结合着stat和目录操作实现完整的ls-l 功能
时间: 2024-05-06 20:21:01 浏览: 8
好的,这个问题是关于Linux系统命令的,可以直接回答。可以使用stat和目录操作实现ls -l功能,具体实现可以参考以下代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <dirent.h>
#include <time.h>
#include <sys/stat.h>
#include <pwd.h>
#include <grp.h>
int main(int argc, char *argv[])
{
DIR *dp;
struct dirent *dirp;
struct stat buf;
struct passwd *pwd;
struct group *grp;
char s[100];
if(argc != 2)
{
fprintf(stderr, "Usage: %s directory_name\n", argv[0]);
exit(1);
}
if((dp = opendir(argv[1])) == NULL)
{
fprintf(stderr, "Can't open %s\n", argv[1]);
exit(1);
}
while((dirp = readdir(dp)) != NULL)
{
sprintf(s, "%s/%s", argv[1], dirp->d_name);
if(stat(s, &buf) == -1)
continue;
printf("%d ", buf.st_blocks);
printf((buf.st_mode & S_IRUSR) ? "r" : "-");
printf((buf.st_mode & S_IWUSR) ? "w" : "-");
printf((buf.st_mode & S_IXUSR) ? "x" : "-");
printf((buf.st_mode & S_IRGRP) ? "r" : "-");
printf((buf.st_mode & S_IWGRP) ? "w" : "-");
printf((buf.st_mode & S_IXGRP) ? "x" : "-");
printf((buf.st_mode & S_IROTH) ? "r" : "-");
printf((buf.st_mode & S_IWOTH) ? "w" : "-");
printf((buf.st_mode & S_IXOTH) ? "x" : "-");
printf(" %d ", (int)buf.st_nlink);
if((pwd = getpwuid(buf.st_uid)) != NULL)
printf("%s ", pwd->pw_name);
else
printf("%d ", buf.st_uid);
if((grp = getgrgid(buf.st_gid)) != NULL)
printf("%s ", grp->gr_name);
else
printf("%d ", buf.st_gid);
printf("%6d ", (int)buf.st_size);
printf("%.12s ", 4+ctime(&buf.st_mtime));
printf("%s\n", dirp->d_name);
}
closedir(dp);
exit(0);
}
```
以上是一个简单的实现代码,它实现了类似于Linux的ls -l命令的功能,遍历指定目录下的所有文件,打印出所有文件的详细信息,包括文件名、权限、大小、所属用户和组、最后修改时间等。
相关推荐
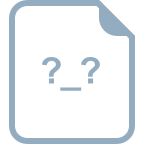
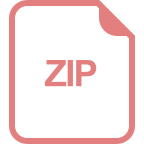














