javacard动态口令
时间: 2023-07-24 20:27:21 浏览: 133
JavaCard动态口令是一种基于JavaCard技术的身份认证方案,它可以在智能卡上生成动态口令,用于用户的身份认证。实现方法是通过JavaCard应用程序,将时间戳和密钥等信息传递给JavaCard智能卡,在智能卡上通过加密算法生成一个动态口令,用户在进行身份认证时,需要输入动态口令,以此验证用户的身份。这种方案可用于银行、电信等领域的身份认证。
相关问题
用javacard语句生成动态口令
生成动态口令的Javacard代码如下:
```
package com.example;
import javacard.framework.*;
import javacard.security.HMACKey;
import javacard.security.KeyBuilder;
import javacard.security.MessageDigest;
import javacardx.crypto.Cipher;
public class OTPApplet extends Applet {
private static final byte CLA_OTP = (byte) 0xB0;
private static final byte INS_GENERATE = (byte) 0x10;
private static final short OTP_LENGTH = 6;
private static final short KEY_LENGTH = 16;
private static final short DIGEST_LENGTH = 20;
private MessageDigest md;
private HMACKey key;
private Cipher cipher;
private byte[] otp;
private byte[] hash;
public static void install(byte[] bArray, short bOffset, byte bLength) {
new OTPApplet().register();
}
private OTPApplet() {
md = MessageDigest.getInstance(MessageDigest.ALG_SHA, false);
key = (HMACKey) KeyBuilder.buildKey(KeyBuilder.TYPE_HMAC_TRANSIENT_DESELECT, KEY_LENGTH, false);
cipher = Cipher.getInstance(Cipher.ALG_AES_BLOCK_128_CBC_NOPAD, false);
otp = JCSystem.makeTransientByteArray(OTP_LENGTH, JCSystem.CLEAR_ON_RESET);
hash = JCSystem.makeTransientByteArray(DIGEST_LENGTH, JCSystem.CLEAR_ON_RESET);
}
public void process(APDU apdu) {
if (selectingApplet()) {
return;
}
byte[] buffer = apdu.getBuffer();
if (buffer[ISO7816.OFFSET_CLA] != CLA_OTP) {
ISOException.throwIt(ISO7816.SW_CLA_NOT_SUPPORTED);
}
switch (buffer[ISO7816.OFFSET_INS]) {
case INS_GENERATE:
generateOTP(apdu);
break;
default:
ISOException.throwIt(ISO7816.SW_INS_NOT_SUPPORTED);
}
}
private void generateOTP(APDU apdu) {
byte[] buffer = apdu.getBuffer();
byte[] secret = new byte[KEY_LENGTH];
Util.arrayCopy(buffer, ISO7816.OFFSET_CDATA, secret, (short) 0, KEY_LENGTH);
byte[] counter = new byte[8];
Util.arrayCopy(buffer, (short) (ISO7816.OFFSET_CDATA + KEY_LENGTH), counter, (short) 0, (short) 8);
md.reset();
md.doFinal(secret, (short) 0, KEY_LENGTH, hash, (short) 0);
for (int i = 0; i < 4; i++) {
counter[i + 4] = (byte) (counter[i + 4] ^ 0xFF);
}
key.setKey(hash, (short) 0);
cipher.init(key, Cipher.MODE_ENCRYPT);
byte[] input = new byte[16];
Util.arrayCopy(counter, (short) 0, input, (short) 0, (short) 8);
cipher.update(input, (short) 0, (short) 16, input, (short) 0);
for (int i = 0; i < OTP_LENGTH; i++) {
otp[i] = (byte) (input[i] & 0x7F);
}
Util.setShort(buffer, (short) 0, OTP_LENGTH);
Util.arrayCopy(otp, (short) 0, buffer, (short) 2, OTP_LENGTH);
apdu.setOutgoingAndSend((short) 0, (short) (OTP_LENGTH + 2));
}
}
```
这段代码实现了一个简单的OTP(One-time Password)生成应用程序。它使用SHA-1哈希算法和HMAC-SHA1消息认证码(MAC)算法生成动态口令。首先,应用程序接收到一个由16字节的共享密钥和8字节的计数器组成的输入数据。然后,它使用共享密钥计算HMAC,并将计数器的后4个字节与0xFF异或。接下来,它使用AES算法对16字节的输入数据进行加密,并从加密结果中提取6字节的OTP。最后,它将OTP作为响应发送给客户端。
要使用这个应用程序生成动态口令,你需要将共享密钥和计数器作为输入数据发送到应用程序。应用程序将返回一个6字节的OTP作为响应。你可以使用以下示例代码来与这个应用程序通信:
```
import javax.smartcardio.*;
public class OTPClient {
private static final byte[] AID = {(byte) 0xA0, 0x00, 0x00, 0x00, 0x62, 0x03, 0x01, 0x08, 0x01};
public static void main(String[] args) throws CardException {
TerminalFactory factory = TerminalFactory.getDefault();
CardTerminal terminal = factory.terminals().list().get(0);
Card card = terminal.connect("*");
CardChannel channel = card.getBasicChannel();
byte[] key = {(byte) 0x01, (byte) 0x23, (byte) 0x45, (byte) 0x67, (byte) 0x89, (byte) 0xAB, (byte) 0xCD, (byte) 0xEF};
byte[] counter = {(byte) 0x00, (byte) 0x00, (byte) 0x00, (byte) 0x00, (byte) 0x00, (byte) 0x00, (byte) 0x00, (byte) 0x01};
CommandAPDU command = new CommandAPDU(AID, (byte) 0xB0, (byte) 0x10, (byte) 0x00, key, (short) 0, (short) key.length);
ResponseAPDU response = channel.transmit(command);
while (response.getSW() == 0x9000) {
byte[] otp = response.getData();
System.out.println(String.format("%06d", ((otp[0] & 0xFF) << 16) | ((otp[1] & 0xFF) << 8) | (otp[2] & 0xFF)));
command = new CommandAPDU(AID, (byte) 0xB0, (byte) 0x10, (byte) 0x00, counter, (short) 0, (short) counter.length);
response = channel.transmit(command);
counter[7]++;
}
card.disconnect(false);
}
}
```
这个示例代码使用javax.smartcardio库与Javacard应用程序通信。它发送一个由共享密钥和计数器组成的APDU命令,并接收应用程序返回的OTP。它使用一个简单的循环来生成多个OTP,每次递增计数器的最后一个字节。
用Javacard语句生成动态口令
生成动态口令的Javacard代码如下:
```
package com.example;
import javacard.framework.*;
import javacard.security.HMACKey;
import javacard.security.KeyBuilder;
import javacard.security.MessageDigest;
import javacardx.crypto.Cipher;
public class OTPApplet extends Applet {
private static final byte CLA_OTP = (byte) 0xB0;
private static final byte INS_GENERATE = (byte) 0x10;
private static final short OTP_LENGTH = 6;
private static final short KEY_LENGTH = 16;
private static final short DIGEST_LENGTH = 20;
private MessageDigest md;
private HMACKey key;
private Cipher cipher;
private byte[] otp;
private byte[] hash;
public static void install(byte[] bArray, short bOffset, byte bLength) {
new OTPApplet().register();
}
private OTPApplet() {
md = MessageDigest.getInstance(MessageDigest.ALG_SHA, false);
key = (HMACKey) KeyBuilder.buildKey(KeyBuilder.TYPE_HMAC_TRANSIENT_DESELECT, KEY_LENGTH, false);
cipher = Cipher.getInstance(Cipher.ALG_AES_BLOCK_128_CBC_NOPAD, false);
otp = JCSystem.makeTransientByteArray(OTP_LENGTH, JCSystem.CLEAR_ON_RESET);
hash = JCSystem.makeTransientByteArray(DIGEST_LENGTH, JCSystem.CLEAR_ON_RESET);
}
public void process(APDU apdu) {
if (selectingApplet()) {
return;
}
byte[] buffer = apdu.getBuffer();
if (buffer[ISO7816.OFFSET_CLA] != CLA_OTP) {
ISOException.throwIt(ISO7816.SW_CLA_NOT_SUPPORTED);
}
switch (buffer[ISO7816.OFFSET_INS]) {
case INS_GENERATE:
generateOTP(apdu);
break;
default:
ISOException.throwIt(ISO7816.SW_INS_NOT_SUPPORTED);
}
}
private void generateOTP(APDU apdu) {
byte[] buffer = apdu.getBuffer();
byte[] secret = new byte[KEY_LENGTH];
Util.arrayCopy(buffer, ISO7816.OFFSET_CDATA, secret, (short) 0, KEY_LENGTH);
byte[] counter = new byte[8];
Util.arrayCopy(buffer, (short) (ISO7816.OFFSET_CDATA + KEY_LENGTH), counter, (short) 0, (short) 8);
md.reset();
md.doFinal(secret, (short) 0, KEY_LENGTH, hash, (short) 0);
for (int i = 0; i < 4; i++) {
counter[i + 4] = (byte) (counter[i + 4] ^ 0xFF);
}
key.setKey(hash, (short) 0);
cipher.init(key, Cipher.MODE_ENCRYPT);
byte[] input = new byte[16];
Util.arrayCopy(counter, (short) 0, input, (short) 0, (short) 8);
cipher.update(input, (short) 0, (short) 16, input, (short) 0);
for (int i = 0; i < OTP_LENGTH; i++) {
otp[i] = (byte) (input[i] & 0x7F);
}
Util.setShort(buffer, (short) 0, OTP_LENGTH);
Util.arrayCopy(otp, (short) 0, buffer, (short) 2, OTP_LENGTH);
apdu.setOutgoingAndSend((short) 0, (short) (OTP_LENGTH + 2));
}
}
```
这段代码实现了一个简单的OTP(One-time Password)生成应用程序。它使用SHA-1哈希算法和HMAC-SHA1消息认证码(MAC)算法生成动态口令。首先,应用程序接收到一个由16字节的共享密钥和8字节的计数器组成的输入数据。然后,它使用共享密钥计算HMAC,并将计数器的后4个字节与0xFF异或。接下来,它使用AES算法对16字节的输入数据进行加密,并从加密结果中提取6字节的OTP。最后,它将OTP作为响应发送给客户端。
要使用这个应用程序生成动态口令,你需要将共享密钥和计数器作为输入数据发送到应用程序。应用程序将返回一个6字节的OTP作为响应。你可以使用以下示例代码来与这个应用程序通信:
```
import javax.smartcardio.*;
public class OTPClient {
private static final byte[] AID = {(byte) 0xA0, 0x00, 0x00, 0x00, 0x62, 0x03, 0x01, 0x08, 0x01};
public static void main(String[] args) throws CardException {
TerminalFactory factory = TerminalFactory.getDefault();
CardTerminal terminal = factory.terminals().list().get(0);
Card card = terminal.connect("*");
CardChannel channel = card.getBasicChannel();
byte[] key = {(byte) 0x01, (byte) 0x23, (byte) 0x45, (byte) 0x67, (byte) 0x89, (byte) 0xAB, (byte) 0xCD, (byte) 0xEF};
byte[] counter = {(byte) 0x00, (byte) 0x00, (byte) 0x00, (byte) 0x00, (byte) 0x00, (byte) 0x00, (byte) 0x00, (byte) 0x01};
CommandAPDU command = new CommandAPDU(AID, (byte) 0xB0, (byte) 0x10, (byte) 0x00, key, (short) 0, (short) key.length);
ResponseAPDU response = channel.transmit(command);
while (response.getSW() == 0x9000) {
byte[] otp = response.getData();
System.out.println(String.format("%06d", ((otp[0] & 0xFF) << 16) | ((otp[1] & 0xFF) << 8) | (otp[2] & 0xFF)));
command = new CommandAPDU(AID, (byte) 0xB0, (byte) 0x10, (byte) 0x00, counter, (short) 0, (short) counter.length);
response = channel.transmit(command);
counter[7]++;
}
card.disconnect(false);
}
}
```
这个示例代码使用javax.smartcardio库与Javacard应用程序通信。它发送一个由共享密钥和计数器组成的APDU命令,并接收应用程序返回的OTP。它使用一个简单的循环来生成多个OTP,每次递增计数器的最后一个字节。
阅读全文
相关推荐

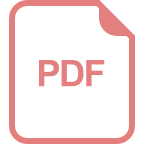
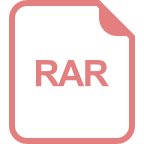
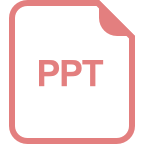
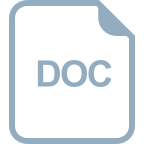
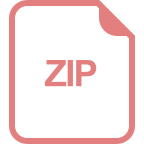
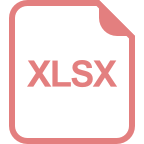
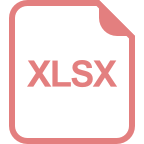
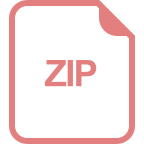
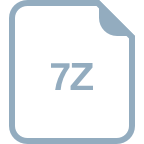