Ceemdan-lstm代码
时间: 2023-10-05 11:10:23 浏览: 46
以下是使用 PyTorch 实现的 Ceemdan-LSTM 代码示例:
```python
import numpy as np
import torch
import torch.nn as nn
import torch.optim as optim
# 定义 Ceemdan-LSTM 模型
class CeemdanLSTM(nn.Module):
def __init__(self, input_dim, hidden_dim, output_dim, num_layers):
super(CeemdanLSTM, self).__init__()
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.output_dim = output_dim
self.num_layers = num_layers
self.ceemdan = nn.Sequential(
nn.Conv1d(input_dim, hidden_dim, kernel_size=3, stride=1, padding=1),
nn.ReLU(),
nn.Conv1d(hidden_dim, hidden_dim, kernel_size=3, stride=1, padding=1),
nn.ReLU(),
nn.Conv1d(hidden_dim, hidden_dim, kernel_size=3, stride=1, padding=1),
nn.ReLU(),
nn.Conv1d(hidden_dim, hidden_dim, kernel_size=3, stride=1, padding=1),
nn.ReLU(),
nn.Conv1d(hidden_dim, hidden_dim, kernel_size=3, stride=1, padding=1),
nn.ReLU(),
nn.Conv1d(hidden_dim, hidden_dim, kernel_size=3, stride=1, padding=1),
nn.ReLU(),
nn.Conv1d(hidden_dim, hidden_dim, kernel_size=3, stride=1, padding=1),
nn.ReLU(),
nn.Conv1d(hidden_dim, hidden_dim, kernel_size=3, stride=1, padding=1),
nn.ReLU(),
nn.Conv1d(hidden_dim, hidden_dim, kernel_size=3, stride=1, padding=1),
nn.ReLU(),
nn.Conv1d(hidden_dim, hidden_dim, kernel_size=3, stride=1, padding=1),
nn.ReLU()
)
self.lstm = nn.LSTM(hidden_dim, hidden_dim, num_layers=num_layers, batch_first=True)
self.fc = nn.Linear(hidden_dim, output_dim)
def forward(self, x):
x = self.ceemdan(x.permute(0, 2, 1)).permute(0, 2, 1)
h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_dim).to(device)
c0 = torch.zeros(self.num_layers, x.size(0), self.hidden_dim).to(device)
output, _ = self.lstm(x, (h0, c0))
output = self.fc(output[:, -1, :])
return output
# 加载数据
data = np.load('data.npy')
target = np.load('target.npy')
# 将数据转化为 PyTorch 张量
data = torch.from_numpy(data).float()
target = torch.from_numpy(target).float()
# 定义超参数
input_dim = data.shape[2]
hidden_dim = 32
output_dim = 1
num_layers = 1
lr = 0.01
num_epochs = 100
# 定义设备
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
# 初始化模型、损失函数和优化器
model = CeemdanLSTM(input_dim, hidden_dim, output_dim, num_layers).to(device)
criterion = nn.MSELoss()
optimizer = optim.Adam(model.parameters(), lr=lr)
# 训练模型
for epoch in range(num_epochs):
model.train()
optimizer.zero_grad()
output = model(data.to(device))
loss = criterion(output, target.to(device))
loss.backward()
optimizer.step()
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, num_epochs, loss.item()))
# 保存模型
torch.save(model.state_dict(), 'model.pth')
```
相关推荐
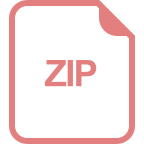
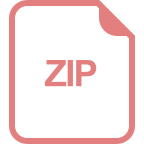
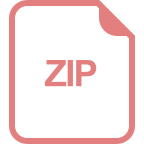







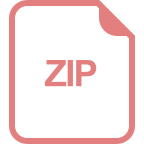
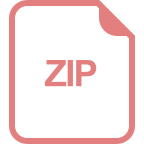
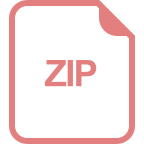
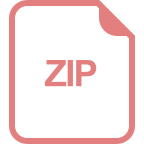
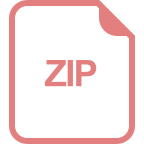
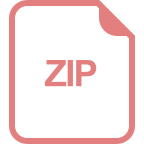
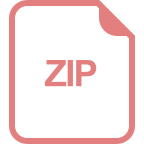
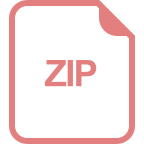
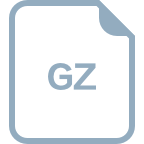