android studio实现评论区代码
时间: 2023-06-30 21:24:06 浏览: 291
要在Android Studio中实现评论区,需要做以下几个步骤:
1. 在XML布局文件中添加评论区的UI元素,例如EditText和Button控件,用于输入评论和提交评论。
2. 在Java代码中,设置Button的点击监听器,当点击按钮时,获取EditText中的评论内容,将其发送给服务器。
3. 服务器接收到评论内容后,将其存储在数据库中。
4. 当用户请求查看评论时,客户端向服务器请求数据,服务器将存储在数据库中的评论内容返回给客户端。
5. 客户端将评论内容显示在评论区中,例如使用ListView或RecyclerView控件。
以下是一个简单的示例代码,用于实现评论区:
XML布局文件:
```
<EditText
android:id="@+id/commentEditText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="输入评论" />
<Button
android:id="@+id/submitButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="提交" />
<ListView
android:id="@+id/commentListView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
```
Java代码:
```
public class MainActivity extends AppCompatActivity {
private EditText commentEditText;
private Button submitButton;
private ListView commentListView;
private CommentAdapter commentAdapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
commentEditText = findViewById(R.id.commentEditText);
submitButton = findViewById(R.id.submitButton);
commentListView = findViewById(R.id.commentListView);
// 设置提交按钮的点击监听器
submitButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String comment = commentEditText.getText().toString();
// 将评论发送给服务器
sendComment(comment);
}
});
// 请求评论数据并更新UI
getComments();
}
private void sendComment(String comment) {
// TODO: 将评论发送给服务器
}
private void getComments() {
// TODO: 请求评论数据并更新UI
// 示例代码:
List<Comment> comments = new ArrayList<>();
comments.add(new Comment("user1", "comment1"));
comments.add(new Comment("user2", "comment2"));
comments.add(new Comment("user3", "comment3"));
commentAdapter = new CommentAdapter(this, comments);
commentListView.setAdapter(commentAdapter);
}
}
```
评论数据的模型类:
```
public class Comment {
private String user;
private String content;
public Comment(String user, String content) {
this.user = user;
this.content = content;
}
public String getUser() {
return user;
}
public String getContent() {
return content;
}
}
```
评论区的适配器:
```
public class CommentAdapter extends BaseAdapter {
private Context context;
private List<Comment> comments;
public CommentAdapter(Context context, List<Comment> comments) {
this.context = context;
this.comments = comments;
}
@Override
public int getCount() {
return comments.size();
}
@Override
public Comment getItem(int position) {
return comments.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
if (convertView == null) {
convertView = LayoutInflater.from(context).inflate(R.layout.comment_item, parent, false);
}
TextView userTextView = convertView.findViewById(R.id.userTextView);
TextView contentTextView = convertView.findViewById(R.id.contentTextView);
Comment comment = getItem(position);
userTextView.setText(comment.getUser());
contentTextView.setText(comment.getContent());
return convertView;
}
}
```
评论区的列表项布局文件:
```
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:id="@+id/userTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textStyle="bold" />
<TextView
android:id="@+id/contentTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
```
这是一个简单的评论区示例,具体实现可能需要根据实际需求进行调整。
阅读全文
相关推荐
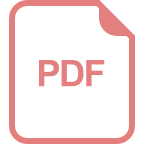
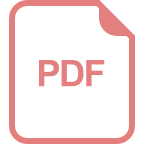
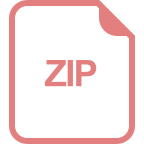
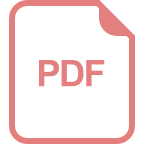
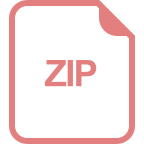
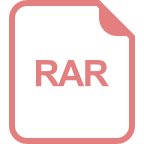
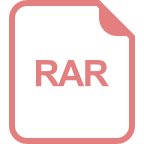
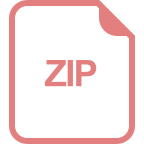
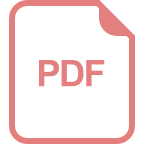
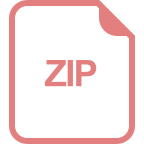
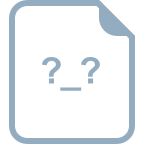
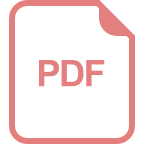
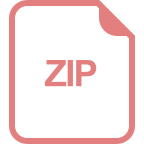
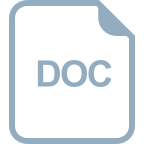
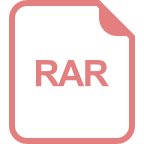