsPixmapItem>
#include <QWheelEvent>
#include "HalconCpp.h"
using namespace HalconCpp;
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
// 创建一个 QGraphicsScene 对象,并将其设置为 QGraphicsView 的场景
QGraphicsScene *scene = new QGraphicsScene(this);
ui->graphicsView->setScene(scene);
// 设置 QGraphicsView 的滚轮事件过滤器,以便处理滚轮事件
ui->graphicsView->viewport()->installEventFilter(this);
// 初始化图像缩放比例和图像偏移量
zoom_factor_ = 1.0;
offset_x_ = 0.0;
offset_y_ = 0.0;
}
MainWindow::~MainWindow()
{
delete ui;
}
bool MainWindow::eventFilter(QObject *obj, QEvent *event)
{
if (obj == ui->graphicsView->viewport() && event->type() == QEvent::Wheel) {
// 处理 QGraphicsView 的滚轮事件
QWheelEvent *wheelEvent = static_cast<QWheelEvent*>(event);
if (wheelEvent->modifiers() & Qt::ControlModifier) {
// 如果同时按下了 Ctrl 键,则缩放图像
zoomImage(wheelEvent->delta() > 0 ? 1.1 : 1.0/1.1);
return true;
}
}
return QMainWindow::eventFilter(obj, event);
}
void MainWindow::on_actionOpen_triggered()
{
// 打开图像文件
QString fileName = QFileDialog::getOpenFileName(this, tr("Open Image"), "", tr("Image Files (*.png *.jpg *.bmp)"));
if (fileName.isEmpty())
return;
// 使用 Halcon 的 read_image 函数读取图像文件
HImage image;
image.ReadImage(fileName.toStdString().c_str());
// 将 Halcon 的 HImage 转换为 QImage
const char *format = (image.CountChannels() == 1) ? "Mono" : "Rgb";
QImage qimage((uchar*)image.GetImagePointer1(), image.Width(), image.Height(), image.Width() * image.CountChannels(), QImage::Format(format));
// 显示图像
QGraphicsPixmapItem *item = new QGraphicsPixmapItem(QPixmap::fromImage(qimage));
ui->graphicsView->scene()->addItem(item);
// 将图像居中显示
zoom_factor_ = 1.0;
offset_x_ = (ui->graphicsView->width() - qimage.width()) / 2.0;
offset_y_ = (ui->graphicsView->height() - qimage.height()) / 2.0;
updateTransform();
}
void MainWindow::zoomImage(double factor)
{
// 更新图像缩放比例
zoom_factor_ *= factor;
if (zoom_factor_ < 0.1) zoom_factor_ = 0.1;
if (zoom_factor_ > 10.0) zoom_factor_ = 10.0;
// 更新 QGraphicsView 的变换矩阵
updateTransform();
}
void MainWindow::updateTransform()
{
QTransform transform;
transform.translate(offset_x_, offset_y_);
transform.scale(zoom_factor_, zoom_factor_);
ui->graphicsView->setTransform(transform);
}
void MainWindow::on_actionZoomIn_triggered()
{
zoomImage(1.1);
}
void MainWindow::on_actionZoomOut_triggered()
{
zoomImage(1.0/1.1);
}
void MainWindow::on_actionFit_triggered()
{
// 将图像缩放比例设置为 1.0,并将图像居中显示
zoom_factor_ = 1.0;
offset_x_ = (ui->graphicsView->width() - ui->graphicsView->scene()->width()) / 2.0;
offset_y_ = (ui->graphicsView->height() - ui->graphicsView->scene()->height()) / 2.0;
updateTransform();
}
void MainWindow::on_actionReset_triggered()
{
// 将图像缩放比例和图像偏移量都设置为 0.0
zoom_factor_ = 1.0;
offset_x_ = 0.0;
offset_y_ = 0.0;
updateTransform();
}
void MainWindow::on_actionAbout_triggered()
{
QMessageBox::about(this, tr("About"), tr("Qt and Halcon Image Viewer"));
}
```
在 Qt 的项目文件中,需要将 Halcon 的头文件路径和库文件路径添加到 INCLUDEPATH 和 LIBS 中,例如:
```
INCLUDEPATH += /opt/halcon/include
LIBS += -L/opt/halcon/lib -lhalconcpp -lhalcon
```
注意:以上示例代码仅演示了如何通过 Qt 和 Halcon 实现图像的打开、显示、缩放和移动,实际应用中还需要考虑其他因素,例如图像的旋转、镜像等操作,以及性能优化等问题。
阅读全文
相关推荐
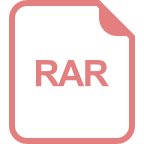
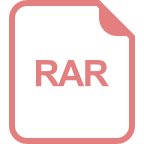
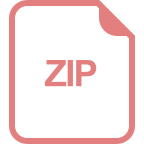
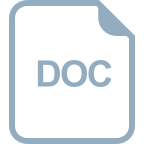

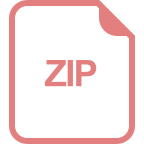
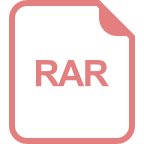
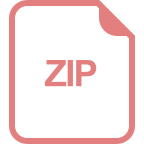
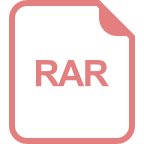
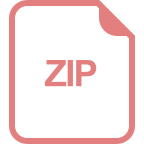
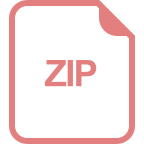
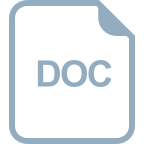
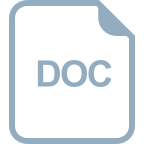
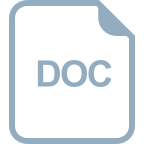
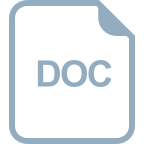