gd32串口与基于ISO/IEC 14443 Type A标准的读卡器通信的c语言程序
时间: 2024-03-23 10:38:07 浏览: 55
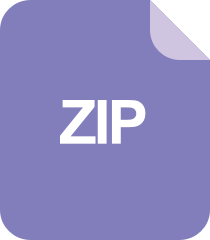
与读卡器连接的一个cgi程序
好的,下面是一个简单的基于ISO/IEC 14443 Type A标准的读卡器与GD32串口通信的C语言程序:
```c
#include <stdio.h>
#include "gd32f10x.h"
#define RST_PIN GPIO_PIN_2
#define RST_PORT GPIOB
#define IRQ_PIN GPIO_PIN_3
#define IRQ_PORT GPIOB
#define BUFFER_SIZE 64
uint8_t rx_buffer[BUFFER_SIZE];
uint8_t tx_buffer[BUFFER_SIZE];
uint8_t command[] = {0x52, 0x01, 0x00, 0x00, 0x00};
void uart_init(void)
{
/* enable GPIO clock */
rcu_periph_clock_enable(RCU_GPIOA);
/* enable USART clock */
rcu_periph_clock_enable(RCU_USART0);
/* configure USART0 Tx and Rx as alternate function push-pull */
gpio_init(GPIOA, GPIO_MODE_AF_PP, GPIO_OSPEED_50MHZ, GPIO_PIN_9);
gpio_init(GPIOA, GPIO_MODE_IN_FLOATING, GPIO_OSPEED_50MHZ, GPIO_PIN_10);
/* configure USART0 */
usart_deinit(USART0);
usart_baudrate_set(USART0, 115200);
usart_word_length_set(USART0, USART_WL_8BIT);
usart_stop_bit_set(USART0, USART_STB_1BIT);
usart_parity_config(USART0, USART_PM_NONE);
usart_hardware_flow_rts_config(USART0, USART_RTS_DISABLE);
usart_hardware_flow_cts_config(USART0, USART_CTS_DISABLE);
usart_receive_config(USART0, USART_RECEIVE_ENABLE);
usart_transmit_config(USART0, USART_TRANSMIT_ENABLE);
/* enable USART0 */
usart_enable(USART0);
}
void spi_init(void)
{
/* enable SPI clock */
rcu_periph_clock_enable(RCU_SPI1);
/* configure SPI1 GPIO */
gpio_init(GPIOA, GPIO_MODE_AF_PP, GPIO_OSPEED_50MHZ, GPIO_PIN_5);
gpio_init(GPIOA, GPIO_MODE_AF_PP, GPIO_OSPEED_50MHZ, GPIO_PIN_6);
gpio_init(GPIOA, GPIO_MODE_AF_PP, GPIO_OSPEED_50MHZ, GPIO_PIN_7);
/* configure SPI1 */
spi_parameter_struct spi_init_struct;
spi_init_struct.device_mode = SPI_SLAVE;
spi_init_struct.trans_mode = SPI_TRANSMODE_FULLDUPLEX;
spi_init_struct.frame_size = SPI_FRAMESIZE_8BIT;
spi_init_struct.clock_polarity_phase = SPI_CK_PL_LOW_PH_1EDGE;
spi_init_struct.nss = SPI_NSS_SOFT;
spi_init_struct.prescale = SPI_PSC_64;
spi_init_struct.endian = SPI_ENDIAN_MSB;
spi_init(SPI1, &spi_init_struct);
/* enable SPI1 */
spi_enable(SPI1);
}
void reset_reader(void)
{
gpio_bit_reset(RST_PORT, RST_PIN);
delay_1ms(10);
gpio_bit_set(RST_PORT, RST_PIN);
delay_1ms(100);
}
void send_command(uint8_t *command, uint8_t len)
{
uint8_t i;
for (i = 0; i < len; i++) {
while (RESET == usart_flag_get(USART0, USART_FLAG_TBE));
usart_data_transmit(USART0, command[i]);
}
}
void receive_response(uint8_t *response, uint8_t len)
{
uint8_t i;
for (i = 0; i < len; i++) {
while (RESET == usart_flag_get(USART0, USART_FLAG_RBNE));
response[i] = usart_data_receive(USART0);
}
}
int main(void)
{
uart_init();
spi_init();
gpio_init(RST_PORT, GPIO_MODE_OUT_PP, GPIO_OSPEED_50MHZ, RST_PIN);
gpio_init(IRQ_PORT, GPIO_MODE_IN_FLOATING, GPIO_OSPEED_50MHZ, IRQ_PIN);
reset_reader();
while (1) {
send_command(command, sizeof(command));
receive_response(rx_buffer, BUFFER_SIZE);
spi_i2s_data_transmit(SPI1, rx_buffer[0]);
spi_i2s_data_receive(SPI1);
}
}
```
这个程序使用的是GD32F103芯片的USART0串口和SPI1接口,其中USART0串口用于与读卡器通信,SPI1接口用于将读卡器的响应数据传输到主控芯片中。程序中的send_command()函数用于向读卡器发送指令,receive_response()函数用于接收读卡器的响应数据,reset_reader()函数用于复位读卡器。程序的主循环中,通过调用send_command()函数发送指令,然后调用receive_response()函数接收读卡器的响应数据,并将响应数据通过SPI1接口传输到主控芯片中。
阅读全文
相关推荐
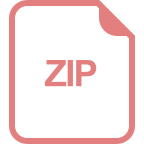
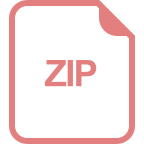
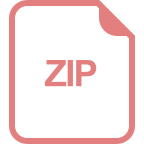
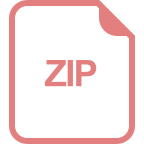
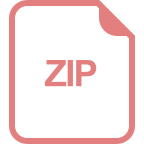
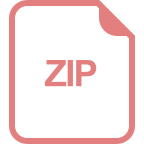
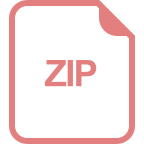
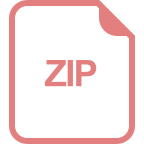
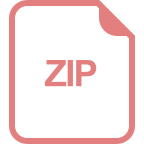
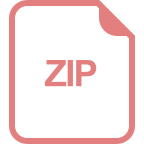
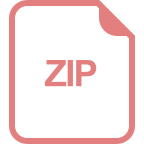
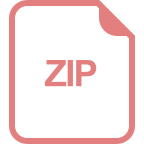