java hadoop读取从CSV文件,然后提取信息该怎么做
时间: 2024-03-01 18:55:45 浏览: 140
在 Hadoop 中读取和处理 CSV 文件,可以使用 Hadoop 自带的 `TextInputFormat` 以及 `LineRecordReader` 类来实现。下面是一个简单的示例代码,可以读取 CSV 文件并提取信息:
```java
import java.io.IOException;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.*;
import org.apache.hadoop.mapreduce.lib.input.*;
import org.apache.hadoop.mapreduce.lib.output.*;
import org.apache.hadoop.util.*;
public class CsvReader extends Configured implements Tool {
public static class CsvMapper extends Mapper<LongWritable, Text, Text, Text> {
@Override
public void map(LongWritable key, Text value, Context context)
throws IOException, InterruptedException {
String line = value.toString();
String[] fields = line.split(",");
String name = fields[0];
String age = fields[1];
String city = fields[2];
String outputValue = "Age: " + age + ", City: " + city;
context.write(new Text(name), new Text(outputValue));
}
}
public int run(String[] args) throws Exception {
if (args.length != 2) {
System.out.println("Usage: CsvReader <input path> <output path>");
return -1;
}
Configuration conf = getConf();
Job job = Job.getInstance(conf, "CsvReader");
job.setJarByClass(CsvReader.class);
job.setMapperClass(CsvMapper.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
job.setInputFormatClass(TextInputFormat.class);
job.setOutputFormatClass(TextOutputFormat.class);
FileInputFormat.setInputPaths(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
boolean success = job.waitForCompletion(true);
return success ? 0 : 1;
}
public static void main(String[] args) throws Exception {
int exitCode = ToolRunner.run(new CsvReader(), args);
System.exit(exitCode);
}
}
```
在上面的示例代码中,首先定义了一个 `CsvMapper` 类,用来处理 CSV 文件中的每一行数据。在 `map` 方法中,将每一行数据按照逗号分隔符进行拆分,然后提取出需要的数据,最后将姓名作为 key,年龄和城市作为 value 写入输出。
在 `run` 方法中,首先获取输入和输出路径,然后设置作业的 Mapper 类、输出 Key 和 Value 的类型、输入和输出格式等属性。接着将输入和输出路径设置到作业中,并等待作业执行完成。最后,通过 `ToolRunner` 来运行 `CsvReader` 类。
需要注意的是,这里只是一个示例代码,实际情况中,你需要根据自己的需求来修改代码。例如,如果你的 CSV 文件中有标题行,那么需要在 Mapper 类中进行特殊处理,跳过第一行标题。
阅读全文
相关推荐
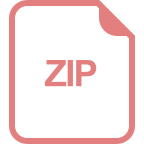
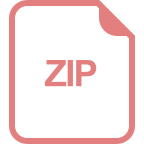
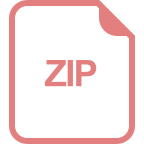
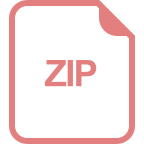
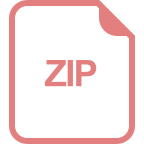
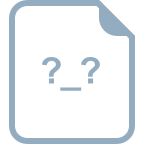
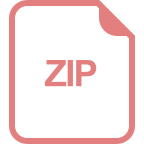
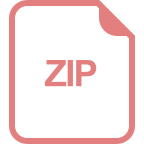