用Java语言写一个LRU算法的程序
时间: 2023-06-08 09:08:30 浏览: 55
好的,以下是Java语言实现LRU算法的代码:
```
import java.util.HashMap;
import java.util.Map;
public class LRUCache<K, V> {
private final int CACHE_SIZE;
private final Map<K, Node<K, V>> cacheMap;
private Node<K, V> head;
private Node<K, V> tail;
public LRUCache(int cacheSize) {
CACHE_SIZE = cacheSize;
cacheMap = new HashMap<>();
}
public void put(K key, V value) {
Node<K, V> node = cacheMap.get(key);
if (node == null) {
node = new Node<>(key, value);
cacheMap.put(key, node);
addNode(node);
if (cacheMap.size() > CACHE_SIZE) {
removeTail();
}
} else {
node.value = value;
moveNodeToHead(node);
}
}
public V get(K key) {
Node<K, V> node = cacheMap.get(key);
if (node == null) {
return null;
}
moveNodeToHead(node);
return node.value;
}
private void addNode(Node<K, V> node) {
if (tail == null) {
head = tail = node;
} else {
node.next = head;
head.prev = node;
head = node;
}
}
private void removeNode(Node<K, V> node) {
if (node == head) {
head = node.next;
}
if (node == tail) {
tail = node.prev;
}
if (node.prev != null) {
node.prev.next = node.next;
}
if (node.next != null) {
node.next.prev = node.prev;
}
}
private void moveNodeToHead(Node<K, V> node) {
removeNode(node);
addNode(node);
}
private void removeTail() {
cacheMap.remove(tail.key);
removeNode(tail);
}
private static class Node<K, V> {
private final K key;
private V value;
private Node<K, V> prev;
private Node<K, V> next;
public Node(K key, V value) {
this.key = key;
this.value = value;
}
}
}
```
LRU(Least Recently Used)算法是一种常用的缓存替换算法,它根据数据的历史访问记录来决定要淘汰哪些数据。基本思想是:优先淘汰最长时间未被访问的数据项。以上代码实现了LRU算法的缓存数据结构,可以通过put方法新增数据项,通过get方法获取数据项,缓存的最大容量可以通过构造函数传入。
相关推荐
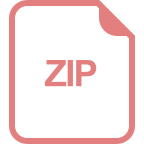














