写个实例并且保留html样式
时间: 2023-09-04 09:10:18 浏览: 50
当使用Spring Boot将HTML文件转换为docx时,保留HTML样式会有一些挑战。因为HTML和docx的样式系统不完全相同,所以无法直接将HTML样式转换为docx样式。但是,我们可以尝试将HTML中的一些常见样式转换为docx中的样式。
以下是一个示例,演示如何使用Apache POI将HTML文件转换为docx,并尝试保留一些常见的HTML样式:
```java
import org.apache.poi.xwpf.usermodel.*;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.springframework.stereotype.Controller;
import org.springframework.util.ResourceUtils;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.ResponseBody;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
@Controller
public class HtmlToDocxController {
@PostMapping("/convert")
@ResponseBody
public String convertHtmlToDocx(@RequestBody String html) {
try {
// 创建docx文档对象
XWPFDocument document = new XWPFDocument();
// 解析HTML内容
Document doc = Jsoup.parse(html);
// 遍历解析后的HTML元素
Elements elements = doc.body().children();
for (Element element : elements) {
parseElement(element, document);
}
// 保存为docx文件
File file = ResourceUtils.getFile("classpath:result.docx");
FileOutputStream out = new FileOutputStream(file);
document.write(out);
out.close();
return "转换成功!";
} catch (IOException e) {
e.printStackTrace();
return "转换失败!";
}
}
private void parseElement(Element element, XWPFDocument document) {
// 创建段落对象
XWPFParagraph paragraph = document.createParagraph();
// 根据元素标签设置段落样式
if ("h1".equalsIgnoreCase(element.tagName())) {
paragraph.setStyle("Heading1");
} else if ("h2".equalsIgnoreCase(element.tagName())) {
paragraph.setStyle("Heading2");
} else if ("h3".equalsIgnoreCase(element.tagName())) {
paragraph.setStyle("Heading3");
} else if ("p".equalsIgnoreCase(element.tagName())) {
paragraph.setStyle("Normal");
}
// 解析元素的子元素
Elements children = element.children();
for (Element child : children) {
parseElement(child, paragraph);
}
}
private void parseElement(Element element, XWPFParagraph paragraph) {
// 创建文本对象
XWPFRun run = paragraph.createRun();
// 设置文本内容
run.setText(element.text());
// 设置文本样式
if ("b".equalsIgnoreCase(element.tagName())) {
run.setBold(true);
} else if ("i".equalsIgnoreCase(element.tagName())) {
run.setItalic(true);
} else if ("u".equalsIgnoreCase(element.tagName())) {
run.setUnderline(UnderlinePatterns.SINGLE);
} else if ("strike".equalsIgnoreCase(element.tagName())) {
run.setStrike(true);
}
}
}
```
在这个示例中,我们使用了jsoup库来解析HTML内容,并使用Apache POI来创建docx文档。在遍历解析后的HTML元素时,我们尝试根据元素的标签和属性设置docx段落和文本的样式。请注意,这只是一个简单的示例,无法涵盖所有HTML样式。你可能需要根据实际需求进行更多的样式转换处理。
请确保在Spring Boot应用中运行该Controller,并使用POST请求将HTML内容作为请求体发送到`/convert`路径。转换后的docx文件将保存在项目的`classpath`目录下的`result.docx`文件中。
鉴于HTML和docx的样式系统的差异,无法保证完全准确地保留HTML样式。在实际使用中,可能需要根据具体需求进行更复杂的样式转换和处理。
相关推荐
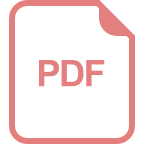
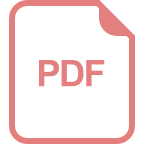










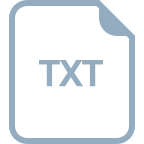
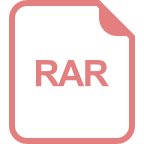
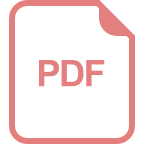
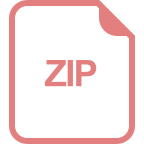