#include <stdio.h>#include <ctype.h>void stringcount(char *s) { int upper = 0, lower = 0, space = 0, digit = 0, other = 0; while (*s) { if (isupper(*s)) upper++; else if (islower(*s)) lower++; else if (*s == ' ') space++; else if (isdigit(*s)) digit++; else other++; s++; } printf("大写字母:%d\n", upper); printf("小写字母:%d\n", lower); printf("空格:%d\n", space); printf("数字:%d\n", digit); printf("其他字符:%d\n", other);}
时间: 2024-04-26 14:23:10 浏览: 55
这段代码实现了一个函数 stringcount,它接受一个指向字符串的指针参数,然后统计这个字符串中包含的大写字母、小写字母、空格、数字和其他字符的个数,并输出统计结果。该函数使用了 C 标准库中的 isupper、islower、isdigit 函数来判断字符的类型。在 while 循环中,每遍历到一个字符,就根据其类型更新相应的计数器。最后,使用 printf 函数输出统计结果。
相关问题
本题要求实现一个函数,统计给定字符串中的大写字母、小写字母、空格、数字以及其他字符各有多少。 函数接口定义如下: void stringcount(char *s) 其中*s为要统计的字符串,统计结果可以直接输出或保存在变量中。
好的,我会根据您的要求进行回答。以下是实现该函数的代码:
```c
#include <stdio.h>
#include <ctype.h>
void stringcount(char *s) {
int upper = 0, lower = 0, space = 0, digit = 0, other = 0;
while (*s) {
if (isupper(*s))
upper++;
else if (islower(*s))
lower++;
else if (*s == ' ')
space++;
else if (isdigit(*s))
digit++;
else
other++;
s++;
}
printf("大写字母:%d\n", upper);
printf("小写字母:%d\n", lower);
printf("空格:%d\n", space);
printf("数字:%d\n", digit);
printf("其他字符:%d\n", other);
}
```
该函数中使用了ctype.h头文件中的函数,isupper、islower、isdigit分别用于判断字符是否为大写字母、小写字母、数字。函数中定义了五个变量,分别统计五种字符的数量。然后使用while循环遍历字符串中的每一个字符,根据字符的类型进行相应的计数。最后输出各种字符的数量。
调用该函数的方式如下:
```c
int main() {
char str[] = "Hello, World! 123";
stringcount(str);
return 0;
}
```
输出结果为:
```
大写字母:2
小写字母:8
空格:2
数字:3
其他字符:2
```
不使用ctype.h
如果你不想使用C标准库中的`ctype.h`头文件,可以手动创建一个大写字母到小写字母的映射表来进行转换。下面是一个简单的实现,不依赖于库函数:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 100
#define LOWER ('a')
#define UPPER ('A')
char lower_map[26] = {'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z'};
char upper_map[26] = {'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z'};
void toLowerCase(char str[]) {
int len = strlen(str);
for (int i = 0; i < len; i++) {
if (str[i] >= UPPER && str[i] <= UPPER + 25) { // 判断是否为大写字母
str[i] = lower_map[str[i] - UPPER]; // 转换为对应的小写字母
}
}
}
int main() {
char input[MAX_LEN];
printf("请输入一行英文:");
fgets(input, MAX_LEN, stdin); // 读取用户输入,包括换行符
// 移除fgets读取的换行符
if (input[strlen(input) - 1] == '\n') {
input[strlen(input) - 1] = '\0'; // 修改字符串结束字符
}
toLowerCase(input);
printf("转换后的字符串:%s\n", input);
return 0;
}
阅读全文
相关推荐
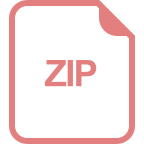
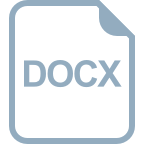
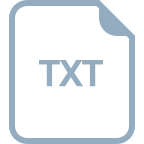





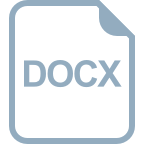
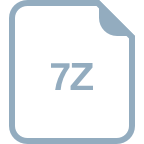
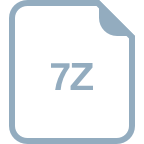
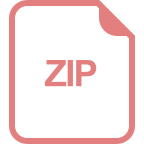
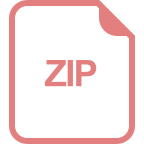