react - beautiful-dnd 写一个列表拖拽
时间: 2024-04-29 14:26:24 浏览: 167
React-beautiful-dnd是一个React组件库,用于实现漂亮且易于使用的拖放列表。要实现一个列表拖放,需要执行以下步骤:
1. 安装react-beautiful-dnd:
```
npm install --save react-beautiful-dnd
```
2. 导入DragDropContext、Droppable和Draggable组件:
```javascript
import { DragDropContext, Droppable, Draggable } from 'react-beautiful-dnd';
```
3. 创建状态并为每个列表项目分配一个唯一标识符:
```javascript
const [items, setItems] = useState([
{ id: "1", content: "Item 1" },
{ id: "2", content: "Item 2" },
{ id: "3", content: "Item 3" },
{ id: "4", content: "Item 4" },
{ id: "5", content: "Item 5" },
{ id: "6", content: "Item 6" }
]);
```
4. 创建渲染函数以呈现每个拖动项目:
```javascript
const renderItems = () => {
return items.map((item, index) => (
<Draggable key={item.id} draggableId={item.id} index={index}>
{(provided, snapshot) => (
<div ref={provided.innerRef} {...provided.draggableProps} {...provided.dragHandleProps} style={getItemStyle(snapshot.isDragging, provided.draggableProps.style)}>
{item.content}
</div>
)}
</Draggable>
));
};
```
5. 创建渲染函数以呈现拖放列表:
```javascript
const renderList = () => {
return (
<DragDropContext onDragEnd={onDragEnd}>
<Droppable droppableId="items">
{(provided, snapshot) => (
<div {...provided.droppableProps} ref={provided.innerRef} style={getListStyle(snapshot.isDraggingOver)}>
{renderItems()}
{provided.placeholder}
</div>
)}
</Droppable>
</DragDropContext>
);
};
```
6. 创建onDragEnd函数以处理拖动项目:
```javascript
const onDragEnd = result => {
if (!result.destination) {
return;
}
const itemsCopy = [...items];
const [reorderedItem] = itemsCopy.splice(result.source.index, 1);
itemsCopy.splice(result.destination.index, 0, reorderedItem);
setItems(itemsCopy);
};
```
7. 创建样式函数以获取拖动和放置元素的样式:
```javascript
const getItemStyle = (isDragging, draggableStyle) => ({
userSelect: 'none',
padding: 16,
margin: `0 0 ${8}px 0`,
border: isDragging ? '2px solid #000' : 'none',
...draggableStyle
});
const getListStyle = isDraggingOver => ({
background: isDraggingOver ? 'lightblue' : '#eee',
padding: 8,
width: 250
});
```
最后,用renderList函数渲染列表,将其放在你的React组件中,就可以开始拖放操作了!
阅读全文
相关推荐
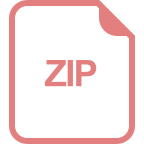

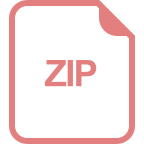

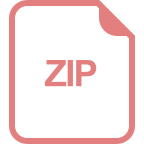
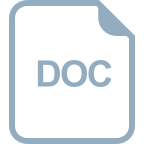



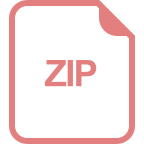
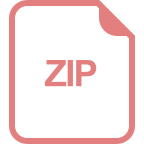
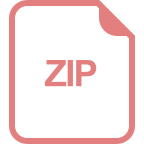
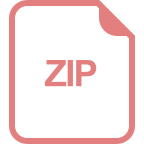

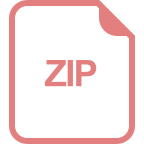
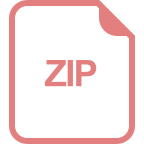
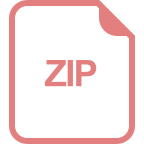