只将摄像头采集到的图片作为yolov5输入的图片
时间: 2023-06-18 09:02:49 浏览: 124
如果你想将摄像头采集到的图片作为 YOLOv5 的输入图片,你可以使用 OpenCV 库中的 VideoCapture 函数从摄像头采集视频帧,并将每个视频帧作为 YOLOv5 的输入图片。以下是一个基本的示例代码:
```python
import cv2
import torch
from models.experimental import attempt_load
from utils.general import non_max_suppression, scale_coords
from utils.plots import colors, plot_one_box
# 加载 YOLOv5 模型
model = attempt_load('yolov5s.pt', map_location=torch.device('cpu'))
# 打开摄像头
cap = cv2.VideoCapture(0)
while True:
# 从摄像头采集视频帧
ret, frame = cap.read()
# 将视频帧转换为 YOLOv5 所需的格式
img = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
img = cv2.resize(img, (640, 640))
img = torch.from_numpy(img.transpose(2, 0, 1)).float().div(255.0).unsqueeze(0)
# 对图片进行目标检测
pred = model(img)[0]
pred = non_max_suppression(pred, conf_thres=0.5, iou_thres=0.5)
# 在图片上绘制检测框
for det in pred:
if det is not None and len(det):
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], frame.shape).round()
for *xyxy, conf, cls in reversed(det):
c = int(cls)
label = f'{model.names[c]} {conf:.2f}'
plot_one_box(xyxy, frame, label=label, color=colors(c, True), line_thickness=2)
# 显示检测结果
cv2.imshow('YOLOv5', frame)
# 按下 q 键退出程序
if cv2.waitKey(1) == ord('q'):
break
# 关闭摄像头和窗口
cap.release()
cv2.destroyAllWindows()
```
在这个示例中,我们使用 OpenCV 库中的 VideoCapture 函数从摄像头采集视频帧,并将每个视频帧转换为 YOLOv5 所需的格式。然后,我们使用 YOLOv5 模型对图片进行目标检测,并将检测结果绘制在图片上。最后,我们使用 OpenCV 库中的 imshow 函数将图片显示在窗口中,并使用 waitKey 函数等待用户按下 q 键退出程序。
阅读全文
相关推荐
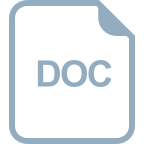
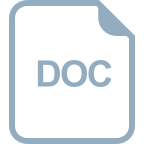
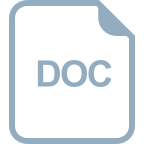


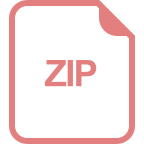
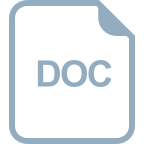
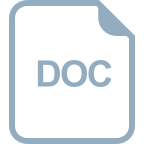
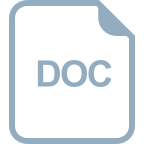
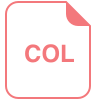
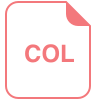
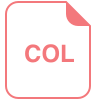
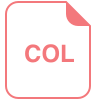
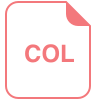
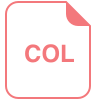



