用C语言编写程序使得在屏幕上绘制幂函数 f(x)=ex 的曲线与对数函数 f(x)=ln(x) 曲线 的迭加图形。其中幂函数用“*”表示,对数函数用“+” 表示。两种曲 线分别用两种颜色显示。
时间: 2023-11-28 08:50:17 浏览: 63
这个问题需要使用图形库来绘图,我可以提供一种使用 C 语言编写的绘制函数,但是需要使用 Windows 操作系统。我们可以使用 Windows 的 GDI+ 库来绘制图形。
首先,需要在代码中引入 GDI+ 库:
```c
#include <windows.h>
#include <gdiplus.h>
#pragma comment (lib, "Gdiplus.lib")
using namespace Gdiplus;
```
然后,定义绘制函数如下:
```c
void DrawGraph(HDC hdc, int width, int height) {
Graphics graphics(hdc);
// 绘制背景
SolidBrush brush(Color(255, 255, 255));
graphics.FillRectangle(&brush, 0, 0, width, height);
// 设置坐标系
Pen pen(Color(0, 0, 0));
graphics.DrawLine(&pen, 50, height - 50, width - 50, height - 50); // x轴
graphics.DrawLine(&pen, 50, height - 50, 50, 50); // y轴
// 绘制幂函数
Pen ePen(Color(255, 0, 0), 2.0f);
for (float x = -5.0f; x <= 5.0f; x += 0.01f) {
float y = expf(x);
graphics.DrawEllipse(&ePen, 50 + (x + 5.0f) / 10.0f * (width - 100), (height - 100) - y / 10.0f * (height - 100), 1, 1);
}
// 绘制对数函数
Pen lnPen(Color(0, 0, 255), 2.0f);
for (float x = 0.01f; x <= 10.0f; x += 0.01f) {
float y = logf(x);
graphics.DrawEllipse(&lnPen, 50 + x / 10.0f * (width - 100), (height - 100) - y / 2.0f * (height - 100), 1, 1);
}
}
```
最后,在主函数中调用该绘制函数即可:
```c
int main() {
HWND hWnd = GetConsoleWindow();
HDC hdc = GetDC(hWnd);
RECT rect;
GetClientRect(hWnd, &rect);
int width = rect.right - rect.left;
int height = rect.bottom - rect.top;
DrawGraph(hdc, width, height);
ReleaseDC(hWnd, hdc);
return 0;
}
```
这段代码将在 Windows 窗口中绘制出幂函数和对数函数的迭加图形。其中,幂函数用红色表示,对数函数用蓝色表示。
相关推荐
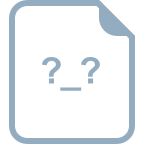
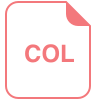
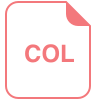
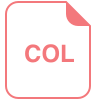
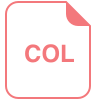
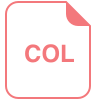









