python导入12导联的ECG文本文件,并1.画出各导联的波形图、数据值分布柱状图 2.采用函数/模块定义,取出各导联中数据最大值、最小值、平均值、数据总点数 3.取出特定跨度内的ECG数据片段并画图显示
时间: 2024-03-14 19:45:04 浏览: 131
好的,针对您的问题,您可以使用Python内置的`csv`模块来读取ECG文本文件。以下是示例代码,以帮助您开始处理ECG数据。
1. 画出各导联的波形图、数据值分布柱状图
```python
import csv
import numpy as np
import matplotlib.pyplot as plt
# 导入12导联ECG数据
with open('文件路径', 'r') as f:
reader = csv.reader(f, delimiter='\t')
data = list(reader)
data = np.array(data, dtype=float)
# 绘制各导联波形图
plt.figure(figsize=(10, 10))
for i in range(12):
plt.subplot(6, 2, i+1)
plt.plot(data[:,i])
plt.title('Lead %d' % (i+1))
plt.xlabel('Sample index')
plt.ylabel('Amplitude (mV)')
plt.tight_layout()
plt.show()
# 绘制各导联数据值分布柱状图
plt.figure(figsize=(10, 10))
for i in range(12):
plt.subplot(6, 2, i+1)
plt.hist(data[:,i])
plt.title('Lead %d' % (i+1))
plt.xlabel('Amplitude (mV)')
plt.ylabel('Number of samples')
plt.tight_layout()
plt.show()
```
2. 采用函数/模块定义,取出各导联中数据最大值、最小值、平均值、数据总点数
```python
import csv
import numpy as np
def get_signal_stats(file_path):
with open(file_path, 'r') as f:
reader = csv.reader(f, delimiter='\t')
data = list(reader)
data = np.array(data, dtype=float)
stats = {}
for i in range(12):
stats['Lead %d' % (i+1)] = {}
stats['Lead %d' % (i+1)]['max'] = np.max(data[:,i])
stats['Lead %d' % (i+1)]['min'] = np.min(data[:,i])
stats['Lead %d' % (i+1)]['mean'] = np.mean(data[:,i])
stats['Lead %d' % (i+1)]['num_points'] = data.shape[0]
return stats
record_stats = get_signal_stats('文件路径')
print(record_stats)
```
3. 取出特定跨度内的ECG数据片段并画图显示
```python
import csv
import numpy as np
import matplotlib.pyplot as plt
# 导入12导联ECG数据
with open('文件路径', 'r') as f:
reader = csv.reader(f, delimiter='\t')
data = list(reader)
data = np.array(data, dtype=float)
# 取出特定跨度内的ECG数据片段并画图显示
start_idx = 1000
end_idx = 2000
plt.figure(figsize=(10, 10))
for i in range(12):
plt.subplot(6, 2, i+1)
plt.plot(data[start_idx:end_idx, i])
plt.title('Lead %d' % (i+1))
plt.xlabel('Sample index')
plt.ylabel('Amplitude (mV)')
plt.tight_layout()
plt.show()
```
希望这些示例代码能够帮助您开始处理ECG数据。如果您有任何问题或需要进一步帮助,请告诉我。
阅读全文
相关推荐
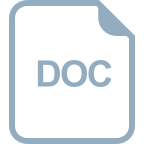
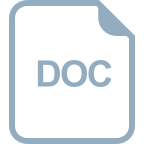
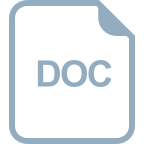




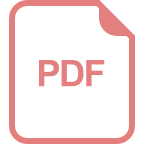
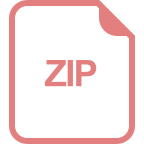
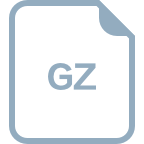
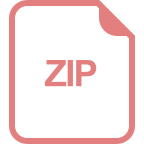
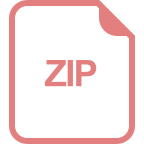
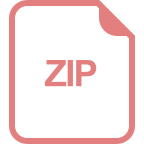
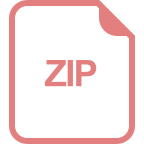
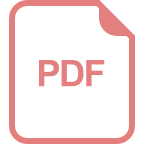
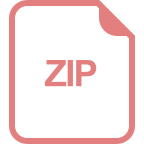
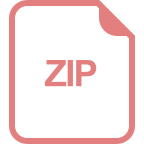
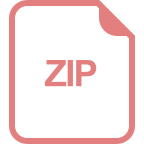