java实现文件预览部分内容再点击按钮操作完别的内容后再预览全部内容
时间: 2023-11-18 15:03:00 浏览: 42
您可以使用Java的RandomAccessFile类来实现这个功能。以下是一种可能的实现方式:
1. 创建一个RandomAccessFile对象,打开要预览的文件。
2. 使用readLine()方法读取文件中的一行或者使用read(byte[])方法读取指定长度的字节数据,以此来预览文件的部分内容。
3. 创建一个按钮,当用户点击时,执行操作完别的内容后再预览全部内容的操作。
4. 在按钮的事件处理方法中,使用seek()方法将文件指针移动到文件的开头。
5. 使用read(byte[])方法读取文件的全部内容,并将其显示到用户界面中。
以下是一个示例代码:
```
import java.io.RandomAccessFile;
import javax.swing.*;
public class FilePreview {
public static void main(String[] args) {
JFrame frame = new JFrame("File Preview");
JTextArea textArea = new JTextArea();
JButton previewButton = new JButton("Preview");
previewButton.addActionListener(e -> {
try {
RandomAccessFile file = new RandomAccessFile("file.txt", "r");
String line = file.readLine();
while (line != null && textArea.getLineCount() < 10) {
textArea.append(line + "\n");
line = file.readLine();
}
file.close();
} catch (Exception ex) {
ex.printStackTrace();
}
});
JButton fullPreviewButton = new JButton("Full Preview");
fullPreviewButton.addActionListener(e -> {
try {
RandomAccessFile file = new RandomAccessFile("file.txt", "r");
byte[] bytes = new byte[(int) file.length()];
file.read(bytes);
String content = new String(bytes);
textArea.setText(content);
file.close();
} catch (Exception ex) {
ex.printStackTrace();
}
});
JPanel buttonPanel = new JPanel();
buttonPanel.add(previewButton);
buttonPanel.add(fullPreviewButton);
frame.add(new JScrollPane(textArea));
frame.add(buttonPanel, "South");
frame.setSize(400, 300);
frame.setLocationRelativeTo(null);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
```
在这个示例中,我们使用了一个JTextArea组件来显示文件内容。当用户点击"Preview"按钮时,我们读取文件的前10行内容并将其显示在JTextArea中。当用户点击"Full Preview"按钮时,我们读取文件的全部内容并将其显示在JTextArea中。注意,这个示例仅针对文本文件,如果需要预览其他类型的文件,需要使用不同的方法来读取文件内容。
相关推荐
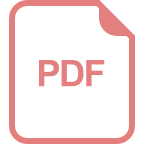
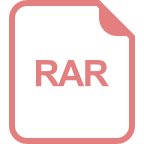
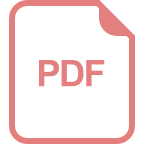














