用c++写一个银行员工权限管理系统
时间: 2023-08-04 07:10:26 浏览: 154
好的,以下是一个简单的银行员工权限管理系统的C++代码,实现了登录、权限管理和员工信息查询等功能。
```cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 定义员工类
class Employee {
public:
Employee(int id, string name, string password, int authority) :
m_id(id), m_name(name), m_password(password), m_authority(authority) {}
int getId() const {
return m_id;
}
string getName() const {
return m_name;
}
string getPassword() const {
return m_password;
}
int getAuthority() const {
return m_authority;
}
private:
int m_id; // 员工ID
string m_name; // 员工姓名
string m_password; // 登录密码
int m_authority; // 权限等级
};
// 定义员工列表
vector<Employee> employeeList = {
Employee(1001, "张三", "123456", 1),
Employee(1002, "李四", "123456", 2),
Employee(1003, "王五", "123456", 3)
};
// 登录函数,返回登录成功的员工ID,失败返回-1
int login() {
cout << "请输入员工ID:";
int id;
cin >> id;
cout << "请输入密码:";
string password;
cin >> password;
for (auto employee : employeeList) {
if (employee.getId() == id && employee.getPassword() == password) {
cout << "登录成功!" << endl;
return id;
}
}
cout << "登录失败!" << endl;
return -1;
}
// 查询员工信息函数,需要传入登录成功的员工ID
void queryEmployeeInfo(int employeeId) {
int authority = 0;
for (auto employee : employeeList) {
if (employee.getId() == employeeId) {
authority = employee.getAuthority();
cout << "员工ID:" << employee.getId() << endl;
cout << "员工姓名:" << employee.getName() << endl;
break;
}
}
if (authority >= 2) {
cout << "员工列表:" << endl;
for (auto employee : employeeList) {
cout << "员工ID:" << employee.getId() << ",员工姓名:" << employee.getName() << endl;
}
}
if (authority >= 3) {
cout << "管理员权限:可以修改员工信息!" << endl;
}
}
int main() {
int employeeId = login();
if (employeeId != -1) {
queryEmployeeInfo(employeeId);
}
return 0;
}
```
该系统的实现思路是先定义一个员工类,包含员工ID、姓名、密码和权限等级等属性。然后定义一个员工列表,存储所有的员工信息。接着实现登录函数,让用户输入员工ID和密码,然后遍历员工列表,匹配对应的员工信息,如果匹配成功,则登录成功,返回员工ID。登录成功后,调用查询员工信息函数,根据员工ID查询对应的员工信息,并根据员工的权限等级决定是否可以查看员工列表和修改员工信息。
阅读全文
相关推荐


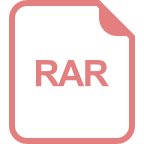
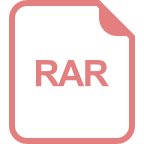
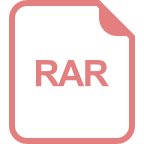
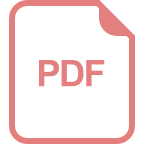
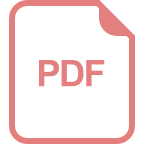
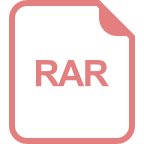
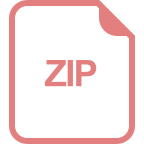
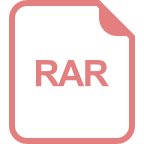
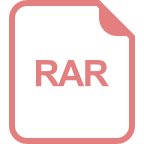
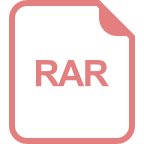
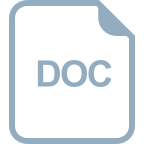
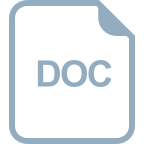
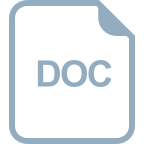
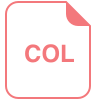