如何在输入数组数据后声明数组的量
时间: 2024-10-11 13:07:47 浏览: 17
在大多数编程语言中,当你想要声明一个数组并指定其长度或大小时,通常需要提供数组的元素类型和长度。这里以几种常见语言为例:
1. **Python**:
```python
# 声明整数数组,包含5个元素
array = [0] * 5
# 或者更明确地指定元素类型
numbers = list(range(5))
```
2. **JavaScript**:
```javascript
let array = new Array(5); // 创建一个空的长度为5的数组
// 或者
let numbers = [0, 1, 2, 3, 4];
```
3. **C/C++**:
```c++
int array[5]; // 声明一个包含5个int类型的元素数组
```
4. **Java**:
```java
int[] numbers = new int[5]; // 创建一个包含5个int元素的数组
```
5. **C#**:
```csharp
int[] array = new int[5]; // 创建一个长度为5的整数数组
```
在声明数组时,你需要先确定数组将存储的数据类型,并根据需要提供的初始值或预设的长度来创建。
相关问题
c语言中定义一维数组,输入数组
在 C 语言中,定义一维数组并输入数组可以按照以下步骤进行:
1. 定义数组:在程序中声明数组变量、指定数组元素的数据类型、数组的名称和数组元素的个数。例如,定义一个包含 5 个整数的数组可以写成:
```
int arr[5];
```
2. 输入数组元素:使用循环结构输入数组中的元素。例如,使用 for 循环输入上述定义的数组中的元素可以写成:
```
for (int i = 0; i < 5; i++) {
scanf("%d", &arr[i]);
}
```
这样,就可以定义一个包含 5 个整数的数组并输入数组元素了。
怎么把一堆数字输入到数组里面
您好!要把一堆数字输入到数组里面,可以按照以下步骤操作:
1. 声明一个数组,例如 int arr[10]; 就可以声明一个长度为10的整型数组。
2. 通过循环语句或者输入函数,将数据逐一输入到数组中。例如:
```
for(int i=0; i<10; i++){
cin >> arr[i]; // 通过输入流将数据输入到数组中
}
```
或者
```
int i=0;
while(i<10){
cin >> arr[i]; // 通过输入流将数据输入到数组中
i++;
}
```
这样就可以把一堆数字输入到数组里面了。
阅读全文
相关推荐
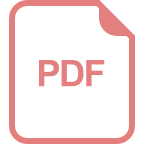
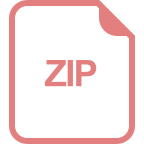
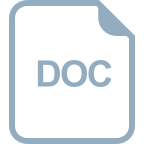
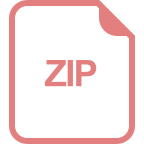
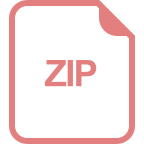
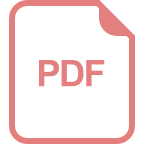
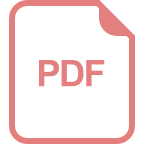
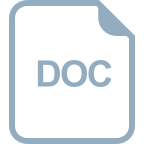
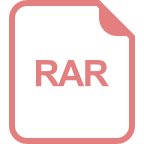
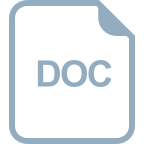
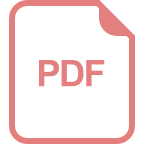
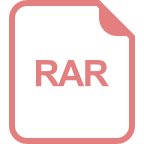
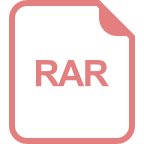
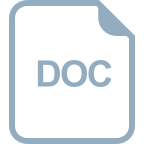
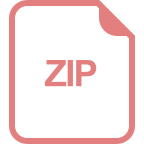
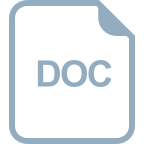
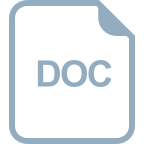