C#读取HEX文件转bin文件
时间: 2023-06-15 19:06:23 浏览: 102
以下是一个简单的示例代码,可以读取一个HEX文件并将其转换为bin文件:
```csharp
using System;
using System.IO;
class HexToBinConverter
{
static void Main()
{
string hexFile = @"C:\example.hex";
string binFile = @"C:\example.bin";
using (StreamReader reader = new StreamReader(hexFile))
using (BinaryWriter writer = new BinaryWriter(File.Open(binFile, FileMode.Create)))
{
while (!reader.EndOfStream)
{
string line = reader.ReadLine();
if (line.StartsWith(":"))
{
byte[] bytes = HexToBytes(line.Substring(1));
writer.Write(bytes);
}
}
}
Console.WriteLine("Conversion complete.");
}
static byte[] HexToBytes(string hex)
{
int len = hex.Length / 2;
byte[] bytes = new byte[len];
for (int i = 0; i < len; i++)
{
bytes[i] = Convert.ToByte(hex.Substring(i * 2, 2), 16);
}
return bytes;
}
}
```
你需要将 `hexFile` 和 `binFile` 变量替换为你自己的文件路径。此代码使用 `StreamReader` 读取HEX文件的每一行,然后使用 `BinaryWriter` 写入转换后的二进制数据。`HexToBytes` 函数将每一行的HEX数据转换为等效的字节数组。
相关推荐
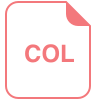
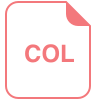












