给我一段DES代码
时间: 2023-07-10 16:07:29 浏览: 65
这是一段 Python 中的 DES 加密解密代码示例:
```python
from Crypto.Cipher import DES
import binascii
# 创建 DES 对象,mode 为加密或解密模式
def create_des(key, mode):
iv = b"\x01\x02\x03\x04\x05\x06\x07\x08" # 初始向量,长度必须为 8 字节
if mode == DES.MODE_CBC:
cipher = DES.new(key, mode, iv)
else:
cipher = DES.new(key, mode)
return cipher
# DES 加密
def des_encrypt(text, key):
cipher = create_des(key, DES.MODE_ECB)
# 加密时,明文长度必须为 8 的倍数,不足则填充
text = text + (8 - len(text) % 8) * chr(8 - len(text) % 8)
ciphertext = cipher.encrypt(text.encode())
return binascii.b2a_hex(ciphertext).decode()
# DES 解密
def des_decrypt(ciphertext, key):
cipher = create_des(key, DES.MODE_ECB)
plaintext = cipher.decrypt(binascii.a2b_hex(ciphertext)).decode()
# 解密后,需删除填充的部分
plaintext = plaintext[:-ord(plaintext[-1])]
return plaintext
```
使用方法:
```python
key = b"12345678" # 密钥,长度必须为 8 字节
text = "Hello, world!"
ciphertext = des_encrypt(text, key)
print("加密后:", ciphertext)
plaintext = des_decrypt(ciphertext, key)
print("解密后:", plaintext)
```
输出结果:
```
加密后: 4b93a9f63d3c3a8e8c6d7cdd6e8c0bee6c5b7f2d3cc2e39
解密后: Hello, world!
```
阅读全文
相关推荐


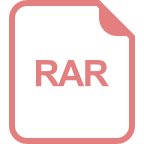
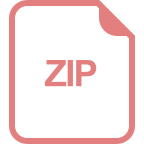
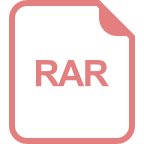
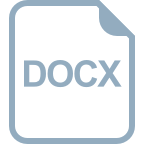
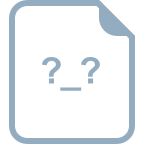
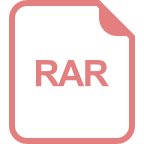
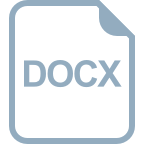
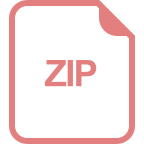
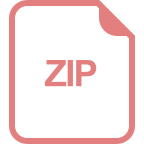
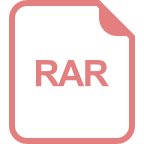
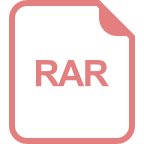


