首页uniapp中断请求
uniapp中断请求
时间: 2023-10-03 20:10:57 浏览: 188
在uniapp中,你可以使用拦截器来中断请求。在页面跳转成功后,可以添加一个拦截器来终止之前的请求并清空任务队列。你可以在App.vue文件中的onShow方法中添加以下代码:
uni.addInterceptor('navigateTo', {
success: () => {
this.$store.commit('request/clearList');
}
});
这样,在每次页面跳转成功后,之前的请求会被终止,并且任务队列会被清空。同时,在App.vue文件的onHide方法中,你可以移除拦截器,以确保不会影响其他页面的请求。
阅读全文
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
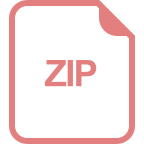
基于python与Django的网上购物平台
基于python与Django的网上购物平台,页面整洁美观,主要功能有:
1、首页包括我的订单、购物车、我的收藏、我的足迹
2、商品分类查找、商品搜索、待收货、待发货、代付款
3、商品详情信息、配送地址选择、加入购物车
4、系统的登录和注册
使用的是mysql数据库,适合初学者下载使用。
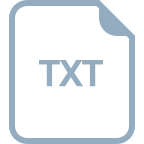
数据库设计管理课程设计系统设计报告(powerdesign+sql+DreamweaverCS)超市管理系统设计与开发2
数据库设计管理课程设计系统设计报告(powerdesign+sql+DreamweaverCS)超市管理系统设计与开发2提取方式是百度网盘分享地址
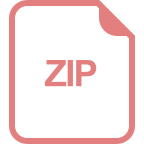
基于springboot的物流管理系统源码数据库文档.zip
基于springboot的物流管理系统源码数据库文档.zip
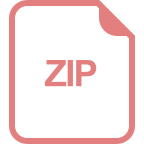
springboot285基于Java web的药店管理系统的设计与实现.zip
论文描述:该论文研究了某一特定领域的问题,并提出了新的解决方案。论文首先对问题进行了详细的分析和理解,并对已有的研究成果进行了综述。然后,论文提出了一种全新的解决方案,包括算法、模型或方法。在整个研究过程中,论文使用了合适的实验设计和数据集,并进行了充分的实验验证。最后,论文对解决方案的性能进行了全面的评估和分析,并提出了进一步的研究方向。
源码内容描述:该源码实现了论文中提出的新的解决方案。源码中包含了算法、模型或方法的具体实现代码,以及相关的数据预处理、实验设计和性能评估代码。源码中还包括了合适的注释和文档,以方便其他研究者理解和使用。源码的实现应该具有可读性、可维护性和高效性,并能够复现论文中的实验结果。此外,源码还应该尽可能具有通用性,以便在其他类似问题上进行进一步的应用和扩展。
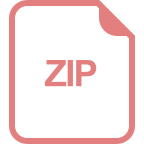
基于springboot云平台的信息安全攻防实训平台源码数据库文档.zip
基于springboot云平台的信息安全攻防实训平台源码数据库文档.zip
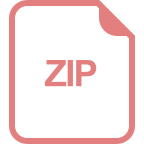
深入浅出:自定义 Grunt 任务的实践指南
资源摘要信息:"Grunt 是一个基于 Node.js 的自动化任务运行器,它极大地简化了重复性任务的管理。在前端开发中,Grunt 经常用于压缩文件、运行测试、编译 LESS/SASS、优化图片等。本文档提供了自定义 Grunt 任务的示例,对于希望深入掌握 Grunt 或者已经开始使用 Grunt 但需要扩展其功能的开发者来说,这些示例非常有帮助。"
### 知识点详细说明
#### 1. 创建和加载任务
在 Grunt 中,任务是由 JavaScript 对象表示的配置块,可以包含任务名称、操作和选项。每个任务可以通过 `grunt.registerTask(taskName, [description, ] fn)` 来注册。例如,一个简单的任务可以这样定义:
```javascript
grunt.registerTask('example', function() {
grunt.log.writeln('This is an example task.');
});
```
加载外部任务,可以通过 `grunt.loadNpmTasks('grunt-contrib-jshint')` 来实现,这通常用在安装了新的插件后。
#### 2. 访问 CLI 选项
Grunt 支持命令行接口(CLI)选项。在任务中,可以通过 `grunt.option('option')` 来访问命令行传递的选项。
```javascript
grunt.registerTask('printOptions', function() {
grunt.log.writeln('The watch option is ' + grunt.option('watch'));
});
```
#### 3. 访问和修改配置选项
Grunt 的配置存储在 `grunt.config` 对象中。可以通过 `grunt.config.get('configName')` 获取配置值,通过 `grunt.config.set('configName', value)` 设置配置值。
```javascript
grunt.registerTask('printConfig', function() {
grunt.log.writeln('The banner config is ' + grunt.config.get('banner'));
});
```
#### 4. 使用 Grunt 日志
Grunt 提供了一套日志系统,可以输出不同级别的信息。`grunt.log` 提供了 `writeln`、`write`、`ok`、`error`、`warn` 等方法。
```javascript
grunt.registerTask('logExample', function() {
grunt.log.writeln('This is a log example.');
grunt.log.ok('This is OK.');
});
```
#### 5. 使用目标
Grunt 的配置可以包含多个目标(targets),这样可以为不同的环境或文件设置不同的任务配置。在任务函数中,可以通过 `this.args` 获取当前目标的名称。
```javascript
grunt.initConfig({
jshint: {
options: {
curly: true,
},
files: ['Gruntfile.js'],
my_target: {
options: {
eqeqeq: true,
},
},
},
});
grunt.registerTask('showTarget', function() {
grunt.log.writeln('Current target is: ' + this.args[0]);
});
```
#### 6. 异步任务
Grunt 支持异步任务,这对于处理文件读写或网络请求等异步操作非常重要。异步任务可以通过传递一个回调函数给任务函数来实现。若任务是一个异步操作,必须调用回调函数以告知 Grunt 任务何时完成。
```javascript
grunt.registerTask('asyncTask', function() {
var done = this.async(); // 必须调用 this.async() 以允许异步任务。
setTimeout(function() {
grunt.log.writeln('This is an async task.');
done(); // 任务完成时调用 done()。
}, 1000);
});
```
### Grunt插件和Gruntfile配置
Grunt 的强大之处在于其插件生态系统。通过 `npm` 安装插件后,需要在 `Gruntfile.js` 中配置这些插件,才能在任务中使用它们。Gruntfile 通常包括任务注册、任务配置、加载外部任务三大部分。
- 任务注册:使用 `grunt.registerTask` 方法。
- 任务配置:使用 `grunt.initConfig` 方法。
- 加载外部任务:使用 `grunt.loadNpmTasks` 方法。
### 结论
通过上述的示例和说明,我们可以了解到创建一个自定义的 Grunt 任务需要哪些步骤以及需要掌握哪些基础概念。自定义任务的创建对于利用 Grunt 来自动化项目中的各种操作是非常重要的,它可以帮助开发者提高工作效率并保持代码的一致性和标准化。在掌握这些基础知识后,开发者可以更进一步地探索 Grunt 的高级特性,例如子任务、组合任务等,从而实现更加复杂和强大的自动化流程。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
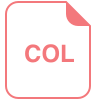
数据可视化在缺失数据识别中的作用
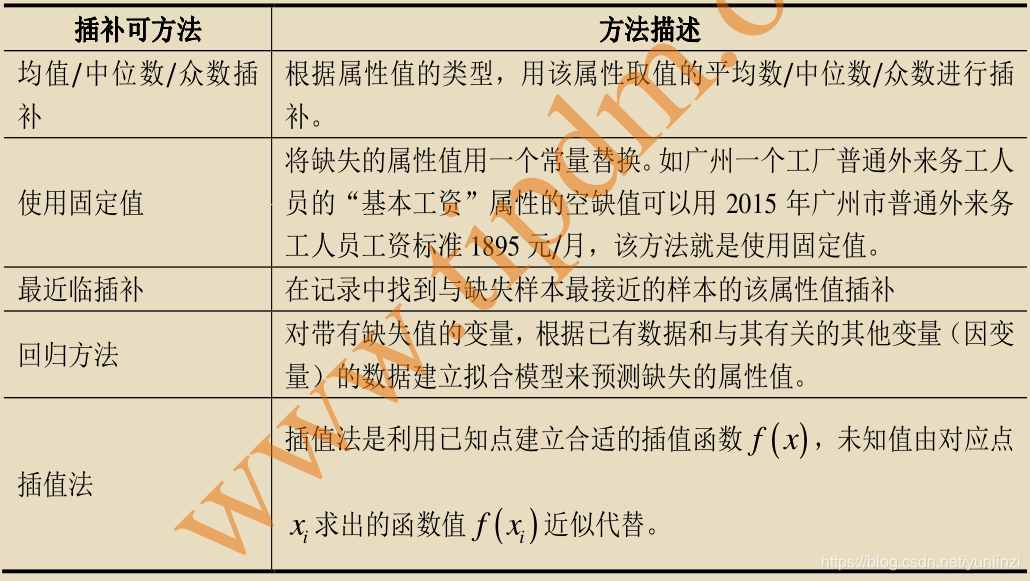
# 1. 数据可视化基础与重要性
在数据科学的世界里,数据可视化是将数据转化为图形和图表的实践过程,使得复杂的数据集可以通过直观的视觉形式来传达信息。它

ABB机器人在自动化生产线中是如何进行路径规划和任务执行的?请结合实际应用案例分析。
ABB机器人在自动化生产线中的应用广泛,其核心在于精确的路径规划和任务执行。路径规划是指机器人根据预定的目标位置和工作要求,计算出最优的移动轨迹。任务执行则涉及根据路径规划结果,控制机器人关节和运动部件精确地按照轨迹移动,完成诸如焊接、装配、搬运等任务。
参考资源链接:[ABB-机器人介绍.ppt](https://wenku.csdn.net/doc/7xfddv60ge?spm=1055.2569.3001.10343)
ABB机器人能够通过其先进的控制器和编程软件进行精确的路径规划。控制器通常使用专门的算法,如A*算法或者基于时间最优的轨迹规划技术,以确保机器人运动的平滑性和效率。此
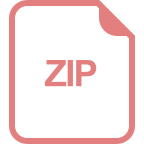
网络物理突变工具的多点路径规划实现与分析
资源摘要信息:"多点路径规划matlab代码-mutationdocker:变异码头工人"
### 知识点概述
#### 多点路径规划与网络物理突变工具
多点路径规划指的是在网络环境下,对多个路径点进行规划的算法或工具。该工具可能被应用于物流、运输、通信等领域,以优化路径和提升效率。网络物理系统(CPS,Cyber-Physical System)结合了计算机网络和物理过程,其中网络物理突变工具是指能够修改或影响网络物理系统中的软件代码的功能,特别是在自动驾驶、智能电网、工业自动化等应用中。
#### 变异与Mutator软件工具
变异(Mutation)在软件测试领域是指故意对程序代码进行小的改动,以此来检测程序测试用例的有效性。mutator软件工具是一种自动化的工具,它能够在编程文件上执行这些变异操作。在代码质量保证和测试覆盖率的评估中,变异分析是提高软件可靠性的有效方法。
#### Mutationdocker
Mutationdocker是一个配置为运行mutator的虚拟机环境。虚拟机环境允许用户在隔离的环境中运行软件,无需对现有系统进行改变,从而保证了系统的稳定性和安全性。Mutationdocker的使用为开发者提供了一个安全的测试平台,可以在不影响主系统的情况下进行变异测试。
#### 工具的五个阶段
网络物理突变工具按照以下五个阶段进行操作:
1. **安装工具**:用户需要下载并构建工具,具体操作步骤可能包括解压文件、安装依赖库等。
2. **生成突变体**:使用`./mutator`命令,顺序执行`./runconfiguration`(如果存在更改的config.txt文件)、`make`和工具执行。这个阶段涉及到对原始程序代码的变异生成。
3. **突变编译**:该步骤可能需要编译运行环境的配置,依赖于项目具体情况,可能需要执行`compilerun.bash`脚本。
4. **突变执行**:通过`runsave.bash`脚本执行变异后的代码。这个脚本的路径可能需要根据项目进行相应的调整。
5. **结果分析**:利用MATLAB脚本对变异过程中的结果进行分析,可能需要参考文档中的文件夹结构部分,以正确引用和处理数据。
#### 系统开源
标签“系统开源”表明该项目是一个开放源代码的系统,意味着它被设计为可供任何人自由使用、修改和分发。开源项目通常可以促进协作、透明性以及通过社区反馈来提高代码质量。
#### 文件名称列表
文件名称列表中提到的`mutationdocker-master`可能是指项目源代码的仓库名,表明这是一个主分支,用户可以从中获取最新的项目代码和文件。
### 详细知识点
1. **多点路径规划**是网络物理系统中的一项重要技术,它需要考虑多个节点或路径点在物理网络中的分布,以及如何高效地规划它们之间的路径,以满足例如时间、成本、距离等优化目标。
2. **突变测试**是软件测试的一种技术,通过改变程序中的一小部分来生成变异体,这些变异体用于测试软件的测试用例集是否能够检测到这些人为的错误。如果测试用例集能够正确地识别出大多数或全部的变异体,那么可以认为测试用例集是有效的。
3. **Mutator软件工具**的使用可以自动化变异测试的过程,包括变异体的生成、编译、执行和结果分析。使用此类工具可以显著提高测试效率,尤其是在大型项目中。
4. **Mutationdocker的使用**提供了一个简化的环境,允许开发者无需复杂的配置就可以进行变异测试。它可能包括了必要的依赖项和工具链,以便快速开始变异测试。
5. **软件的五个操作阶段**为用户提供了清晰的指导,从安装到结果分析,每个步骤都有详细的说明,这有助于减少用户在使用过程中的困惑,并确保操作的正确性。
6. **开源系统的特性**鼓励了代码共享、共同开发和创新,同时也意味着用户可以通过社区的力量不断改进软件工具,这也是开源项目可持续发展的核心。
通过以上描述和知识点的展开,我们可以了解到多点路径规划matlab代码-mutationdocker:变异码头工人是一个涵盖了网络物理系统、变异测试、自动化软件工具以及开源精神的综合性项目。它通过一系列操作流程为用户提供了一个高效和稳定的代码测试环境,并且以开源的形式促进了软件测试技术的共享和创新。