设计老师类和学生类。每个老师有姓名和研究方向,还可以指导若干个研究生。研究生的私有数据成员包括姓名,专业和学号;编写一个程序,创建包含三个教师的数组。输入每个老师的指导所有研究生的信息,输出所有教师的相关信息,包括指导学生情况。 注意:要求不能使用友元函数!
时间: 2023-04-09 07:03:54 浏览: 125
好的,这是一个关于设计老师类和学生类的问题。根据您的描述,老师类应该包含姓名和研究方向两个数据成员,还应该有一个指向研究生的指针。研究生类应该包含姓名、专业和学号三个数据成员。为了实现老师指导研究生的功能,我们可以在老师类中添加一个指导研究生的函数,该函数可以接受一个研究生对象作为参数,并将该研究生对象添加到老师的指导列表中。
下面是一个示例程序,用于创建包含三个教师的数组,并输入每个老师的指导所有研究生的信息,最后输出所有教师的相关信息,包括指导学生情况。
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Student {
public:
Student(string name, string major, string id) : name_(name), major_(major), id_(id) {}
string GetName() const { return name_; }
string GetMajor() const { return major_; }
string GetId() const { return id_; }
private:
string name_;
string major_;
string id_;
};
class Teacher {
public:
Teacher(string name, string research_area) : name_(name), research_area_(research_area) {}
void AddStudent(Student student) {
students_.push_back(student);
}
string GetName() const { return name_; }
string GetResearchArea() const { return research_area_; }
void PrintInfo() const {
cout << "Teacher: " << name_ << endl;
cout << "Research Area: " << research_area_ << endl;
cout << "Students:" << endl;
for (const auto& student : students_) {
cout << "Name: " << student.GetName() << ", Major: " << student.GetMajor() << ", ID: " << student.GetId() << endl;
}
cout << endl;
}
private:
string name_;
string research_area_;
vector<Student> students_;
};
int main() {
Teacher teachers[3] = {
Teacher("Tom", "Computer Science"),
Teacher("Jerry", "Mathematics"),
Teacher("Alice", "Physics")
};
// Input student information for each teacher
for (int i = 0; i < 3; ++i) {
cout << "Input student information for teacher " << teachers[i].GetName() << ":" << endl;
while (true) {
string name, major, id;
cout << "Name: ";
getline(cin, name);
if (name.empty()) {
break;
}
cout << "Major: ";
getline(cin, major);
cout << "ID: ";
getline(cin, id);
Student student(name, major, id);
teachers[i].AddStudent(student);
}
}
// Print teacher information
for (int i = 0; i < 3; ++i) {
teachers[i].PrintInfo();
}
return 0;
}
```
希望这个程序可以帮助您解决问题。如果您有任何其他问题,请随时问我。
阅读全文
相关推荐
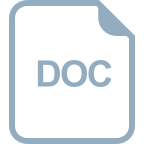
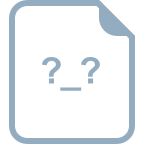

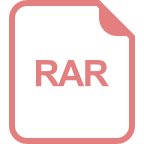
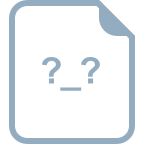
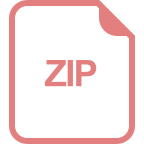
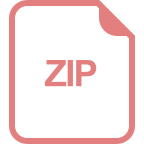
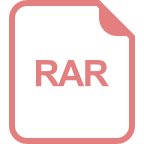
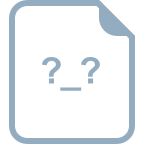
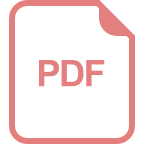
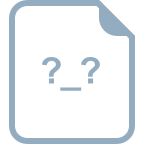
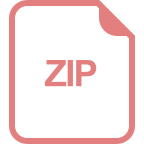
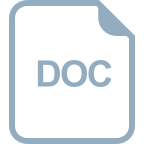
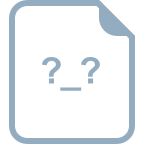
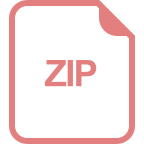
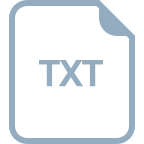
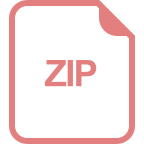