C语言通讯录系统以文件的方式保存通讯录信息
时间: 2024-01-18 22:05:06 浏览: 144
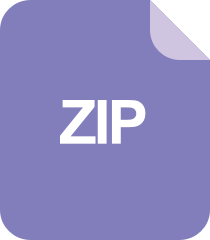
基于C语言的通讯录管理系统设计源码
可以使用文件来保存通讯录信息,这样可以实现信息的持久化存储。具体实现方法如下:
1. 定义联系人结构体
```c
typedef struct {
char name[20];
char phone[12];
char email[30];
} Contact;
```
2. 定义文件名和文件打开方式
```c
#define FILENAME "contacts.txt"
#define MODE "r+b"
```
3. 实现添加联系人函数
```c
void add_contact() {
Contact c;
FILE* fp = fopen(FILENAME, MODE);
if (fp == NULL) {
printf("Failed to open file\n");
return;
}
printf("Enter name: ");
scanf("%s", c.name);
printf("Enter phone number: ");
scanf("%s", c.phone);
printf("Enter email: ");
scanf("%s", c.email);
fwrite(&c, sizeof(Contact), 1, fp);
printf("Contact added successfully.\n");
fclose(fp);
}
```
4. 实现查找联系人函数
```c
void find_contact() {
char name[20];
Contact c;
FILE* fp = fopen(FILENAME, MODE);
if (fp == NULL) {
printf("Failed to open file\n");
return;
}
printf("Enter name to search: ");
scanf("%s", name);
while (fread(&c, sizeof(Contact), 1, fp) != 0) {
if (strcmp(c.name, name) == 0) {
printf("Name: %s\n", c.name);
printf("Phone: %s\n", c.phone);
printf("Email: %s\n", c.email);
fclose(fp);
return;
}
}
printf("Contact not found.\n");
fclose(fp);
}
```
5. 实现删除联系人函数
```c
void delete_contact() {
char name[20];
Contact c;
FILE* fp = fopen(FILENAME, MODE);
if (fp == NULL) {
printf("Failed to open file\n");
return;
}
printf("Enter name to delete: ");
scanf("%s", name);
FILE* tmp = tmpfile();
while (fread(&c, sizeof(Contact), 1, fp) != 0) {
if (strcmp(c.name, name) != 0) {
fwrite(&c, sizeof(Contact), 1, tmp);
}
}
fclose(fp);
fp = fopen(FILENAME, "wb");
rewind(tmp);
while (fread(&c, sizeof(Contact), 1, tmp) != 0) {
fwrite(&c, sizeof(Contact), 1, fp);
}
fclose(fp);
printf("Contact deleted successfully.\n");
}
```
6. 实现修改联系人函数
```c
void update_contact() {
char name[20];
Contact c;
FILE* fp = fopen(FILENAME, MODE);
if (fp == NULL) {
printf("Failed to open file\n");
return;
}
printf("Enter name to update: ");
scanf("%s", name);
FILE* tmp = tmpfile();
while (fread(&c, sizeof(Contact), 1, fp) != 0) {
if (strcmp(c.name, name) == 0) {
printf("Enter new name: ");
scanf("%s", c.name);
printf("Enter new phone number: ");
scanf("%s", c.phone);
printf("Enter new email: ");
scanf("%s", c.email);
}
fwrite(&c, sizeof(Contact), 1, tmp);
}
fclose(fp);
fp = fopen(FILENAME, "wb");
rewind(tmp);
while (fread(&c, sizeof(Contact), 1, tmp) != 0) {
fwrite(&c, sizeof(Contact), 1, fp);
}
fclose(fp);
printf("Contact updated successfully.\n");
}
```
7. 实现显示所有联系人函数
```c
void show_all_contacts() {
Contact c;
FILE* fp = fopen(FILENAME, MODE);
if (fp == NULL) {
printf("Failed to open file\n");
return;
}
while (fread(&c, sizeof(Contact), 1, fp) != 0) {
printf("Name: %s\n", c.name);
printf("Phone: %s\n", c.phone);
printf("Email: %s\n", c.email);
printf("\n");
}
fclose(fp);
}
```
注意事项:
1. 文件打开方式为 "r+b",表示以读写方式打开二进制文件,如果文件不存在则创建。
2. 查找、删除和修改联系人时需要使用临时文件进行操作,最后再将临时文件内容复制回原文件。
3. 代码中没有对输入的数据进行校验和验证,需要自行添加。
阅读全文
相关推荐
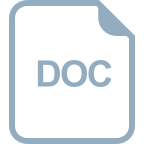
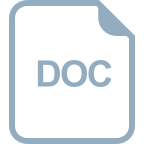
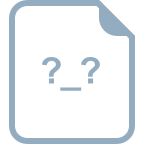
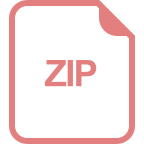
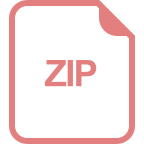
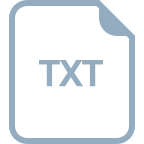
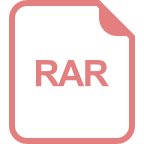
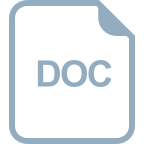
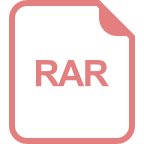
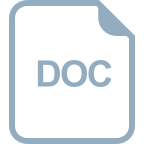

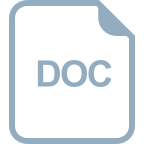