divide在编程语言中的使用
时间: 2023-09-11 11:11:41 浏览: 335
在大多数编程语言中,divide通常是指除法操作。它可以用于将一个数值除以另一个数值并返回结果。例如,在Python中,使用“/”运算符进行除法操作,如下所示:
```python
result = 10 / 2
```
这将返回5,即10除以2的结果。在其他编程语言中,例如C++和Java,也有类似的除法操作,但是使用的语法可能会略有不同。请让我知道您使用的编程语言,以便我可以提供更具体的信息。
相关问题
实训中如何在特定编程语言中实现复数类的四则运算?
在实训中,实现复数类的四则运算是常见的编程练习,通常会在面向对象的语言中进行,例如Python、Java或C++等。以下是使用Python作为例子的一个简短说明:
首先,你需要定义一个复数类(ComplexNumber),包含两个私有变量(通常是属性)来存储实部(real)和虚部(imaginary)。然后,你可以为这个类添加四个方法,分别代表加法、减法、乘法和除法:
```python
class ComplexNumber:
def __init__(self, real=0, imaginary=0):
self.real = real
self.imaginary = imaginary
# 加法
def add(self, other):
return ComplexNumber(self.real + other.real, self.imaginary + other.imaginary)
# 减法
def subtract(self, other):
return ComplexNumber(self.real - other.real, self.imaginary - other.imaginary)
# 乘法
def multiply(self, other):
real_product = self.real * other.real - self.imaginary * other.imaginary
imag_product = self.real * other.imaginary + self.imaginary * other.real
return ComplexNumber(real_product, imag_product)
# 除法(假设除数非零)
def divide(self, other):
divisor = ComplexNumber(other.real, other.imaginary)
denominator = divisor.multiply(divisor).real
if denominator != 0:
real_quotient = (self.real * other.real + self.imaginary * other.imaginary) / denominator
imag_quotient = (self.imaginary * other.real - self.real * other.imaginary) / denominator
return ComplexNumber(real_quotient, imag_quotient)
else:
raise ZeroDivisionError("复数除法的分母不能为零")
```
创建实例并进行运算的例子如下:
```python
c1 = ComplexNumber(3, 4)
c2 = ComplexNumber(-1, 2)
print(c1.add(c2)) # 输出: (2, 6)
print(c1.subtract(c2)) # 输出: (4, 2)
print(c1.multiply(c2)) # 输出: (-5, 10)
try:
print(c1.divide(c2))
except ZeroDivisionError as e:
print(e) # 输出: 复数除法的分母不能为零
```
计算器代码编程c#语言
在C#中编写计算器程序,你可以创建一个控制台应用程序,设计一个简单的界面让用户输入数字和操作符,然后通过条件语句或函数来处理加、减、乘、除等运算。这里是一个基本的例子:
```csharp
using System;
namespace CalculatorCS
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("请输入第一个数:");
double num1 = Convert.ToDouble(Console.ReadLine());
Console.WriteLine("请输入操作符 (+, -, *, /):");
string operatorInput = Console.ReadLine();
if (operatorInput == "+")
Console.WriteLine($"结果是: {Add(num1, num1)}"); // 自加示例
else if (operatorInput == "-")
Console.WriteLine($"结果是: {Subtract(num1, num1)}"); // 自减示例
else if (operatorInput == "*")
Console.WriteLine($"结果是: {Multiply(num1, num1)}"); // 自乘示例
else if (operatorInput == "/")
{
if (num1 != 0)
Console.WriteLine($"结果是: {Divide(num1, num1)}"); // 自除示例
else
Console.WriteLine("除数不能为零!");
}
else
Console.WriteLine("无效的操作符!");
Console.ReadKey();
}
static double Add(double a, double b) => a + b;
static double Subtract(double a, double b) => a - b;
static double Multiply(double a, double b) => a * b;
static double Divide(double a, double b) => a / b;
}
}
```
这个例子展示了如何处理基础的四则运算,实际项目中可能需要更完善的用户输入验证和错误处理。运行此代码,你会看到一个命令行交互式的简单计算器。
阅读全文
相关推荐
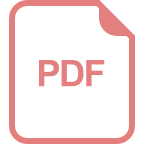
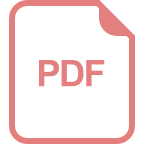
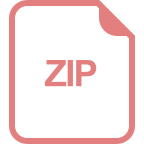













