创建一个计时器,点击开始计时,计时器开始工作,点击结束计时,计时器停止工作,在开始计时前判断当前计时器是否已经开始工作,获取显示时间的p标签,修改页面时间
时间: 2024-05-14 16:12:58 浏览: 12
Sure, I can help you create a timer. Here is the JavaScript code:
```
// Get the p element to display time
let display = document.getElementById("time-display");
let startTime; // variable to store start time
let timerInterval; // setInterval variable for timer
// function to start timer
function startTimer() {
// Check if timer already started
if (startTime) {
alert("Timer already started!");
return;
}
// Get current time and store as start time
startTime = new Date().getTime();
// Call updateTimer function every second
timerInterval = setInterval(updateTimer, 1000);
}
// function to stop timer
function stopTimer() {
// Check if timer not started
if (!startTime) {
alert("Timer not started yet!");
return;
}
// Clear the timer interval
clearInterval(timerInterval);
// Reset start time
startTime = null;
}
// function to update timer display
function updateTimer() {
// Get current time
let currentTime = new Date().getTime();
// Calculate time difference (in seconds)
let timeDiff = (currentTime - startTime) / 1000;
// Calculate minutes and seconds
let minutes = Math.floor(timeDiff / 60);
let seconds = Math.floor(timeDiff % 60);
// Format time display (MM:SS)
let timeDisplay = `${minutes.toString().padStart(2, "0")}:${seconds.toString().padStart(2, "0")}`;
// Update the p element with time display
display.textContent = timeDisplay;
}
```
To start the timer, call the `startTimer()` function. To stop the timer, call the `stopTimer()` function. You need to add an HTML element with `id="time-display"` to display the timer.
相关推荐
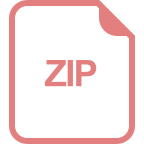
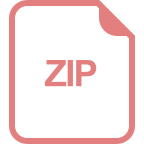














