java复制文件夹中的所有文件和文件夹到另一个文件夹中
时间: 2023-05-12 12:03:51 浏览: 84
可以使用Java的File类和递归函数来实现复制文件夹中的所有文件和文件夹到另一个文件夹中。具体实现可以参考以下代码:
```
import java.io.File;
import java.io.IOException;
public class FileCopy {
public static void main(String[] args) {
String sourcePath = "sourceFolder";
String destPath = "destFolder";
File sourceFolder = new File(sourcePath);
File destFolder = new File(destPath);
try {
copyFolder(sourceFolder, destFolder);
System.out.println("Folder copied successfully.");
} catch (IOException e) {
e.printStackTrace();
}
}
public static void copyFolder(File sourceFolder, File destFolder) throws IOException {
if (sourceFolder.isDirectory()) {
if (!destFolder.exists()) {
destFolder.mkdir();
}
String[] files = sourceFolder.list();
for (String file : files) {
File srcFile = new File(sourceFolder, file);
File destFile = new File(destFolder, file);
copyFolder(srcFile, destFile);
}
} else {
java.nio.file.Files.copy(sourceFolder.toPath(), destFolder.toPath(),
java.nio.file.StandardCopyOption.REPLACE_EXISTING);
}
}
}
```
这个程序会将sourceFolder文件夹中的所有文件和文件夹复制到destFolder文件夹中。如果destFolder不存在,则会创建一个新的文件夹。
相关推荐
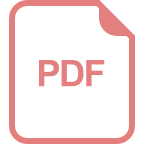
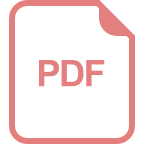
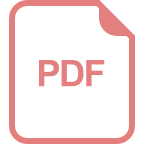
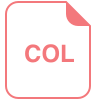
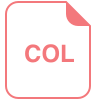
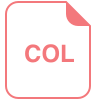
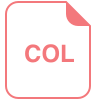
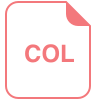









