在网页中制作本学期课程表,使用jQuery技术对表格进行如下格式设置: 1、设置课程表背景图,背景图片自选,要求背景图透明化处理; 2、设置表格标题(caption元素)和表格内容的字体、字号和颜色(自定义); 3、表格第一行设置区别于表格主体的背景色和字体; 4、将表格空白单元格设置背景色
时间: 2023-06-13 18:08:16 浏览: 67
可以使用以下代码实现:
```html
<!DOCTYPE html>
<html>
<head>
<title>课程表</title>
<style>
body {
background-image: url(background.png);
background-color: rgba(255, 255, 255, 0.5);
font-family: Arial, sans-serif;
font-size: 14px;
color: #333;
}
table {
margin: 20px auto;
border-collapse: collapse;
border: 1px solid #ccc;
width: 800px;
}
caption {
font-size: 20px;
color: #fff;
background-color: #333;
padding: 10px;
}
th {
background-color: #eee;
font-weight: bold;
padding: 10px;
}
td {
padding: 10px;
background-color: #fff;
}
td:empty {
background-color: #eee;
}
</style>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
$(document).ready(function() {
// 设置表格标题
$("table").prepend("<caption>本学期课程表</caption>");
// 设置第一行背景色和字体
$("table tr:first-child th").css({
"background-color": "#333",
"color": "#fff",
"font-size": "16px",
"font-weight": "bold"
});
// 设置空白单元格背景色
$("table td:empty").css("background-color", "#eee");
});
</script>
</head>
<body>
<table>
<tr>
<th></th>
<th>星期一</th>
<th>星期二</th>
<th>星期三</th>
<th>星期四</th>
<th>星期五</th>
</tr>
<tr>
<th>上午</th>
<td>语文</td>
<td>数学</td>
<td>英语</td>
<td></td>
<td>音乐</td>
</tr>
<tr>
<th>下午</th>
<td>化学</td>
<td>物理</td>
<td></td>
<td>历史</td>
<td>美术</td>
</tr>
<tr>
<th>晚上</th>
<td>篮球</td>
<td></td>
<td>足球</td>
<td>乒乓球</td>
<td></td>
</tr>
</table>
</body>
</html>
```
其中,`background.png`是自选的背景图片,`rgba(255, 255, 255, 0.5)`是背景色的透明度设置,`th`和`td`分别是表格的表头和单元格,`td:empty`表示空白单元格。在jQuery中,使用`$(document).ready()`方法来确保DOM加载完成后再执行JavaScript代码。
相关推荐
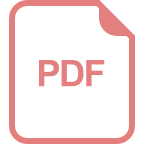
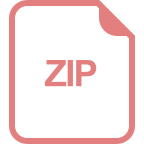
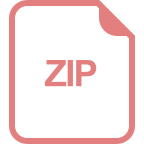








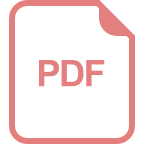
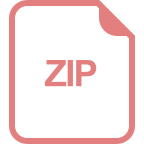