Spring AOP——基于注解的AOP实现
时间: 2023-10-23 14:13:03 浏览: 175
Spring AOP是Spring框架中的一个重要模块,它提供了面向切面编程(AOP)的支持。AOP是一种编程思想,它可以在不改变原有代码的情况下,通过在程序运行时动态地将代码“织入”到现有代码中,从而实现对原有代码的增强。
Spring AOP提供了基于注解的AOP实现,使得开发者可以通过注解的方式来定义切面、切点和通知等相关内容,从而简化了AOP的使用。
下面是一个基于注解的AOP实现的例子:
1. 定义切面类
```java
@Aspect
@Component
public class LogAspect {
@Pointcut("@annotation(Log)")
public void logPointcut() {}
@Before("logPointcut()")
public void beforeLog(JoinPoint joinPoint) {
// 前置通知
System.out.println("执行方法:" + joinPoint.getSignature().getName());
}
@AfterReturning("logPointcut()")
public void afterLog(JoinPoint joinPoint) {
// 后置通知
System.out.println("方法执行完成:" + joinPoint.getSignature().getName());
}
@AfterThrowing(pointcut = "logPointcut()", throwing = "ex")
public void afterThrowingLog(JoinPoint joinPoint, Exception ex) {
// 异常通知
System.out.println("方法执行异常:" + joinPoint.getSignature().getName() + ",异常信息:" + ex.getMessage());
}
}
```
2. 定义业务逻辑类
```java
@Service
public class UserService {
@Log
public void addUser(User user) {
// 添加用户
System.out.println("添加用户:" + user.getName());
}
@Log
public void deleteUser(String userId) {
// 删除用户
System.out.println("删除用户:" + userId);
throw new RuntimeException("删除用户异常");
}
}
```
3. 在配置文件中开启AOP
```xml
<aop:aspectj-autoproxy/>
<context:component-scan base-package="com.example"/>
```
在这个例子中,我们定义了一个切面类LogAspect,其中通过@Aspect注解定义了一个切面,通过@Pointcut注解定义了一个切点,通过@Before、@AfterReturning和@AfterThrowing注解分别定义了前置通知、后置通知和异常通知。
在业务逻辑类中,我们通过@Log注解标注了需要增强的方法。
最后,在配置文件中,我们通过<aop:aspectj-autoproxy/>开启了AOP功能,并通过<context:component-scan>扫描了指定包下的所有组件。
这样,当我们调用UserService中的方法时,就会触发LogAspect中定义的通知,从而实现对原有代码的增强。
阅读全文
相关推荐
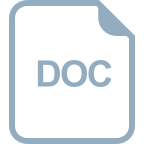
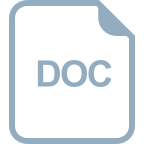
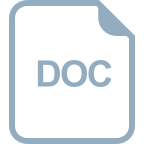
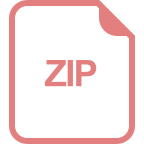
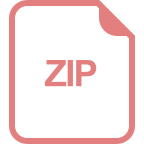
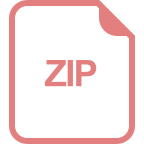
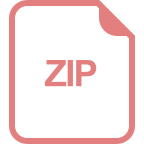
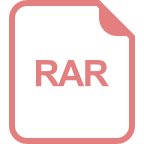
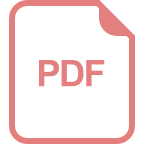
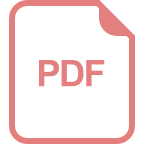
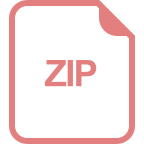
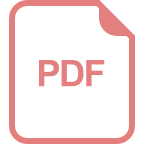
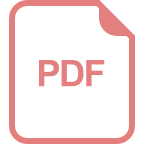
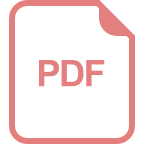
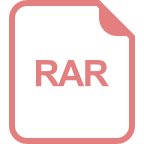
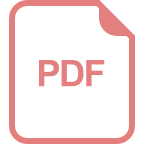
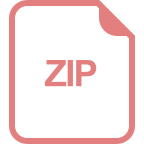