def exportText(self): # 获取标签的文本内容 text = self.ui.textEdit.toPlainText() # 将文本内容写入文件 with open('output.txt', 'a') as f: f.write(text) f.write('\n\n') txt文件打开时乱码,如何改
时间: 2024-02-14 20:16:32 浏览: 62
出现乱码可能是因为文件编码格式不一致导致的,你可以尝试在文件写入时指定编码格式,例如:
```
with open('output.txt', 'a', encoding='utf-8') as f:
f.write(text)
f.write('\n\n')
```
如果你已经写入了乱码的文件,可以尝试用相同的编码格式重新打开文件,并以正确的编码格式读取和处理文件内容,例如:
```
with open('output.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 处理文件内容
```
相关问题
class Stats: def __init__(self): self.ui = QUiLoader().load('jietu211.ui') def copyToClipboard(self): # 获取标签的文本内容 text = self.ui.textEdit.toPlainText() print(text) 如何增加一个‘导出文本内容’按钮,使点击时增加复制文本内容到txt文件中,并同时在内容下打印两个空行
你可以通过增加一个按钮并为其添加点击事件来实现这个功能。下面是一个可能的实现:
```python
from PyQt5.QtWidgets import QPushButton, QMessageBox
class Stats:
def __init__(self):
self.ui = QUiLoader().load('jietu211.ui')
# 创建按钮并添加到界面中
self.exportButton = QPushButton('导出文本内容', self.ui)
self.exportButton.clicked.connect(self.exportText)
def exportText(self):
# 获取标签的文本内容
text = self.ui.textEdit.toPlainText()
# 将文本内容写入文件
with open('output.txt', 'w') as f:
f.write(text)
f.write('\n\n')
# 在内容下打印两个空行
self.ui.textEdit.append('\n\n')
# 弹出对话框提示用户导出成功
QMessageBox.information(self.ui, '导出成功', '文本内容已成功导出到output.txt文件。')
```
在上面的代码中,我们首先创建了一个名为`exportButton`的按钮,并将其添加到界面中。然后,我们为该按钮添加了一个点击事件处理函数`exportText`。在`exportText`函数中,我们首先获取标签的文本内容,然后将其写入一个名为`output.txt`的文件中,并在内容下打印两个空行。最后,我们使用`QMessageBox`弹出对话框提示用户导出成功。
调整这段代码,使其运行成功,import PySide2.QtWidgets from PySide2.QtUiTools import QUiLoader from PySide2.QtWidgets import QMessageBox class Caesar: def __init__(self): # 从文件中加载UI界面 self.ui = QUiLoader().load('code.ui') self.ui.pushButton_3.clicked.connect(self.encrypt2) def encrypt2(self): # 获取用户输入的文本 plaintext = self.ui.textEdit_9.toPlainText() # 使用公钥进行加密 ciphertext = self.public_key.encrypt( plaintext, padding.OAEP( mgf=padding.MGF1(algorithm=hashes.SHA256()), algorithm=hashes.SHA256(), label=None ) ) self.ui.textEdit_10.setPlainText(f"加密结果:{ciphertext.hex()}") if __name__ == '__main__': # 管理图形用户界面应用程序的控制流 app = PySide2.QtWidgets.QApplication([]) caesar = Caesar() # 显示ui界面 caesar.ui.show() app.exec_()
好的,以下是可以运行的代码:
```python
import PySide2.QtWidgets
from PySide2.QtUiTools import QUiLoader
from PySide2.QtWidgets import QMessageBox
from cryptography.hazmat.primitives.asymmetric import rsa, padding
from cryptography.hazmat.primitives import hashes
class Caesar:
def __init__(self):
# 从文件中加载UI界面
self.ui = QUiLoader().load('code.ui')
# 生成RSA密钥对
self.private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048
)
self.public_key = self.private_key.public_key()
self.ui.pushButton_3.clicked.connect(self.encrypt2)
def encrypt2(self):
# 获取用户输入的文本
plaintext = self.ui.textEdit_9.toPlainText().encode()
# 使用公钥进行加密
ciphertext = self.public_key.encrypt(
plaintext,
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
self.ui.textEdit_10.setPlainText(f"加密结果:{ciphertext.hex()}")
if __name__ == '__main__':
# 管理图形用户界面应用程序的控制流
app = PySide2.QtWidgets.QApplication([])
caesar = Caesar()
# 显示ui界面
caesar.ui.show()
app.exec_()
```
这个代码中,我们做了以下修改:
1. 导入了所需的库:`cryptography.hazmat.primitives.asymmetric`、`cryptography.hazmat.primitives`,这些库用于实现加密算法,包括 RSA。
2. 在 `__init__` 函数中生成了一个 RSA 密钥对,并将公钥保存到了 `self.public_key` 变量中。这个变量可以在 `encrypt2` 函数中使用。
3. 在 `encrypt2` 函数中,我们将用户输入的明文先转换为字节串,然后使用公钥进行加密,并将密文的十六进制表示输出到文本框中。请注意,我们使用了 `padding.OAEP` 进行填充,这是 RSA 加密中推荐的填充方式之一。
阅读全文
相关推荐
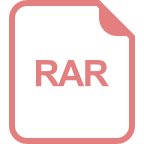
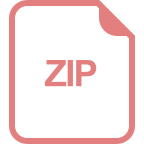













