利用lambda表达式的例子
时间: 2023-05-29 17:05:53 浏览: 102
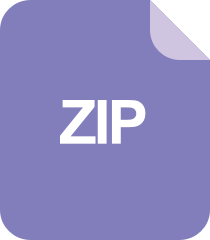
Lambda表达式的例子
1. 求和函数
```python
sum = lambda x, y: x + y
print(sum(2, 3)) # Output: 5
```
2. 列表排序
```python
students = [('Tom', 20), ('John', 15), ('Alex', 25)]
students.sort(key=lambda x: x[1])
print(students) # Output: [('John', 15), ('Tom', 20), ('Alex', 25)]
```
3. 过滤列表
```python
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers) # Output: [2, 4, 6, 8, 10]
```
4. 列表映射
```python
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
squared_numbers = list(map(lambda x: x**2, numbers))
print(squared_numbers) # Output: [1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
```
5. 匿名函数作为参数
```python
def my_function(numbers, func):
result = []
for number in numbers:
result.append(func(number))
return result
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
squared_numbers = my_function(numbers, lambda x: x**2)
print(squared_numbers) # Output: [1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
```
阅读全文
相关推荐

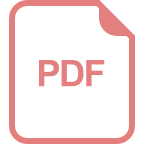
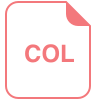
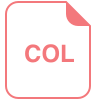
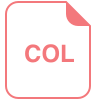


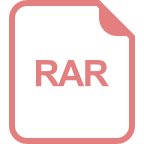
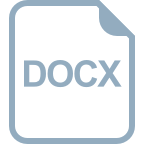
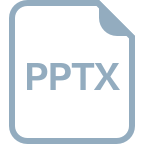
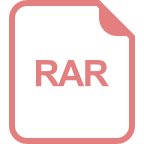
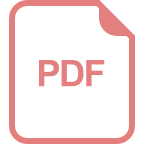
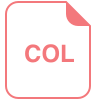
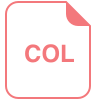

