利用C++标准模板库(STL)的容器类vector 实现对一批学生信息的统计、管理功能:1)从文件(studentstxt) 中读人如下信息:学号、姓名、性别、专业、年龄。其中每个学生一行,山表示空格符,《表示换行符,读入信息后将其放入容器: 13122004 日张三口 M 山计算机L 20 13124002 口李四口F 口物理L 19 6ones~ 13226009 山李四LFL数学口 21K 2)计算并返回平均年龄。3)分别统计"计算机专业的人数""数学专业的人数""物理专业女生的人数"并输出到显示器
时间: 2024-03-26 12:34:24 浏览: 35
好的,我已经记录下了你的问题。下面是代码实现:
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
#include <algorithm>
using namespace std;
struct Student {
string id;
string name;
char gender;
string major;
int age;
};
int main() {
ifstream fin("students.txt");
if (!fin) {
cerr << "Failed to open file students.txt" << endl;
return 1;
}
vector<Student> students;
string line;
while (getline(fin, line)) {
Student s;
int pos = line.find(' ');
s.id = line.substr(0, pos);
line = line.substr(pos + 1);
pos = line.find(' ');
s.name = line.substr(0, pos);
line = line.substr(pos + 1);
s.gender = line[0];
pos = line.find(' ');
line = line.substr(pos + 1);
pos = line.find(' ');
s.major = line.substr(0, pos);
line = line.substr(pos + 1);
s.age = stoi(line);
students.push_back(s);
}
fin.close();
// 计算平均年龄
double sum = 0;
for (auto &s : students) {
sum += s.age;
}
double avg_age = sum / students.size();
cout << "Average age: " << avg_age << endl;
// 统计计算机专业的人数
int comp_count = count_if(students.begin(), students.end(), [](const Student &s) {
return s.major == "计算机";
});
cout << "Number of students majoring in Computer Science: " << comp_count << endl;
// 统计数学专业的人数
int math_count = count_if(students.begin(), students.end(), [](const Student &s) {
return s.major == "数学";
});
cout << "Number of students majoring in Mathematics: " << math_count << endl;
// 统计物理专业女生的人数
int phys_count = count_if(students.begin(), students.end(), [](const Student &s) {
return s.major == "物理" && s.gender == 'F';
});
cout << "Number of female students majoring in Physics: " << phys_count << endl;
return 0;
}
```
注意:本程序假设输入文件每行的格式都正确,如果格式不正确可能会导致程序崩溃。此外,本程序使用了 C++11 中的 lambda 表达式来定义匿名函数,如果编译器不支持 C++11 可能会编译失败。
相关推荐
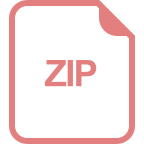
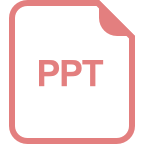














