TypeError: If no scoring is specified, the estimator passed should have a 'score' method. The estimator KNNImputer(n_neighbors=1) does not.
时间: 2024-05-07 17:21:21 浏览: 251
这个错误是因为你使用了一个没有 score 方法的 KNNImputer 估计器,并且在没有指定评分方法的情况下使用了它。为了解决这个问题,你可以尝试使用一个具有 score 方法的估计器,或者指定一个评分方法。
如果你想使用 KNNImputer 估计器,你可以使用 sklearn.metrics.make_scorer 方法来创建一个自定义的评分方法,并将其传递给 GridSearchCV 或 cross_val_score 方法。例如:
```python
from sklearn.impute import KNNImputer
from sklearn.metrics import make_scorer
from sklearn.model_selection import GridSearchCV
import numpy as np
# Create a custom scoring function
def my_scorer(y_true, y_pred):
return np.mean(np.abs(y_true - y_pred))
# Create a KNNImputer estimator
imputer = KNNImputer(n_neighbors=1)
# Create a parameter grid for GridSearchCV
param_grid = {'n_neighbors': [1, 3, 5]}
# Create a GridSearchCV object with the imputer and the parameter grid
grid_search = GridSearchCV(imputer, param_grid, scoring=make_scorer(my_scorer))
# Fit the GridSearchCV object to your data
grid_search.fit(X, y)
# Get the best estimator and its score
best_estimator = grid_search.best_estimator_
best_score = grid_search.best_score_
```
这个例子中,我们首先创建了一个自定义的评分函数 my_scorer,它计算真实值和预测值之间的平均绝对误差。然后,我们创建了一个 KNNImputer 估计器,并定义了一个参数网格 param_grid。接下来,我们创建了一个 GridSearchCV 对象,并将估计器、参数网格和自定义评分方法传递给它。最后,我们使用数据拟合 GridSearchCV 对象,并获取最佳估计器和它的分数。
如果你想使用一个具有 score 方法的估计器,你可以使用 sklearn.neighbors.KNeighborsRegressor 估计器来替换 KNNImputer。它实现了一个回归模型,并且具有 score 方法。例如:
```python
from sklearn.neighbors import KNeighborsRegressor
from sklearn.model_selection import GridSearchCV
# Create a KNeighborsRegressor estimator
knn = KNeighborsRegressor()
# Create a parameter grid for GridSearchCV
param_grid = {'n_neighbors': [1, 3, 5]}
# Create a GridSearchCV object with the knn estimator and the parameter grid
grid_search = GridSearchCV(knn, param_grid)
# Fit the GridSearchCV object to your data
grid_search.fit(X, y)
# Get the best estimator and its score
best_estimator = grid_search.best_estimator_
best_score = grid_search.best_score_
```
这个例子中,我们创建了一个 KNeighborsRegressor 估计器,并定义了一个参数网格 param_grid。然后,我们创建了一个 GridSearchCV 对象,并将估计器和参数网格传递给它。最后,我们使用数据拟合 GridSearchCV 对象,并获取最佳估计器和它的分数。由于 KNeighborsRegressor 估计器具有 score 方法,我们不需要定义一个自定义评分方法。
阅读全文
相关推荐
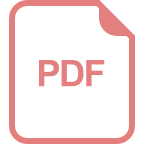
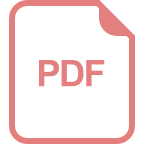








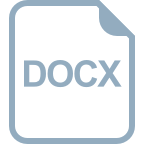