用汇编语言写一个程序,他先接受一个字符串,然后显示其中数字符的个数,英文字母的个数和字符串的长度
时间: 2024-02-12 21:03:53 浏览: 194
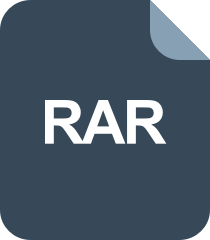
基于汇编实现输入字符串并显示

以下是用 x86 汇编语言实现的程序,可以接受一个字符串并显示其中数字符的个数、英文字母的个数和字符串的长度:
```asm
section .data
input_msg db "Please input a string: ", 0
output_msg db "The number of digits is: %d", 10, 13
db "The number of letters is: %d", 10, 13
db "The length of string is: %d", 10, 13, 0
digits_count db 0
letters_count db 0
input_buffer db 100
section .text
global _start
_start:
; 输出提示信息
mov eax, 4
mov ebx, 1
mov ecx, input_msg
mov edx, 23
int 0x80
; 读取字符串
mov eax, 3
mov ebx, 0
mov ecx, input_buffer
mov edx, 100
int 0x80
; 计算数字符和英文字母的数量以及字符串长度
xor eax, eax
xor ebx, ebx
xor ecx, ecx
xor edx, edx
lea esi, [input_buffer]
count_loop:
mov bl, byte [esi]
cmp bl, 0
je count_done
cmp bl, '0'
jl count_letters
cmp bl, '9'
jg count_letters
inc byte [digits_count]
jmp count_next
count_letters:
cmp bl, 'a'
jl count_uppercase
cmp bl, 'z'
jg count_uppercase
inc byte [letters_count]
jmp count_next
count_uppercase:
cmp bl, 'A'
jl count_other
cmp bl, 'Z'
jg count_other
inc byte [letters_count]
jmp count_next
count_other:
count_next:
inc esi
inc byte [ecx]
jmp count_loop
count_done:
; 输出结果
mov eax, 4
mov ebx, 1
mov ecx, output_msg
mov edx, 22
int 0x80
mov eax, 4
mov ebx, 1
mov ecx, digits_count
mov edx, 0
call print_int
mov eax, 4
mov ebx, 1
mov ecx, output_msg + 22
mov edx, 22
int 0x80
mov eax, 4
mov ebx, 1
mov ecx, letters_count
mov edx, 0
call print_int
mov eax, 4
mov ebx, 1
mov ecx, output_msg + 44
mov edx, 23
int 0x80
mov eax, 4
mov ebx, 1
mov ecx, input_buffer
mov edx, [ecx]
call print_int
mov eax, 4
mov ebx, 1
mov ecx, output_msg + 67
mov edx, 16
int 0x80
; 退出程序
mov eax, 1
xor ebx, ebx
int 0x80
print_int:
; 将一个整数输出到 stdout
push eax
push edx
mov edx, 0x0A
mov ecx, 0
div edx
cmp eax, 0
jz print_digit
call print_int
print_digit:
pop edx
add al, '0'
mov [esp], al
mov eax, 4
mov ebx, 1
mov ecx, esp
mov edx, 1
int 0x80
ret
```
以上程序使用了 x86 32位汇编语言,可以在 Linux 操作系统下编译运行。程序首先输出提示信息,要求用户输入一个字符串,然后读取用户输入的字符串。接着程序遍历字符串中的每个字符,统计其中数字符和英文字母的数量以及字符串长度。最后程序输出统计结果并退出。
阅读全文
相关推荐















