c# html写一个简单的评论页面,可以实现评论的发表显示和删除,给出完整代码并进行详细解释
时间: 2024-03-03 17:53:05 浏览: 21
以下是一个简单的C# HTML评论页面的完整代码,并附有详细解释:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace CommentPage
{
public partial class Comment : System.Web.UI.Page
{
// 创建一个Dictionary对象,用于存储评论
Dictionary<int, string> comments = new Dictionary<int, string>();
protected void Page_Load(object sender, EventArgs e)
{
// 在页面加载时,检查是否有保存的评论
if (Session["Comments"] != null)
{
comments = (Dictionary<int, string>)Session["Comments"];
}
else
{
// 如果没有保存的评论,则创建一个新的Dictionary对象
comments = new Dictionary<int, string>();
}
// 显示所有评论
ShowComments();
}
protected void btnSubmit_Click(object sender, EventArgs e)
{
// 获取用户输入的评论内容
string comment = txtComment.Text.Trim();
// 如果评论内容不为空,则将其添加到Dictionary对象中
if (!string.IsNullOrEmpty(comment))
{
// 获取当前评论的ID
int commentId = comments.Count + 1;
// 添加评论到Dictionary对象中
comments.Add(commentId, comment);
// 保存评论到Session中
Session["Comments"] = comments;
// 显示所有评论
ShowComments();
// 清空评论输入框
txtComment.Text = "";
}
}
protected void btnDelete_Click(object sender, EventArgs e)
{
// 获取用户输入的评论ID
int commentId = int.Parse(txtCommentId.Text.Trim());
// 如果评论ID存在于Dictionary对象中,则将其删除
if (comments.ContainsKey(commentId))
{
comments.Remove(commentId);
// 保存评论到Session中
Session["Comments"] = comments;
// 显示所有评论
ShowComments();
// 清空评论ID输入框
txtCommentId.Text = "";
}
else
{
// 如果评论ID不存在于Dictionary对象中,则提示用户
lblMessage.Text = "该评论ID不存在,请重新输入。";
}
}
private void ShowComments()
{
// 清空评论显示区域
lblComments.Text = "";
// 如果Dictionary对象中有评论,则将其显示出来
if (comments.Count > 0)
{
foreach (KeyValuePair<int, string> comment in comments)
{
lblComments.Text += "<div class='comment'>";
lblComments.Text += "<span class='comment-id'>" + comment.Key + "</span>";
lblComments.Text += "<span class='comment-text'>" + comment.Value + "</span>";
lblComments.Text += "</div>";
}
}
else
{
// 如果Dictionary对象中没有评论,则提示用户
lblComments.Text = "暂无评论。";
}
}
}
}
```
在这个页面中,我们创建了一个`Comment`类,继承自`System.Web.UI.Page`类。在页面加载时,我们检查是否有保存的评论,并显示所有评论。当用户点击“提交”按钮时,我们获取用户输入的评论内容,并将其添加到`Dictionary`对象中。当用户点击“删除”按钮时,我们获取用户输入的评论ID,并从`Dictionary`对象中删除该评论。最后,我们使用`ShowComments`方法来显示所有评论。
在HTML部分,我们创建了一个评论输入框,一个“提交”按钮和一个“删除”按钮。我们还创建了一个评论显示区域,用于显示所有评论。当用户输入评论ID并点击“删除”按钮时,我们还创建了一个评论ID输入框和一个消息标签,用于向用户显示错误消息。
```html
<!DOCTYPE html>
<html>
<head>
<title>评论页面</title>
<style>
.comment {
margin-bottom: 10px;
padding: 10px;
border: 1px solid #ccc;
background-color: #f5f5f5;
}
.comment-id {
display: inline-block;
font-weight: bold;
margin-right: 10px;
}
.comment-text {
display: inline-block;
}
</style>
</head>
<body>
<h1>评论页面</h1>
<h2>发表评论</h2>
<div>
<textarea id="txtComment" name="txtComment" rows="5" cols="50"></textarea>
</div>
<div>
<button id="btnSubmit" name="btnSubmit" onclick="javascript:__doPostBack('btnSubmit','')">提交</button>
</div>
<h2>删除评论</h2>
<div>
<label for="txtCommentId">评论ID:</label>
<input type="text" id="txtCommentId" name="txtCommentId" />
</div>
<div>
<button id="btnDelete" name="btnDelete" onclick="javascript:__doPostBack('btnDelete','')">删除</button>
</div>
<div>
<span id="lblMessage" name="lblMessage"></span>
</div>
<h2>评论列表</h2>
<div id="lblComments" name="lblComments"></div>
</body>
</html>
```
在CSS部分,我们为评论和评论ID创建了样式。我们使用`margin-bottom`和`padding`属性来添加间距和内边距。我们使用`border`属性来添加边框,并使用`background-color`属性来设置背景颜色。我们使用`display`属性来将评论ID和评论文本显示在同一行,并使用`font-weight`属性来加粗评论ID。
这是一个非常基本的评论页面,只包含了最基本的功能。您可以根据需要对其进行扩展,例如添加用户验证、显示评论时间戳等等。
相关推荐
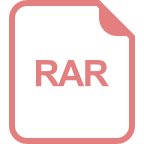
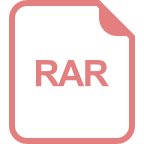
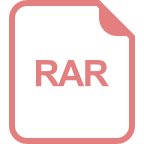














