稀疏自编码器降维 tensorflow
时间: 2023-08-29 12:10:57 浏览: 85
稀疏自编码器是一种能够学习数据中最重要特征的神经网络模型。它可以通过对输入数据进行压缩表示,从而对数据进行降维。在 tensorflow 中实现稀疏自编码器的步骤如下:
1. 导入 tensorflow 库
```python
import tensorflow as tf
```
2. 定义输入数据的占位符
```python
X = tf.placeholder("float", [None, n_input])
```
其中,n_input 是输入数据的维度。
3. 定义自编码器的参数
```python
n_hidden_1 = 256 # 第一层隐藏层神经元个数
n_hidden_2 = 128 # 第二层隐藏层神经元个数
n_output = n_input # 输出层神经元个数
weights = {
'encoder_h1': tf.Variable(tf.random_normal([n_input, n_hidden_1])),
'encoder_h2': tf.Variable(tf.random_normal([n_hidden_1, n_hidden_2])),
'decoder_h1': tf.Variable(tf.random_normal([n_hidden_2, n_hidden_1])),
'decoder_h2': tf.Variable(tf.random_normal([n_hidden_1, n_output])),
}
biases = {
'encoder_b1': tf.Variable(tf.random_normal([n_hidden_1])),
'encoder_b2': tf.Variable(tf.random_normal([n_hidden_2])),
'decoder_b1': tf.Variable(tf.random_normal([n_hidden_1])),
'decoder_b2': tf.Variable(tf.random_normal([n_output])),
}
```
4. 定义自编码器模型
```python
def encoder(x):
layer_1 = tf.nn.sigmoid(tf.add(tf.matmul(x, weights['encoder_h1']), biases['encoder_b1']))
layer_2 = tf.nn.sigmoid(tf.add(tf.matmul(layer_1, weights['encoder_h2']), biases['encoder_b2']))
return layer_2
def decoder(x):
layer_1 = tf.nn.sigmoid(tf.add(tf.matmul(x, weights['decoder_h1']), biases['decoder_b1']))
layer_2 = tf.nn.sigmoid(tf.add(tf.matmul(layer_1, weights['decoder_h2']), biases['decoder_b2']))
return layer_2
encoder_op = encoder(X)
decoder_op = decoder(encoder_op)
```
其中,encoder() 函数实现了自编码器的编码过程,decoder() 函数实现了自编码器的解码过程。encoder_op 和 decoder_op 分别是编码和解码的输出结果。
5. 定义损失函数
```python
cost = tf.reduce_mean(tf.pow(X - decoder_op, 2))
```
6. 定义优化器
```python
optimizer = tf.train.AdamOptimizer(learning_rate).minimize(cost)
```
其中,learning_rate 是学习率。
7. 训练模型
```python
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for epoch in range(training_epochs):
_, c = sess.run([optimizer, cost], feed_dict={X: batch_xs})
if epoch % display_step == 0:
print("Epoch:", '%04d' % (epoch+1), "cost=", "{:.9f}".format(c))
print("Optimization Finished!")
encode_decode = sess.run(
decoder_op, feed_dict={X: mnist.test.images[:examples_to_show]})
```
其中,batch_xs 是训练数据,examples_to_show 是展示结果的样本数量。
8. 可视化结果
```python
import matplotlib.pyplot as plt
f, a = plt.subplots(2, 10, figsize=(10, 2))
for i in range(examples_to_show):
a[0][i].imshow(np.reshape(mnist.test.images[i], (28, 28)))
a[1][i].imshow(np.reshape(encode_decode[i], (28, 28)))
plt.show()
```
其中,第一行展示了原始图像,第二行展示了经过自编码器压缩和解码后的图像。
阅读全文
相关推荐
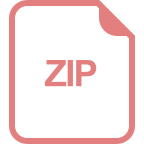



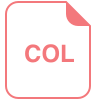


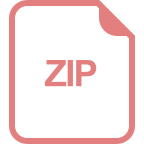
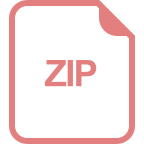
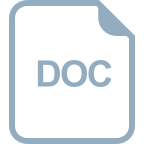
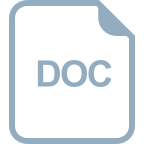
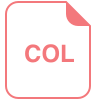
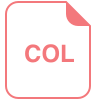
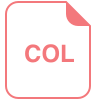
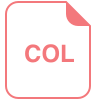