mac_aton()函数用法
时间: 2023-11-30 19:02:44 浏览: 47
mac_aton()函数是一个将MAC地址字符串转换为二进制格式的函数,其用法如下:
```
#include <stdlib.h>
#include <string.h>
#include <netinet/ether.h>
int main() {
char *mac_str = "00:11:22:33:44:55";
struct ether_addr *mac = ether_aton(mac_str);
if (mac == NULL) {
printf("Invalid MAC address\n");
exit(1);
}
printf("MAC address in binary format: ");
for (int i = 0; i < 6; i++) {
printf("%02x ", mac->ether_addr_octet[i]);
}
printf("\n");
return 0;
}
```
该函数需要传入一个MAC地址字符串作为参数,返回一个指向ether_addr结构体的指针。如果MAC地址字符串无效,则返回NULL。可以使用该函数将MAC地址字符串转换为二进制格式,并以十六进制形式打印出来。
相关问题
inet_aton函数实现
inet_aton函数是一个网络编程中常用的函数,其作用是将一个IP地址的字符串表示形式转换为网络字节序的二进制表示形式。
在网络编程中,IP地址通常用点分十进制的形式表示,例如"192.168.0.1"。然而,在计算机内部进行网络通信时,IP地址需要以二进制的形式表示,即网络字节序。
inet_aton函数的具体实现思路如下:
1. 首先,函数会检查传入的IP地址字符串是否合法,不合法的IP地址将返回0.
2. 如果IP地址合法,则函数会创建一个in_addr结构体来存储转换后的二进制形式的IP地址。
3. 函数会根据IP地址的字符串表示形式,从高位开始,逐段将其转换为二进制形式,并存储在in_addr结构体的s_addr字段中。在转换时,函数会将每一段IP地址解析为一个32位无符号整数,并根据网络字节序进行存储。
4. 最后,函数会返回转换后的in_addr结构体中的s_addr字段,即表示IP地址的二进制形式。
通过使用inet_aton函数,我们可以方便地将IP地址的字符串表示转换为网络字节序的二进制形式,从而方便进行网络通信和IP地址的相关操作。
inet_aton函数如何编写
inet_aton函数是一个用于将IPv4地址字符串转换为二进制形式的函数,它的主要功能是将形如 "192.168.1.1" 的字符串形式的IPv4地址转换成32位的整数,便于网络编程中的一些操作。
要编写inet_aton函数,可以按照以下步骤进行:
1. 首先,需要解析IPv4地址字符串,判断其格式是否正确。IPv4地址由四个十进制数构成,每个数的范围是0-255,并且用点分隔。
2. 然后,将每个十进制数转换为二进制形式。可以使用 standard library 中提供的 atoi 函数,将每个十进制数转换为对应的整数。
3. 接下来,将转换后的四个整数组合起来,形成一个32位的整数。可以使用位运算操作,将每个整数按位左移并累加。
4. 最后,将得到的32位整数作为函数的返回值。
下面是一个示例代码,展示了如何实现inet_aton函数:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
unsigned int inet_aton(const char* addr) {
unsigned int ip = 0;
int nums[4];
if (sscanf(addr, "%d.%d.%d.%d", &nums[0], &nums[1], &nums[2], &nums[3]) != 4) {
printf("Invalid IP address format\n");
return 0;
}
for (int i = 0; i < 4; i++) {
if (nums[i] < 0 || nums[i] > 255) {
printf("Invalid IP address range\n");
return 0;
}
ip = (ip << 8) + nums[i];
}
return ip;
}
int main() {
const char* ip_addr = "192.168.1.1";
unsigned int ip = inet_aton(ip_addr);
printf("IP address in binary format: %u\n", ip);
return 0;
}
```
通过这段代码,我们可以将IPv4地址字符串 "192.168.1.1" 转换为二进制形式的整数,并且输出转换后的结果为 3232235777。这样,我们就成功地实现了inet_aton函数。
相关推荐
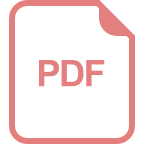
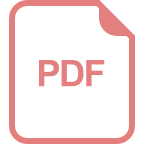
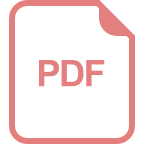












