c++实现数制转换
时间: 2023-09-13 12:12:32 浏览: 80
数制转换是将一个数在不同进制下的表达方式进行转换的过程。以下是 c++ 实现十进制数转换为二进制、八进制和十六进制的代码示例:
```cpp
#include<iostream>
#include<stack>
using namespace std;
// 十进制数转二进制数
void decimalToBinary(int n) {
stack<int> s;
while(n) {
s.push(n % 2);
n /= 2;
}
while(!s.empty()) {
cout << s.top();
s.pop();
}
cout << endl;
}
// 十进制数转八进制数
void decimalToOctal(int n) {
stack<int> s;
while(n) {
s.push(n % 8);
n /= 8;
}
while(!s.empty()) {
cout << s.top();
s.pop();
}
cout << endl;
}
// 十进制数转十六进制数
void decimalToHexadecimal(int n) {
stack<int> s;
while(n) {
s.push(n % 16);
n /= 16;
}
while(!s.empty()) {
int x = s.top();
if(x < 10) cout << x;
else cout << (char)(x - 10 + 'A');
s.pop();
}
cout << endl;
}
int main() {
int n;
cin >> n;
decimalToBinary(n);
decimalToOctal(n);
decimalToHexadecimal(n);
return 0;
}
```
以上代码使用了栈来保存每一位的余数,然后再从栈中取出余数进行输出,最终得到了对应进制下的数。
相关推荐
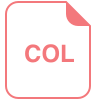
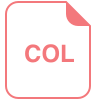
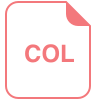
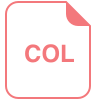
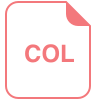









