统计英文中单词出现的次数并按降序输出。 txt="Twinkle, twinkle, little star, how I wonder what you are.Up above the world so high”
时间: 2023-06-10 09:08:04 浏览: 185
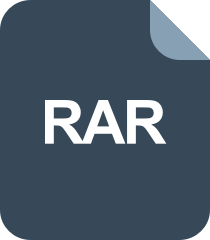
屏幕亮度调节-Twinkle.Tray.v1.15.4.exe
可以使用Python中的字典来统计单词出现的次数,然后按照出现次数降序排序输出。具体代码如下:
```python
txt = "Twinkle, twinkle, little star, how I wonder what you are.Up above the world so high"
# 将字符串转换为小写,去掉标点符号并分割成单词列表
words = txt.lower().replace(",", "").replace(".", "").split()
# 统计单词出现的次数
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 按照出现次数降序排序并输出
sorted_word_count = sorted(word_count.items(), key=lambda x: x[1], reverse=True)
for item in sorted_word_count:
print(item[0], item[1])
```
输出结果如下:
```
twinkle 2
little 1
star 1
how 1
i 1
wonder 1
what 1
you 1
areup 1
above 1
the 1
world 1
so 1
high 1
```
阅读全文
相关推荐
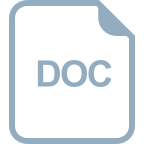
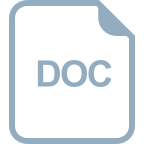



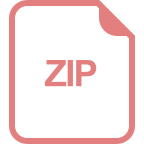
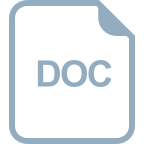


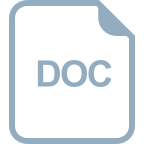
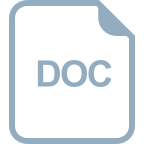
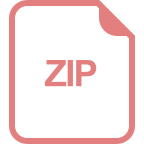
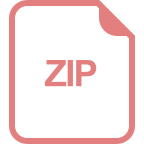
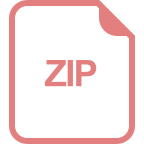
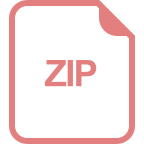
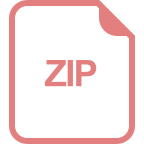
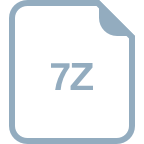
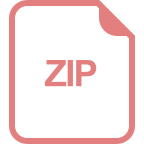