如何使用Python编写一个具备图片爬取功能的高级网络爬虫程序?
时间: 2024-12-19 10:22:46 浏览: 8
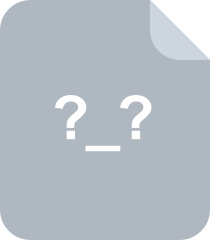
使用python编写·爬虫程序,主要用于爬取图片
在Python中编写一个具备图片爬取功能的高级网络爬虫,通常会使用一些库如BeautifulSoup、requests和Scrapy等。以下是一个简化的步骤:
1. **安装所需库**:
首先需要安装`requests`库用于发送HTTP请求获取网页内容,`beautifulsoup4`用于解析HTML,以及`scrapy`如果要用到更强大的爬虫框架。
```bash
pip install requests beautifulsoup4 scrapy
```
2. **基本爬虫结构**:
使用`requests.get()`获取目标网站的HTML,然后用BeautifulSoup解析这个HTML文档。
```python
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
```
3. **找到图片元素**:
在BeautifulSoup中,你可以通过CSS选择器或XPath表达式来定位图片元素,例如所有`img`标签。
```python
img_tags = soup.select('img')
```
4. **下载图片**:
对于每个图片元素,提取出URL,然后使用`requests`下载图片并保存到本地。
```python
import os
import urllib.request
image_urls = [img['src'] for img in img_tags]
for url in image_urls:
response = requests.get(url, stream=True)
filename = os.path.join(os.getcwd(), os.path.basename(url))
with open(filename, 'wb') as f:
for chunk in response.iter_content(chunk_size=1024):
if chunk: # filter out keep-alive new chunks
f.write(chunk)
```
5. **高级爬虫**:
如果要构建更复杂的爬虫,可以考虑使用Scrapy框架,它提供了一套完整的API和中间件系统,处理登录、反爬虫策略等问题更为方便。
```python
# 使用Scrapy
import scrapy
class ImageSpider(scrapy.Spider):
name = 'imagespider'
start_urls = ['https://example.com']
def parse(self, response):
img_links = response.css('img::attr(src)').getall()
for link in img_links:
yield {'image_url': link}
# 下一页链接
next_page = response.css('a.next::attr(href)').get()
if next_page is not None:
yield response.follow(next_page, self.parse)
```
阅读全文
相关推荐
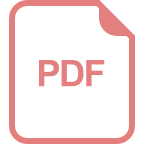
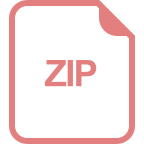
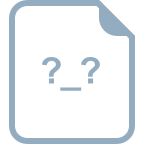
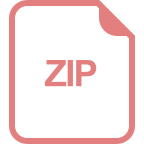
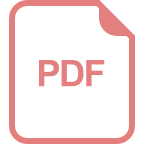
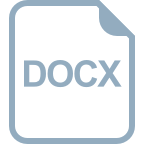
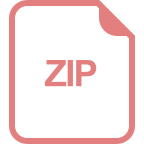
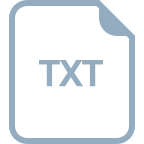
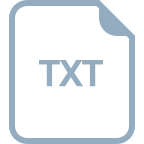
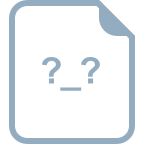
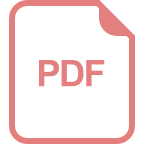
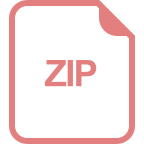
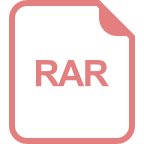
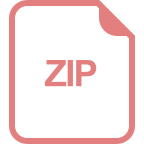
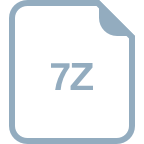
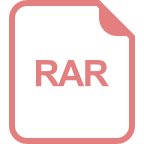
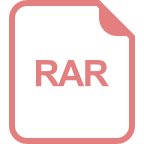