任意输入一组数据作为二叉排序树中结点的键值,创建一棵二叉排序树,然后对给定的值进行查找。c语言
时间: 2024-02-01 10:13:31 浏览: 67
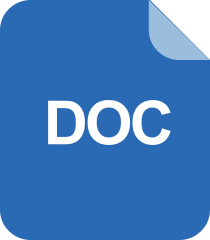
C语言 求一个二叉树中位于先序序列中第k个位置的结点的值.doc
以下是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉排序树的结构体
typedef struct BSTNode {
int data;
struct BSTNode* left;
struct BSTNode* right;
} BSTNode;
// 创建新节点并返回
BSTNode* createNode(int data) {
BSTNode* newNode = (BSTNode*)malloc(sizeof(BSTNode));
newNode->data = data;
newNode->left = NULL;
newNode->right = NULL;
return newNode;
}
// 将新节点插入到二叉排序树中
BSTNode* insertNode(BSTNode* root, int data) {
// 如果根节点为空,直接插入
if (root == NULL) {
return createNode(data);
}
// 如果插入的值小于根节点的值,则插入到左子树中
if (data < root->data) {
root->left = insertNode(root->left, data);
}
// 如果插入的值大于等于根节点的值,则插入到右子树中
else {
root->right = insertNode(root->right, data);
}
return root;
}
// 在二叉排序树中查找key是否存在
BSTNode* searchNode(BSTNode* root, int key) {
// 如果根节点为空,或者根节点的值等于key,则返回根节点
if (root == NULL || root->data == key) {
return root;
}
// 如果key小于根节点的值,则在左子树中查找
if (key < root->data) {
return searchNode(root->left, key);
}
// 如果key大于根节点的值,则在右子树中查找
return searchNode(root->right, key);
}
int main() {
BSTNode* root = NULL;
int n, key;
printf("请输入要插入的节点个数:");
scanf("%d", &n);
printf("请输入%d个节点的值,用空格分隔:", n);
for (int i = 0; i < n; i++) {
int data;
scanf("%d", &data);
root = insertNode(root, data);
}
printf("请输入要查找的值:");
scanf("%d", &key);
BSTNode* result = searchNode(root, key);
if (result == NULL) {
printf("%d不存在于二叉排序树中\n", key);
}
else {
printf("%d存在于二叉排序树中\n", key);
}
return 0;
}
```
输入样例:
```
请输入要插入的节点个数:6
请输入6个节点的值,用空格分隔:5 3 7 2 4 6
请输入要查找的值:4
```
输出样例:
```
4存在于二叉排序树中
```
阅读全文
相关推荐
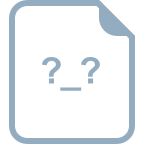












