Hadoop编写矩阵乘法实例及演示
时间: 2024-05-16 16:13:52 浏览: 21
Hadoop编写矩阵乘法的实现可以分为以下几个步骤:
1. 将输入的矩阵划分为多个块,每个块的大小由用户指定。
2. 将矩阵块分发给不同的计算节点。
3. 在每个计算节点上,对两个矩阵块进行乘法计算,并将结果输出到本地文件系统。
4. 将所有计算节点的输出合并,得到最终的矩阵乘积结果。
下面是一个简单的矩阵乘法实现示例,假设输入的矩阵都是稠密矩阵:
1. 首先定义一个Mapper类实现矩阵乘法的第一步,即将矩阵块分发给不同的计算节点。Mapper的输入为矩阵A和B的块,输出为每个元素相乘的结果。
```java
public class MatrixMapper extends Mapper<LongWritable, Text, Text, Text> {
private int blockHeightA;
private int blockWidthB;
private int blockWidthA;
private int blockIndexA;
private int blockIndexB;
public void setup(Context context) {
Configuration conf = context.getConfiguration();
blockHeightA = conf.getInt("blockHeightA", 0);
blockWidthB = conf.getInt("blockWidthB", 0);
blockWidthA = conf.getInt("blockWidthA", 0);
blockIndexA = conf.getInt("blockIndexA", 0);
blockIndexB = conf.getInt("blockIndexB", 0);
}
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] input = value.toString().split(",");
int rowA = Integer.parseInt(input[0]);
int colA = Integer.parseInt(input[1]);
int valA = Integer.parseInt(input[2]);
int rowB = Integer.parseInt(input[3]);
int colB = Integer.parseInt(input[4]);
int valB = Integer.parseInt(input[5]);
if (colA == rowB) {
Text outputKey = new Text();
Text outputValue = new Text();
outputKey.set(rowA / blockHeightA + "," + colB / blockWidthB);
outputValue.set(blockIndexA + "," + rowA % blockHeightA + "," + colA + "," + valA + "," + blockIndexB + "," + colB % blockWidthB + "," + valB);
context.write(outputKey, outputValue);
}
}
}
```
2. 接下来定义一个Reducer类实现矩阵乘法的第二步,即对每个计算节点上的矩阵块进行乘法计算。Reducer的输入为每个块的索引和元素,输出为每个元素相乘的结果。
```java
public class MatrixReducer extends Reducer<Text, Text, Text, IntWritable> {
private int blockHeightA;
private int blockWidthB;
private int blockWidthA;
public void setup(Context context) {
Configuration conf = context.getConfiguration();
blockHeightA = conf.getInt("blockHeightA", 0);
blockWidthB = conf.getInt("blockWidthB", 0);
blockWidthA = conf.getInt("blockWidthA", 0);
}
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
List<Tuple> listA = new ArrayList<>();
List<Tuple> listB = new ArrayList<>();
for (Text value : values) {
String[] input = value.toString().split(",");
int blockIndexA = Integer.parseInt(input[0]);
int rowA = Integer.parseInt(input[1]);
int colA = Integer.parseInt(input[2]);
int valA = Integer.parseInt(input[3]);
int blockIndexB = Integer.parseInt(input[4]);
int colB = Integer.parseInt(input[5]);
int valB = Integer.parseInt(input[6]);
if (blockIndexA == 0) {
listA.add(new Tuple(rowA, colA, valA));
} else {
listB.add(new Tuple(rowA, colB, valB));
}
}
for (Tuple a : listA) {
for (Tuple b : listB) {
if (a.col == b.row) {
Text outputKey = new Text();
IntWritable outputValue = new IntWritable();
outputKey.set(a.row + "," + b.col);
outputValue.set(a.val * b.val);
context.write(outputKey, outputValue);
}
}
}
}
private static class Tuple {
int row;
int col;
int val;
public Tuple(int row, int col, int val) {
this.row = row;
this.col = col;
this.val = val;
}
}
}
```
3. 最后,在主函数中配置Job并提交作业。
```java
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "Matrix Multiplication");
job.setJarByClass(MatrixMultiplication.class);
Path inputPathA = new Path(args[0]);
Path inputPathB = new Path(args[1]);
Path outputPath = new Path(args[2]);
int blockHeightA = Integer.parseInt(args[3]);
int blockWidthA = Integer.parseInt(args[4]);
int blockWidthB = Integer.parseInt(args[5]);
job.getConfiguration().setInt("blockHeightA", blockHeightA);
job.getConfiguration().setInt("blockWidthA", blockWidthA);
job.getConfiguration().setInt("blockWidthB", blockWidthB);
job.setMapperClass(MatrixMapper.class);
job.setReducerClass(MatrixReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, inputPathA);
FileInputFormat.addInputPath(job, inputPathB);
FileOutputFormat.setOutputPath(job, outputPath);
job.waitForCompletion(true);
}
```
以上就是一个简单的Hadoop矩阵乘法实现示例。
相关推荐
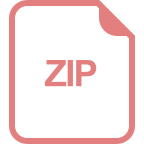
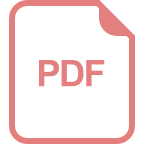














