java实现aes-cbc加密
时间: 2023-11-26 15:42:22 浏览: 32
以下是Java实现AES-CBC加密的示例代码:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
public class AESCBC {
private static final String ALGORITHM = "AES/CBC/PKCS5Padding";
private static final byte[] IV = {0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09, 0x0a, 0x0b, 0x0c, 0x0d, 0x0e, 0x0f};
public static byte[] encrypt(byte[] key, byte[] data) throws Exception {
SecretKeySpec keySpec = new SecretKeySpec(key, "AES");
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, keySpec, new IvParameterSpec(IV));
return cipher.doFinal(data);
}
public static byte[] decrypt(byte[] key, byte[] data) throws Exception {
SecretKeySpec keySpec = new SecretKeySpec(key, "AES");
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, keySpec, new IvParameterSpec(IV));
return cipher.doFinal(data);
}
public static void main(String[] args) throws Exception {
byte[] key = "1234567890123456".getBytes();
byte[] data = "Hello World!".getBytes();
byte[] encryptedData = encrypt(key, data);
System.out.println("Encrypted Data: " + new String(encryptedData));
byte[] decryptedData = decrypt(key, encryptedData);
System.out.println("Decrypted Data: " + new String(decryptedData));
}
}
```
在此示例代码中,我们使用AES-CBC算法进行加密和解密。首先,我们定义了算法名称和IV(初始化向量)。然后,我们定义了两个方法encrypt()和decrypt()来实现加密和解密操作。在这些方法中,我们创建一个SecretKeySpec对象,该对象包含密钥。然后,我们创建一个Cipher对象,该对象使用encrypt()方法时传入的密钥、IV和算法进行初始化。然后我们调用doFinal()方法来加密或解密数据。在main()方法中,我们使用一个简单的字符串作为密钥和数据来测试我们的加密和解密方法。
相关推荐
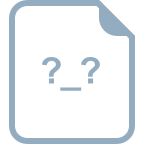
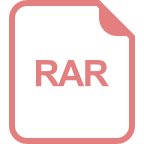
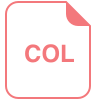













